Language refers to a developer's programming language for writing codes. It is a formalised set of syntax, rules and instructions. Moreover, it lets you converse with computers and direct them to perform specific tasks. Some of the most common languages used in programming are JavaScript, Python and C++. The different programming languages have their own syntax and semantics. You will choose a language based on the task requirements and familiarity with the language.
To use this language in programming, you must apply its syntax and layout. Language syntax refers to rules and conventions that define the format of expressions in a programming language. It specifies how you write, organise and combine the language elements to create valid and meaningful code. Syntax ensures your code is valid and well-structured. It also avoids syntax errors and ensures the code writes the intended logic accurately. You will learn more about the syntax in this topic.
On the other hand, layout refers to the arrangement of code elements within a programming language. It involves using indentation, line breaks, whitespace and other formatting conventions. The layout makes the code easier to understand, navigate and change. Likewise, it reduces the likelihood of errors
As you learnt, language syntax is rules and conventions that define the format of expressions in a programming language. They ensure you write the codes logically and accurately to avoid errors. There are various basic syntax rules that you should know. These syntax rules can vary between programming languages. However, here are the common basic syntax:
Identifiers
Identifiers are names that identify variables, functions, classes or other entities. They provide a way to refer to specific elements defined by the language's syntax rules. Moreover, they follow certain conventions, such as:
- Starting with a letter or underscore
- Consisting of letters, digits or underscores
The rules for creating identifiers may vary depending on the programming language. However, here are the common basic syntax rules that apply to most languages:
Identifiers typically consist of letters (uppercase and lowercase), digits and underscores (_). However, some languages may have extra rules, such as allowing certain special characters. Likewise, they can disallow digits as the first character.
There may be restrictions on the minimum and maximum length of identifiers. For example, a language may require identifiers to be at least one character long. Likewise, they have a maximum length of approximately 255 characters.
Programming languages often have naming conventions for identifiers to enhance code readability. These conventions may specify using camel case, snake case or other naming styles. Moreover, certain words or phrases may be reserved or discouraged for using identifiers. These can prevent conflicts or confusion with keywords or builtin functions.
Some languages distinguish between lowercase and uppercase characters, making identifiers case-sensitive. This means that 'myVariable' and 'MyVariable' may be treated as distinct identifiers in such languages.
Languages have reserved keywords that cannot be used as identifiers. These have predefined meanings. Using reserved keywords as identifiers can lead to syntax errors. You must familiarise yourself with the reserved keywords in the language you will use.
Keywords
Keywords are reserved words in a language with special meanings and purposes. They are predefined, and you cannot use them as identifiers. Likewise, they define control structures, data types or other language-specific functionalities. Here are some examples of keywords:
Keywords | Meaning |
---|---|
if | Used for conditional statements, allowing the program to decide based on certain conditions |
for | Used to create a loop that repeats over a sequence of values |
while | Used to create a loop that continues executing a block of codes – This is as long as a certain condition is true. |
class | Used to define a new user-defined data type – Class is a blueprint for creating objects. |
def | Used to define a function in a program – Functions are blocks of code that do a specific task or operation. |
The basic syntax rules for keywords in coding are as follows:
- Case sensitivity: Keywords are typically case-sensitive. It means they must be written exactly as specified by the programming language. For example, 'if' and 'IF' may be treated as different keywords.
- Reserved usage: Keywords cannot be used as identifiers within the code. Attempting to use a keyword as an identifier will result in a syntax error.
- Spelling-specific and language-specific: The specific set of keywords depends on the programming language. Different languages have sets of reserved words that serve specific purposes in their syntax.
Literals
Literals are fixed values that appear directly in the code and represent specific data types. They assign values to variables or represent constant values. Here are the basic language syntax rules you can follow:
Literals | Rules | Examples |
---|---|---|
Numeric | This represents numerical values. You can use it in mathematical equations and storing numerical data. |
|
String | This represents sequences of characters enclosed in quotation marks (“…”). You can use it to represent text and alphanumeric data. |
|
Boolean | This represents the two truth values, either true or false. You can use it in conditional statements and logical expressions. |
|
Character | This represents individual characters enclosed in single quotes (‘…’). You can use it to represent letters, digits and special symbols. |
|
Literals provide explicit data representations within the code.
Operators
Operators are symbols or keywords that perform operations on one or more operands to produce results. They can control, calculate or compare values. You will learn more about these in the next subtopic.
Here are the basic language syntax rules you can follow:
Operators | Rules | Examples |
---|---|---|
Arithmetic | This is used to perform basic mathematical operations. |
|
Assignment | This is used to assign a value to a variable. The value on the right side of the operator is assigned to the variable on the left side. | Assignment (= |
Comparison | This is used to compare values and return a Boolean (true or false) result. |
|
Logical | This is used to perform logical operations and return Boolean results. |
|
Bitwise | This is used to perform operations on the binary representation of integer values. |
|
Conditional (Ternary) | This provides a concise way of writing an if-else statement. | Result = expression_if_true if condition else expression_if_false |
Separators
Separators are characters that separate or delimit different parts of code or values. They help structure and organise the code. Here are the basic language syntax rules you can follow:
Separator | Symbol | Rules |
---|---|---|
Comma | , |
|
Semicolon | ; |
|
Parenthesis | () |
|
Brace | {} |
|
Square bracket | [] |
|
Colon | : |
|
Full stop or dot | . |
|
Arrow | -> |
|
Vertical bar | | |
|
Underscore | _ |
|
Expressions
Expressions are combinations of literals, identifiers, operators and function calls. They represent computations or calculations within a program.
Expressions can include the following:
Expression | Description |
---|---|
Arithmetic expression | An arithmetic expression involves mathematical operations like addition, subtraction, multiplication and division. |
Comparison expression | A comparison expression compares two values and produces a Boolean result (true or false) based on the comparison. |
Logical expression | A logical expression involves logical operations like ‘AND’, ‘OR’ and ‘NOT’. |
Function call expressions | A function call expression calls a function with arguments and returns a value based on the function's implementation. |
Variable expression | A variable expression involves the use of variables to represent values. |
String concatenation expression | In languages that support strings, a string concatenation expression combines strings using the concatenation operator (+). |
Expressions with parentheses | Parentheses are used to control the order of operations in complex expressions. |
Here are the basic language syntax rules for expressions in coding:
The arrangement of operands and operators determines the value the expression evaluates to.
Parentheses group parts of an expression and control the order of operations. Expressions inside parentheses are evaluated first, following the standard rules of precedence.
Expressions can involve function calls, where a function is called with arguments to produce a value. The return value of the function becomes part of the expression.
Variables are used to store values that are part of expressions. The values of variables can be combined with literals and operators to create expressions.
Expressions must conform to data type rules. Sometimes, typecasting is required to ensure correct evaluations. The result of an expression is often of the same data type as the operands involved, but some operators may produce different data types.
In languages that support strings, expressions can involve string concatenation using operators like (+).
Statements
Statements are executable units of code that perform specific actions or operations. They represent instructions to be carried out by the program. Moreover, these can include the following:
- Variable assignments
- Function calls
- Conditional statements (if-else)
- Loops (for and while)
Each statement typically ends with a delimiter, such as a semicolon (;) or a newline character. Here is an example in Python:
In this statement, the 'print()' function displays the text 'Hello, World!' on the console or output screen. The string "Hello, World!" is enclosed in double quotes to represent a string literal. When this statement is executed, it will output the text "Hello, World!" to the standard output.
Here are the basic language syntax rules for statements in coding:
Termination
Statements are typically terminated with a specific character, such as a semicolon (;). The semicolon serves as a separator between statements, indicating the end of one statement and the beginning of the next. Not all languages need semicolons as statement terminators, but they are commonly used in C++ and JavaScript. Here is an example from Python:
This code terminates the program's execution. It is commonly used to exit the program when a specific condition is met, or an error occurs.
Conditional statements
Conditional statements let the program make decisions based on certain conditions. The most common type of conditional statement is the 'if' statement, which executes a block of code if a condition is true. Some languages also provide variations like 'ifelse', 'if-else if' and 'switch' statements. These apply to more complex branching
Here is an example in C++:
The code takes user input and checks if the number is greater than 0. If it is, it prints 'Positive'; otherwise, it prints 'Negative or Zero'.
Loops (iteration statements)
Loops execute a block of code repeatedly as long as a condition is true or for a specified number of times. They help reduce redundant code and allow efficient processing of repetitive tasks. Here is an example in Python:
This code shows a for loop that iterates from 0 to 4, printing the values at each iteration.
Function calls
Function calls are statements that invoke functions or methods, passing any required arguments. They encapsulate reusable blocks of code, and their execution triggers function calls.
Here is an example in JavaScript:
This code defines a function 'greet' that takes a 'name' parameter and prints a greeting. It then calls the function with the argument 'Alice'.
Declaration and assignment
Statements declare variables and assign values to them. The data type of the variable is often explicitly stated during the declaration. Here is an example in C++:
This code declares an integer variable 'age' and assigns the value '25' to it.
Control flow statements
Control flow statements, such as break, continue and return, are used to control the flow of a program. They allow you to exit loops prematurely, skip iterations or return values from functions. Here is an example in JavaScript:
Important
The basic language syntax rules can vary between programming languages. While there are similarities, each language has its rules and components. Syntax components may have different forms, usage or naming conventions in various languages.Steps to apply basic syntax rules
To apply basic syntax rules, you can follow these general steps:
- Familiarise yourself with the syntax rules and conventions of the programming language you are using. Syntax rules specify how you should structure statements and expressions. These include the proper use of keywords, operators and punctuation.
- Identify the syntaxes in your code. Each programming language may have its specific syntax components.
- Follow naming conventions for identifiers. It includes using meaningful names that reflect the purpose or role of variables, functions or classes. Likewise, ensure that identifiers are valid and follow language-specific rules.
- Follow the recommended formatting guidelines for indentation, spacing and line length. Consistent formatting enhances code readability and helps convey the program's structure.
- Use the appropriate operators to perform desired computations or comparisons. Construct expressions using the syntaxes to create meaningful computations.
- Assemble statements based on the syntax rules of the programming language. Syntax rules are instructions that perform specific actions or tasks.
Following these basic syntax rules help prevent syntax errors. Syntax errors refer to mistakes in the code that violate the syntax rules of the language. It means the code does not follow the correct grammar and form the language expects. Syntax errors prevent the compiling or execution of the code successfully.
When syntax errors occur, they can have various negative consequences. They can cause the application to crash, behave unpredictably and produce incorrect outputs. Moreover, it will confuse developers and need more troubleshooting and debugging time.
Quiz
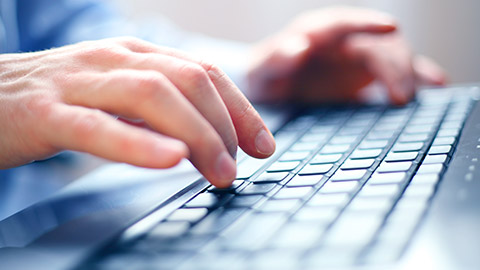
After you learnt how to apply the basic syntax rules, you can use these to create a code. Code refers to instructions written in a programming language the computer can execute. It represents the algorithms, logic and commands to tell the computer the tasks and how to perform them.
These codes are written using a specific language, such as Python, JavaScript or C++. Each language has syntax and rules that define how you will structure and write these codes.
In creating codes, you can use the following:
Data types
Data types are categories that define the data the program can store and manipulate. Each language provides a set of predefined data types. These determine the values you can assign to variables or use in expressions. Here are some common data types found in languages:
Data Type | Meaning | Examples |
---|---|---|
Integer | Integer represents whole numbers without a fractional part. It can be a positive or negative number. Moreover, you can use it for counting or representing discrete quantities. |
|
Float or double | A floating-point or double data represents real numbers with a fractional part. You can use them in calculations requiring decimal precisions. |
|
String | String represents a sequence of characters. You can use it to store text, such as names, sentences or textual data. |
|
Boolean | Boolean represents a logical value that can either be true or false. You can use it for deciding or controlling flow in programming. |
|
Character | A character represents a single character, such as letters, digits or symbols. You can use it for text manipulation or encoding. |
|
Array | An array is a collection of elements of the same data type stored in a contiguous memory block. You can store many values under a single variable name, accessed using an index. |
|
List | A list represents an ordered collection of elements from different data types. It provides flexibility in storing and manipulating data with variable lengths and types. | {1, “apple”, True 3.14} |
Object or class | An object or class represents a user-defined data type containing data and behaviour. You can use it to model realworld entities with their attributed and methods. | To define a person, you can use the code below: |
Null or undefined | A null or undefined represents the absence of a value. It is commonly used to indicate the absence of a meaningful value. | “null” |
Dictionary or map | A dictionary or map represents a collection of key-value pairs. Each value is associated with a unique key. This allows efficient retrieval and storage of data based on specific keys. | {“name” : “John”, “age” : 25, “city” : “New York”} |
Operators
Operators are symbols or keywords that perform operations on one or more operands to produce results. They can control, calculate or compare values. Here are the common operators and their roles in creating codes:
Type of Operators | Meaning | Examples |
---|---|---|
Arithmetic | This type can calculate numeric operands. It follows standard mathematical rules. Likewise, it helps in calculations, data manipulation and numeric analysis within programs. |
|
Assignment | This can assign values to a variable. The basic assignment operator assigns its right-hand side’s value to the variable on its left-hand side. This forms the foundation of updating variable values in a program. | You can use the equal sign (=) |
Bitwise | This can perform operations on binary representation values. It manipulates individual bits of a value. |
|
Comparison | This can compare two values and return a Boolean value (true or false). |
|
Logical | This can perform logical operations on Boolean values. This is employed to make decisions based on multiple conditions or to combine conditions. |
|
Concatenation | This is used to manipulate and concatenate strings. It allows you to build dynamic output, combine user inputs, or create complex messages. |
|
Expressions
Expressions are combinations of literals, identifiers, operators and function calls. They represent computations or calculations within a program. Likewise, they can involve arithmetic operations, logical evaluations or other data transformations.
Expressions use variables to store and represent data. Likewise, they use operators to perform specific operations on the variables or values. They also use values to provide input or constants for the operations. When evaluating an expression, the variables' current values replace the old ones. They also apply the operators to those values to compute a final result.
Here is how the components work together in expressions:
- Variables represent placeholders that hold data or values. You can assign values and use them in expressions. For example, x can be a variable representing a number.
- Operators perform specific operations on variables or values to produce a result. For example, you can add variables using operators.
- Values provide input or constants for the operations. They can be numeric values, strings, Boolean values or other data types. For example, five can be a value representing a numeric constant.
Here are examples of expressions:
Expression | Example | Meaning |
---|---|---|
Arithmetic | X + 5 | It adds the values of the variable ‘x’ to the value ‘5’, resulting in a computed value. |
String concatenation | ‘Hello’ + name | It combines the string ‘Hello’ with the value stored in the variable ‘name’, creating a new string. |
Comparison expression | ‘age >= 18’ | It compares the value stored in the variable ‘age’ with the constant value ‘18’ using the greater-than-or-equal-to operator. It can have a Boolean result (true or false). |
Logical expression | ‘(x > 0) and (y < 10)’ | It combines two comparison expressions using the logical and operator. Thus, it evaluates to ‘True’ if both conditions are satisfied. |
Important
The basic concepts of data types, operators and expressions are similar across common languages. However, you must consult the language-specific documentation and resources. These can provide a more comprehensive understanding of each language's specific syntax and usage.Data types, operators and expressions form the foundation for computations, data manipulation and logic in programming. Understanding these can help you write code that accurately represents desired computations. Likewise, they enhance code readability and execution. They also create robust and functional applications.
You can follow similar steps to create codes using various languages' data types, operators and expressions. Here are the general steps you can follow:
- Familiarise yourself with the syntax, rules and conventions of the language you are using. You have learnt these in the previous subtopics. Likewise, each language has resources that can help you learn the basics.
- Choose appropriate variable names and declare them with the desired data types. Variables will hold values that you will manipulate using operators and expressions.
- Assign values to variables based on the data types you have chosen. You should ensure the assigned values are compatible with the data types.
- Use operators to perform operations on the variables and their values. You should choose the appropriate operators based on the desired computations.
- Combine variables, operators and values to form expressions that represent computations. Expressions can involve arithmetic operations, logical operations, comparisons and more.
- Execute the code in your chosen programming compiler. You should test the output and verify that it matches your expectations. Debug any errors or issues that arise.
- Review your code for readability, efficiency and best practices. You should look for chances to optimise the code for better performance or clarity.
- Practise creating codes using data types, operators and expressions in various scenarios. As you gain experience, you will become more proficient in using these elements effectively.
Watch
Access watch the links below to learn more about various languages' data types, operators and expressions:
Reading
Access and read the link below to find out more about Visual Studio Code in Action
The previous subtopic taught you about data types, operators, syntax and expressions. Thus, you can use this knowledge to apply variables and variable scope. Understanding variables is crucial for writing effective and flexible code. Assigning values to variables helps you work with various data types, such as numbers, strings, Booleans and more.
Variables are named storage locations used to hold values in computer programs. They allow you to store and manipulate data during program execution. Moreover, you can assign a specific data type to each variable, determining the values you can store.
You can use variables for various purposes in programming:
- Data storage: Variables allow keeping and storing values for later use in the program.
- Data manipulation: Assigning values to variables allows you to perform data calculations. You can also transform or modify these stored data.
- Memory management: Variables handle the allocation and deallocation of memory resources. This depends on the need during program execution.
- Information representation: Variables provide a way to represent and work with different data types, such as numbers, text and Boolean values.
- Program control: Variables can store and check conditions, make decisions and control the flow of program execution.
Here are examples of variables:
Python
In this snippet, the variable ‘x’ is assigned the value ‘10’.
JavaScript
In this example, the variable ‘name’ is assigned the string value ‘John’
C++
In this example, the variable ‘count’ is assigned the integer value ‘5’.
Variables provide a way to store and manipulate data during program execution. Thus, it allows for the representation and manipulation of various information. Likewise, they can help you create robust and adaptable software applications.
Here are the different types of variables commonly used in programming:
These are variables whose values cannot be changed once they are assigned. They are usually declared using the ‘const’ keyword. Likewise, these represent fixed values that remain constant throughout the program.
These are declared variables outside of any function or class, making them accessible from anywhere in the program. They have a global scope and can be accessed and modified by any part of the program.
These are also known as static variables. They are associated with a class rather than an instance of the class. Moreover, they are shared among all instances of the class. You can also access them using the class name. You can declare class variables using the 'static' keyword.
These variables are specific to an instance or object of a class. Each instance of the class has its copy of instance variables. Moreover, they are declared within a class but outside any methods or functions.
These variables are declared and used within a specific block, such as a function or a loop. They have a limited scope and are only accessible within the block where they are declared.
You also must know the variable scope. Variable scope refers to the visibility or accessibility of variables within different parts of a program. It determines where a variable you can access and use is, as well as the duration or lifetime of the variable. The scope of a variable is typically determined by its declaration and the block of code.
Examples of variable scope
Global scope
Variables stated outside of any function or block have a global scope. You can access them anywhere within the program, including inside functions or blocks. Here is a snippet to show you:
In the code snippet, a variable named 'global_var' is declared and assigned a value of 10 in the global scope. It means that the variable is accessible from anywhere in the program.
Following that, the function named 'my_function' is defined. Inside the function, the statement 'print(global_var)' is used to print the value of 'global_var'. Since 'global_var' is declared in the global scope, it is accessible within the function. Thus, when 'my_function' is called, it will print the value of 'global_var'.
After the function definition, another statement, 'print(global_var)', is used outside the function. It will also print the value of 'global_var'. As ‘global_var’ is declared in the global scope, it is also accessible outside the function. Thus, when this statement is used, it will print the value of 'global_var' again.
Local scope
Variables declared within a function or block have a local scope. You can only access them from within that specific function or block. Here is a snippet to show you:
In the code snippet, a function named 'my_function' is defined. Inside the function, a variable named 'local_var' is declared and assigned a value of 5. This variable is local because it is defined within the function's scope.
The 'print(local_var)'statement inside the function successfully prints the value of 'local_var'. This is because it is within the scope of the variable.
When the 'my_function()' is called, the code inside the function is executed, including the 'print(local_var)' statement. As a result, it will print the value of 'local_var', which is 5.
However, when the statement 'print(local_var)' is encountered outside the function, it raises an error. This is because 'local_var' is a local variable only accessible within the function's scope. It cannot be accessed outside the function. Thus, attempting to print 'local_var' outside the function results in an error.
The main difference
The main difference between global and local scope is the accessibility of variables. You can access and use global functions from anywhere within the program. On the other hand, local variables are limited to the specific function or block in which they are declared.
Moreover, global variables have a lifetime that spans the entire program duration. They initialise once and access them until the program terminates. Local variables have a shorter lifetime. Each time the associated block or function is executed, they are created and destroyed.
As mentioned, you will store the data types in the variables. In most programming languages, you can store data types into variables using the assignment operator (=). Here are the general steps you can follow:
- Declare a variable by providing a name for it.
- Choose an appropriate data type for the variable based on the value you want to store.
- Assign a value of the corresponding data type to the variable using the assignment operator (=).
Here is an example in Python:
In this example, 'age' is a variable of integer data type storing the value '25'. Likewise, 'name' is a variable of string data type storing the value 'John Doe' and 'is_active' is a variable of Boolean data type storing the value 'True'.
The specific syntax and rules for declaring and initialising variables may vary. It depends on the language you are using, but the concept of storing data types into variables remains the same.
After storing data types into variables, you can apply these variables and variable scope in creating an application. Here are the general steps you can follow:
Identify the purpose and data requirements for your variable. You should choose an appropriate data type that matches the kind of data you want to store in the variable.
Declare the variable by specifying its name and data types. The declaration allocates memory for the variable and associates a name with it.
Assign an initial value to the variable using the assignment operator (=). This step is optional, depending on the requirements. If you do not assign a value, the variable may contain garbage data.
Determine the appropriate scope for the variable based on your program's design and requirements. You should choose from options such as global scope, class scope, instance scope or local scope. Define the variables within the appropriate scope to control their visibility and accessibility.
Access and manipulate the variable as needed in your code. You should read its value, modify it and use it in calculations or operations.
Follow good programming practices when working with variables. You should avoid naming conflicts by using meaningful and descriptive variable names. Ensure you initialise variables before using them. Also, respect variable scopes and avoid using variables outside their defined scope.
Common mistakes
When applying variables and their scope, it is unavoidable to commit mistakes. You must be mindful of these common mistakes:
- Shadowing variables. Shadowing occurs when a variable within a nested scope has a similar name as a variable in an outer scope. It can lead to confusion and bugs. To avoid this, choose distinct names for variables in different scopes.
- Overusing global variables. Excessive use of global variables can make your code harder to understand and maintain. You must limit the use of global variables. Instead, you can use passing variables as parameters or function return values.
- Not checking for variable existence or null values. Always review variables for existence and check for null or undefined values before using them. It helps prevent runtime errors and enhances the code's reliability.
- Declaring unused variables. Avoid declaring variables that are never used. They clutter your code and can mislead other developers. Remove any unused variables to keep your code clean and improve performance.
Comparison of how variables and variable scope are used and defined
Variables and variable scope are basic concepts in languages such as Python, C++ and JavaScript. While their usage and definition may have some similarities, there are also notable differences. Here is a comparison of how variables and variable scope are used and defined in these languages:
Programming Language | Variable Declaration | Variable Scope |
---|---|---|
JavaScript | JavaScript allows variables to be declared using the keywords 'var', 'let' or 'const'. The 'var' keyword has function scope, while 'let' and 'const' have block scope. | In JavaScript, variables declared with the 'var' keyword have function scope. They are accessible within the entire function in which they are defined. On the other hand, variables declared with 'let' and 'const' have block scope. They are limited to the block (enclosed by curly braces) in which they are defined. Block-scoped variables are not accessible outside of the block in which they are declared. |
Python | Python uses dynamic typing, meaning variables do not need an explicit data type declaration. You can assign a value to a variable, and Python will infer its data type. | In Python, variables can have local and global scopes. When variables are defined within a function, they have local scope. This means they are accessible only within that specific function. These variables are not accessible outside of the function. On the other hand, variables declared outside of any function at the module level have global scope. They are accessible from anywhere within the module or program. |
C++ | C++ requires explicitly declaring variables with their data type before they can be used. Variables can be declared within a block of code at any point. | C++ has block scope, where variables defined within a block are only accessible within that block. It also allows for global variables defined outside of any function, which can be accessed anywhere in the program. |
You should note these languages have different syntax and scoping rules. However, the underlying concept of variables remains the same. Variables serve as placeholders to store and manipulate data. Moreover, their scope defines their visibility and accessibility within the program.
You should also note that there are more programming languages that you can use. These can vary depending on your organisation.
You can also use library functions when writing codes for creating an application. You can access this library of functions to perform common tasks and operations. These functions can be used even without manual implementation.
Program library functions are pre-defined functions from the programming languages. These offer a set of proper operations and functionality. Likewise, they are also known as library functions or built-in functions.
The library of functions has implemented functions that you can use. They provide a way to perform common tasks efficiently, saving time and effort. Here are some examples of library functions in various languages:
Programming Language | Library Functions |
---|---|
Python |
‘print()’: Outputs a message or value to the console ‘math.sqrt()’: Calculates the square root of a number ‘str.upper()’: Converts a string to uppercase ‘len()’: Returns the length of a sequence ‘random.randit()’: Generates a random integer within a specified range |
JavaScript |
‘Math.random()’: Generates a random number between 0 and 1 ‘console.log()’: Outputs a message or value to the console ‘String.toLowerCase()’: Converts a string to lowercase ‘parseInt()’: Parses a string and returns an integer ‘Array.push()’: Adds elements to the end of an array |
C++ |
std: :cout’: Outputs a message or value to the console ‘std: :cin’: Reads input from the user ‘std: :sqrt’: Calculates the square root of a number ‘std: :string: :length()’: Returns the length of a string ‘std: :vector.push_back()’: Add elements to the end of a vector |
These examples show the commonly used library functions available in different languages. They can provide functionality for the following tasks:
- mathematical calculations
- input and output operations
- string manipulation
Using library functions can simplify programming tasks. Likewise, they can improve code readability and promote code reusability
Important
All languages have their respective standard libraries. For example, Python's standard library is extensive and covers various domains. On the other hand, JavaScript's standard library is well-suited for web development. It also has a rich ecosystem of third-party libraries. Lastly, C++ is focused on core language features functionality available through external libraries. The language choice and standard library often depend on the project's specific requirements and use cases.Import library functions in programming languages
You can import these library functions in the programming language. However, the process of importing libraries varies across different languages. Here are the ways to import libraries in some languages:
Python
In Python, the 'import' keyword is applied to import libraries. These are the different ways to import libraries:
- import library_name: This imports the entire library and allows you to access its functions and classes using the library name as a prefix.
- import library_name: as alias This imports the library with an alias, allowing you to use a shorter or more convenient name when accessing its functionality.
- from library_name import function_name: This imports a specific function from the library, allowing you to use it directly without using the library name as a prefix.
JavaScript
In JavaScript, the 'import' statement is used to import modules. However, it is important to note that its usage depends on the environment and module system being used. The environment refers to the browser (Node.js) and the JavaScript version (e.g. ES6 modules).
Here are some examples:
- import { function_name } from 'library_name': This imports a specific function from the library.
- import * as alias from 'library_name': This imports the entire library with an alias.
C++
In C++, the '#include' preprocessor directive is used to include libraries or header files. However, it is not used to import specific functions directly. Here are the common ways to include libraries or header files in C++:
- #include <library_name>: This includes the specified library or header file.
- using namespace library_name; :This statement is often used after including a library to avoid using the library name as a prefix when accessing its functionality.
You should note that the specific syntax and usage may vary. They depend on the language version and development software.
It is always advised to refer to the language document or specific resources. These can provide accurate and detailed information on importing libraries.
Ways to use program library functions in different languages
There are various ways to use these program library functions depending on the language. To use these functions from libraries, you can follow these general steps:
Python
- Import the library or module using the 'import' statement.
- Call the desired function from the library using the library name or an alias. Then follow the function name and any required arguments. Here is an example:
The 'math' library is imported in Python using this code's 'import' statement. The 'math' library provides various mathematical functions and constants. The 'sqrt' function from the 'math' library calculates the square root of the number 16. The result is stored in the variable 'result'.Finally, the value of 'result' is printed using the 'print' statement, which outputs the square root 16 to the console. The code demonstrates how to use the 'sqrt' function from the 'math' library to perform mathematical calculations.
JavaScript
- Import the module or library using the 'import' statement or by including the necessary script files.
- Access the desired function from the library using the appropriate syntax. It includes the object destructuring or the imported module's namespace. Here is an example:
This code uses an import statement to import the 'sqrt' function from the 'mathlibrary' library. The 'sqrt' function is a mathematical function that calculates the square root of a number.
The 'sqrt' function is then called with the argument '16', which calculates the square root 16. The result of the function call is stored in the variable 'result'. Finally, the value of 'result' is logged to the console using the 'console.log' function
This code shows how to import and use the 'sqrt' function from the 'math-library' module to calculate the square root of a number. You should note that the specific implementation and syntax may vary depending on the library. Likewise, you should note that the actual syntax and approach can vary. This depends on the JavaScript environment (e.g. Node.js or the browser) and the module system being used (e.g. CommonJS or ES modules).
C++
- Include the necessary header files using the '#include' directive.
- Use the functions from the library by calling them directly with any required arguments. Here is an example:&
The code includes two header files: 'iostream' and 'cmath'. The 'iostream' header file is for input and output operations. On the other hand, the 'cmath' header file is for mathematical functions.
The 'main()' function is the program's entry point. Inside the 'main()' function:
- A variable named 'result' of type 'double' is declared and initialised with the result of the 'std::sqrt()' function. The 'std::sqrt()' function is a part of the 'cmath' library and calculates the square root of a number.
- The calculated 'result' is then printed to the console using the 'std::cout' object and the << operator. The 'std::endl' manipulator inserts a new line after the output.
- The 'return 0;' statement indicates that the program has executed successfully and returns a value of 0 to the operating system.
This code shows how to use the 'std::sqrt()' function from the 'cmath' library to calculate the square root of a number and print the result to the console.
Pitfalls
There are some pitfalls that you should know when using these library functions. These can include the following:
- Not all libraries are compatible with every language or version. Ensure the library you want to use is compatible with your language and version.
- Library functions may not always be available in the standard library. Likewise, they may need more installation or dependencies. You must check the library document to ensure proper availability and installation.
- You must understand how to use the library functions and their parameters correctly. Improper usage may lead to unexpected results or errors. You can refer to the library document or examples for proper usage guidelines.
- Some library functions may have performance implications. These can depend on the input data's complexity and the size of the input data. Consider the performance impact of using specific library functions.
- When using many libraries, there may be compatibility issues between different libraries. Ensure the libraries you use are compatible and resolve any conflicts.
Once you finished applying various syntax, variables and functions, you can clarify the code's meaning. You can use various commenting techniques when clarifying the code's meaning. Commenting techniques refer to the practices and conventions used to add comments within code. These comments explain, document or clarify the meaning of the code. Commenting techniques are important for the following reasons:
- They help developers understand the code logic, intentions and implementation details. They serve as a form of self-documentation, making it easier to maintain, modify and debug code.
- They help collaboration among team members. Comments provide insights into the code's functionality and design choices. Likewise, they help the team communicate and share knowledge effectively.
- They provide information about the purpose of code segments, algorithms or data structures. Likewise, they explain the code's behaviour and usage. This makes it easier to use and extend the codebase.
- They can assist debugging by providing hints, explanations or notes about specific code sections. It can help identify and fix issues more efficiently.
- They improve code readability by breaking down complex logic into smaller parts. Likewise, they provide context and help readers follow the code's flow and intentions.
- They are essential for following coding standards, guidelines or regulatory requirements. They ensure code quality, consistency and compliance with best practices.
Examples of commenting techniques
Here are examples of commenting techniques you can use in various languages:
Single-line comments
These add explanatory text or notes on a single line within the code. Specific characters or symbols typically denote them.Likewise, these characters show a comment that the compiler or interpreter ignores. Here is an example of a single-line comment in Python:
The comment starts with the '#' symbol. Anything following it on the same line is considered a comment.
Multi-line comments
These comments add longer comments or block explanations spanning many code lines. They are enclosed between specific delimiters showing the comment block's beginning and end. Here is an example of this commenting technique in JavaScript:
The comment block starts with '/*' and ends with '*/', allowing many lines of text to be commented on.
Documentation comments
These comments are special comments used to generate code documentation. They can also provide more data about classes, methods or functions. They follow a specific format or syntax defined by the language or documentation generation tools. Here is an example in JavaScript:
The Javadoc comments in Java start with '/**' and end with '**/'. They provide detailed documentation about the method's purpose, parameters and return values.
TODO comments
TODO comments mark areas of the code where more work or improvements are needed. They serve as reminders for future enhancements or bug fixes.Here is an example of this comment in C++:
The comment starts with '// TODO:' and is followed by a description of the pending task.
Commented-Out code:
This comment refers to lines of code that are temporarily disabled by commenting them out. It is often used for testing, debugging or temporarily removing code without deleting it entirely. Here is an example of this comment in Python:
In this example, the codes 'x = 10' and 'print(x)' are commented out by adding '#' at the beginning of each line. This prevents the code from executing.
You can use these commenting techniques in various programming languages. Although there are differences in syntax or conventions, the concepts behind commenting are the same. You can adapt these commenting styles to most programming languages. However, you must consult the document or style guides of the programming language. They can provide precise details on comment syntax and conventions.
Guidelines for using comments effectively
Comments are an essential tool in programming. They improve code readability and aid in understanding. Likewise, they serve as explanatory notes within the code not executed by the compiler or interpreter. Here are some guidelines for using comments effectively:
- Documenting code intent: Comments should explain the purpose and intention behind codes, functions or complex algorithms. They help developers understand the reasoning and logic behind your code.
- Describing functionality: Comments can clarify the functionality of specific code sections or individual lines. It is particularly useful when dealing with intricate or non-obvious code that may not be immediately clear to others.
- Providing context: Comments can provide contextual information about the code, such as
It helps others to work with the code and make informed decisions.
- Assumptions
- Limitations
- Known issues
- Self-documenting code: The goal of self-documenting the code is to write code that is easy to understand without extensive comments. This can be achieved by:
- using meaningful variable and function names
- following coding conventions
- using good code organisation
- avoiding redundant comments
- Comments should not repeat what is already evident from the code itself. Instead, focus on explaining the why and how rather than the what.
-
Maintaining comments: You should keep comments updated as the code evolves. Outdated or inaccurate comments may lead to confusion.
Here are steps you can follow to clarify the meaning of the code using various commenting techniques:
Begin with a comment that provides an overview of the code's purpose or functionality. This can help other developers quickly understand the main objective of the code segment.
Your code can include complex algorithms, calculations or logic. You can use these comments to break down the steps and explain the reasoning behind each step. This can help other developers follow the logic and understand the code's intended behaviour.
Your code takes specific input or produces certain output. You can use these comments to describe the expected input format and the resulting output. This can help other developers understand how to interact with the code and what results to expect.
Use comments to explain the purpose and meaning of variables and functions. This is especially true if the names alone may not provide enough context. This can help other developers understand the role of each code's variable and functions.
If there are any considerations or limitations associated with the code, include comments to highlight them. These can include dependencies, known issues or performance considerations. This can help developers avoid pitfalls or make decisions when working with code.
Whenever you modify the code, ensure to review and update the comments accordingly. Outdated or misleading comments can be more confusing than helpful. Keeping the comments synchronised with the code ensures their accuracy and usefulness.
Quiz
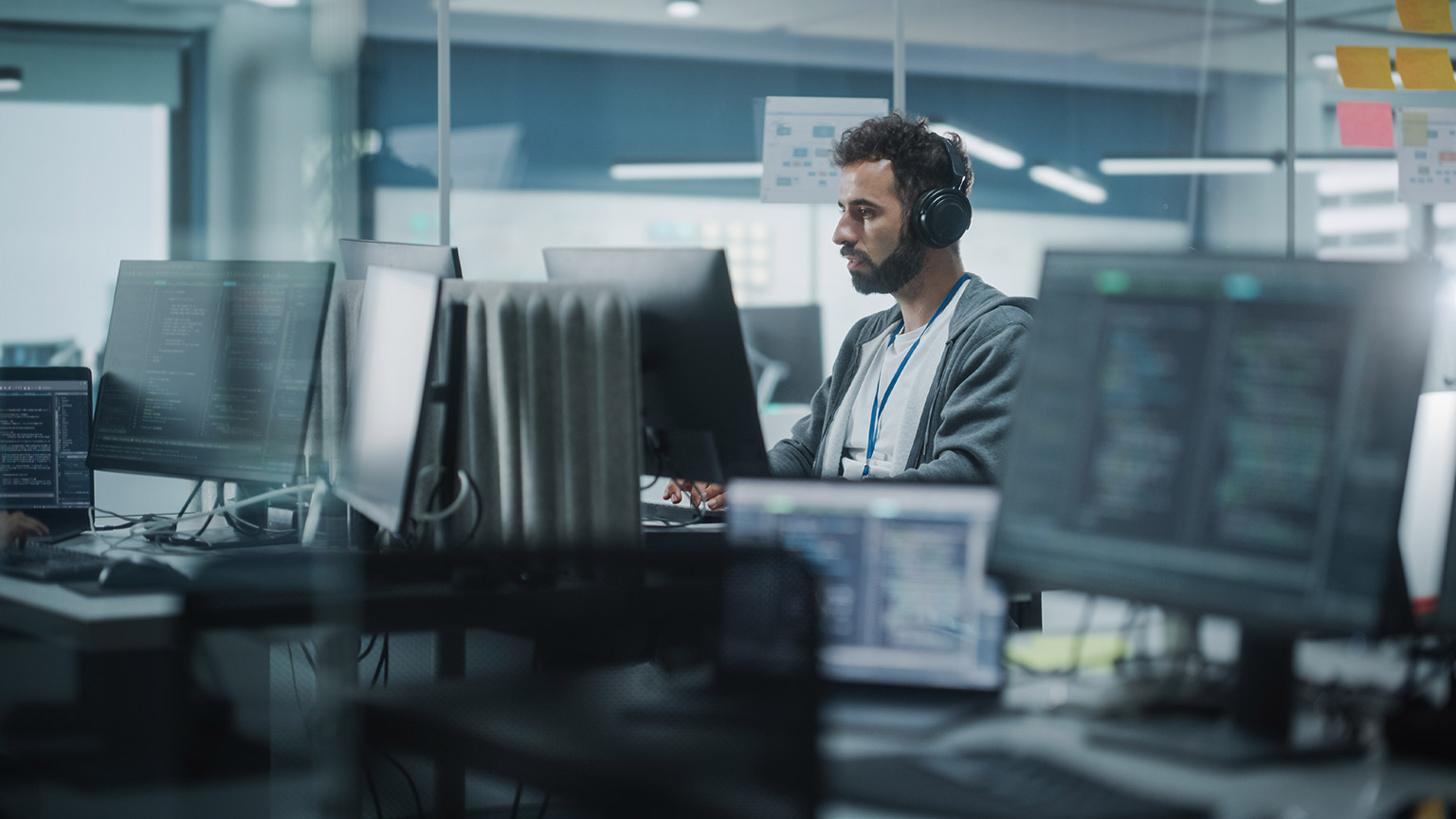