The control structures are integral to a language's syntax and layout. You learnt these syntaxes and layouts in the previous subtopic. They define the rules and syntax for creating conditional and iterative statements. Moreover, the syntax and layout of control structures determine how they are written, nested and formatted within the code.
Control structures refer to the mechanisms or constructs in languages. They allow the control and alteration of the flow of execution based on certain conditions. Moreover, they decide how codes are executed and repeated in different scenarios.
Control structures are important in creating an application. They allow you to decide and repeat actions based on different conditions. Likewise, they let you handle various scenarios and control the program's behaviour. Using these effectively can help you use complex logic and handle user input. You can also iterate over data and create an interactive and responsive app. These let you design flexible and intelligent programs that adapt to different situations.
As previously defined, syntax is rules and conventions defining the format of expressions in a language. Syntax provides a clear way to specify the order of execution for statements within a construct. It ensures that statements are executed in the intended construct. This allows you to control the flow of operations and achieve desired outcomes.
You can apply the language syntax in the following:
- sequencing constructs
- selecting constructs
- iterating constructs
Sequencing constructs
Sequence constructs refer to the arrangement and execution of statements in a specific order. They represent a linear sequence of instructions executed one after another. Likewise, they follow the order in which they appear in the code. Here are some examples:
In Python
In this example, the sequence construct prints the name and age variables in a specific order. The statements are executed one after another, producing the output, 'My name is John' and 'I am 25 years old'.
In JavaScript
In this example, the sequence construct computes the sum of two numbers and prints the result. First, the variables 'num1' and 'num2' are assigned values. Then, the sum is calculated and stored in the 'sum' variable. Finally, the result is printed using 'console.log()', displaying 'The sum is: 30'.
In C++
In this example, the sequence construct calculates the product of two numbers and displays the result. The statements are executed sequentially within the 'main()' function. The product is calculated and stored in the 'product' variable and then printed using 'std::cout', resulting in the output 'The product is: 15'.
Sequence constructs define the logical flow of operations in a program. Organising statements in a specific sequence can control the execution order. They ensure that each statement is processed in the desired sequence. Likewise, they allow you to perform tasks step-by-step, executing one operation after another. This is until you achieve the desired outcome.
In sequence construct, you can encounter the execution order and control flow of the syntax. Execution order refers to the chronological order of the statements during the program's runtime. It determines how the program progresses from one statement to another. Here is an example in Phyton:
In this example, the execution order is sequential. First, the value five is assigned to the variable 'x'. Then, 'y' is assigned the value of 'x + 2', 7. Finally, 'z' is assigned the value of 'x * y', 35. The value of 'z' is then printed, resulting in the output 35.
On the other hand, control flow refers to the path or direction that the program takes. It is based on evaluating conditional statements, loops and other control structures. Likewise, it determines the order in which different parts of the code are executed. These branch between different sections based on certain conditions. Here is an example in JavaScript:
In this example, the control flow is determined by conditional statements, such as 'if', 'else if' or 'else'. Different code blocks are executed based on the value of the 'grade' variable. If 'grade' exceeds or equals 90, the output is 'excellent'. The output is ' good ' if it is between 80 and 89. Otherwise, the output is 'average'. The control flow allows the program to decide and execute different code paths based on conditions.
Both execution order and control flow are basic concepts in programming. Execution order ensures that statements are executed predictably. On the other hand, control flow allows programs to decide and adapt based on conditions. Understanding and managing these are essential for creating functional and logic-driven apps.
You can follow the syntax rules and conventions specific to your language to apply language syntax in a sequence construct. Likewise, there are common practices that you can follow.
Common practices for applying programming language syntax in sequence constructs
Here are some common practices for applying programming language syntax in sequence constructs:
Each language has its syntax for writing statements. You must use the correct syntax for the statements you want to include in the sequence construct. For example, statements are typically written on separate lines in Python. On the other hand, they use semicolons to separate statements in JavaScript.
Indentation is important for readability and maintaining the code structure. Follow the indentation conventions of the language to ensure your code is properly formatted. For example, indentation using spaces or tabs is used to define blocks of code in Python.
Sequential code executes statements in the order they appear. You must arrange your statements in the desired order to achieve the desired sequence of actions. Be mindful of any dependencies between statements and ensure they are executed in the correct order.
When working with variables and data types, use the syntax specified by the language. Declare variables using the correct syntax and follow the naming conventions for data types and variables.
You must follow the syntax rules strictly to avoid syntax errors. Syntax errors occur when code does not conform to the expected syntax of the language. Check for error messages or warnings generated by your code editor or compiler. Moreover, you should fix any syntax errors before executing your program.
You must remember that syntax rules and conventions can vary between programming languages. It is vital to refer to the official document for the language you are working with. Following the correct syntax rules ensures your code is valid and error-free.
Selection constructs
Selection constructs are basic language features allowing decision-making and branching execution paths. They are based on certain conditions. Likewise, they are crucial in controlling the flow of a program and determining which actions to perform. These can include the following:
If statements
If statements evaluate a condition and execute a code block if the condition is true. The syntax of an if statement typically follows this pattern:
The condition is usually a Boolean expression that evaluates to either true or false. The code block inside the curly braces is executed if the condition is true. The code block is skipped if the condition is false, and the program continues with the next statement following the if statement. You can use conditional expressions and Boolean logic within if statements. These create more complex conditions. Here are examples of conditional expressions you can use:
Conditional Expressions | Code |
---|---|
Equality | a == b |
Inequality | a != b |
Less than | a < b |
Greater than | a > b |
Less than or equal to | a <= b |
Greater than or equal to | a >= b |
You can merge multiple conditions using Boolean logic operators, such as 'AND (&&)' and 'OR (||)'. These will create compound conditions.
Switch statements
Switch statements provide an alternative way to handle many possible outcomes based on the value of a variable or an expression. They allow you to specify different code blocks to be executed depending on the value of the switch expression.
Here is an example:
The switch expression is evaluated once, and the program flow is directed to the case that matches the value of the expression. If a matching case is found, the corresponding code block is executed. This is until the 'break' statement is encountered, which exits the switch statement. The code block under the 'default' label is executed if none of the cases matches.
Important
If statements are more flexible. They can also handle complex conditions involving logical operators and many variables. Moreover, they suit situations where you should make decisions based on diverse conditions. On the other hand, switch statements are useful when you have a single variable or expression and want to match its value against many cases. They provide a more concise and readable way to handle many possible values.
Apply programming language syntax in selection constructs
To apply programming language syntax in selection constructs, you can follow these practices:
- use descriptive and meaningful conditionals
- keep conditions simple and concise
- format your code properly
- add comments when necessary
- avoid code duplication
- consider readability over cleverness
- profile for performance optimisation
Ensure the conditions in your selection constructs are clear and easy to understand. Use variable names and logical operators that accurately represent the intended logic. This improves code readability and makes it easier to understand the code.
Avoid unnecessary complex conditions. Break down complex conditions into smaller, more manageable parts using Boolean logic operators like 'AND (&&)' and 'OR (||)'. It helps improve code readability and makes it easier to test and debug.
Use proper indentation and formatting to improve your code’s readability. Indent the code within the selection construct's code block to see it from the surrounding code. Stable indentation improves code readability and helps identify the code's structure.
Use comments to explain the purpose behind the selection construct or any nonobvious conditions. It helps developers understand the code and can be useful when the logic is complex or there are edge cases to consider.
If you have similar conditions or code blocks in many places, consider refactoring your code to remove duplication. Cloned code increases maintenance effort and the potential for introducing bugs. Extract common logic into functions or variables and reuse them where applicable.
While writing clever or concise code is tempting, prioritise code readability and clarity. The code should be easy to understand, not just for you but also for others who will maintain or modify it. Avoid cryptic or overly complex conditions that can confuse readers.
If performance is a concern, consider profiling your code to identify any bottlenecks. Selection constructs themselves are generally not performance-intensive. However, inefficiently written code within the code blocks can impact performance. Optimise the code within the selection constructs to improve overall performance if needed.
Iteration constructs
Iteration constructsrepeat a code block many times based on a specified condition. They allow for the execution of statements repeatedly until a certain condition is met. Likewise, it continues until you reach a specific termination condition.
The iteration constructs automate repetitive tasks and perform operations iteratively. They provide a way to handle scenarios where you must process a block of code many times without duplicating the code manually. It can streamline and optimise your code. Likewise, it improves efficiency and solves problems that involve repetition. These can include the following:
While loop
A while loop is a control structure that allows statements to be executed repeatedly if a given condition is true. The loop iterates as long as the condition remains true and terminates when the condition becomes false. The basic syntax of a while loop is as follows:
The condition is evaluated before each iteration, and the code block executes if it evaluates to true. After each iteration, the condition checks again, and if it still evaluates to true, the loop continues. The loop will only stop when the condition becomes false.
A common example is using a while loop to iterate through a list or array until a specific condition is met. It includes finding an element or performing a specific calculation.
For loop
A for loop enables the iteration over a sequence, such as a list, tuple or range of numbers, for a specific number of times. It provides a concise way to execute a code block repeatedly without maintaining an explicit loop counter.
The general syntax of a for loop is as follows:
The loop iterates over each element in the sequence, and the element is assigned to the variable. The code block inside the loop is then executed. This process continues until all elements in the sequence have been processed.
Do-while loop
The do-while loop is a control flow construct that allows a code block to be executed repeatedly until a certain condition is no longer met. Unlike other loop constructs, the do-while loop guarantees that the code block will be executed. This happens at least once before checking the termination condition. Here is the general structure of a 'dowhile' loop:
The code block within the 'do' section is the first to be executed, and the condition is evaluated after. If the condition is true, the loop will continue executing the code block. If the condition is false, the loop will terminate. Then, the program will move on to the next statement after the loop.
Apply programming language syntax in iteration constructs
To apply programming language syntax in iteration constructs, you can follow the practices below:
- Choose descriptive names for loop variables to enhance code readability and understanding. When you create a loop in your code, it is important to use variable names that clearly show their purpose. Instead of using single-letter names like 'i' or 'x,' choose names that describe what the variable represents.
- Initialise loop variables before entering the loop. When initialising loop variables, it is crucial to use meaningful names and correct data types. It can also set initial values that match the loop's purpose and clear prior values to prevent errors. Likewise, it aligns with loop logic, accounts for termination conditions and documents the process. This practice ensures a dependable starting point and boosts code clarity. It also minimises the chance of errors or unexpected outcomes.
Create loop conditions that accurately represent the desired termination condition. It is important to craft clear and logical termination conditions. This ensures loops end as intended and avoids problems like infinite loops. Likewise, this entails creating expressions aligned with defined criteria, including relevant variables. It also includes testing for correctness and edge cases and documenting the conditions.
Update the loop variables within the loop body to ensure progress towards the loop termination condition. Inside your loop, ensure to update the loop variable according to the logic of your program. This update is what allows the loop to progress towards its termination condition. For example, if you are counting the number of items in a list, you might increment your loop variable by '1' in each iteration. This step ensures that your loop does not run indefinitely and stops when the desired condition is met.
This principle prevents the infinite loops, where a loop continues indefinitely without halting. Infinite loops can lead to resource exhaustion and unresponsive programs. This causes a negative impact on system performance. It ensures that loop termination conditions will eventually evaluate to false.
- Keep the code within the loop body concise and focused. Extract complex logic into separate functions for better maintainability. By trimming needless elements and staying focused on the loop's intended tasks, you enhance code clarity. Likewise, you can streamline debugging and foster efficient execution. This results in more effective and manageable programming.
- Test your iteration construct with various inputs, including the minimum and maximum values. This is to verify that it behaves as expected in all scenarios.
- Use break and continue statements sparingly. Break statements can be used to exit a loop prematurely, while continue statements can skip the current iteration and move to the next one. Ensure their usage aligns with the intended logic.
- Depending on the language and context, you might optimise loop performance. You can minimise unnecessary calculations or reduce the number of iterations. It is a good practice to test your loop with different kinds of inputs. Try using small, large and edge-case inputs to see how your loop behaves in different scenarios. This helps you catch any issues or unexpected behaviours that might arise under specific conditions. For example, if your loop works fine with small numbers but crashes with large numbers, you know there is something to fix.
Follow the syntax and style guidelines of the language. This is to maintain consistency and improve code readability.
You should remember that there are differences in syntax across languages. However, the core concepts of these constructs remain the same. You should be familiar with the specific syntax and conventions of the language to use these constructs effectively.
The language syntax is crucial for selection, sequence, and iteration constructs. It defines the rules and conventions for writing code that the language can execute correctly. Likewise, it provides a structured way of expressing these constructs. This ensures that the code is interpreted or compiled accurately. The correct syntax is essential for the proper functioning of the program. It also maintains code readability and maintainability.
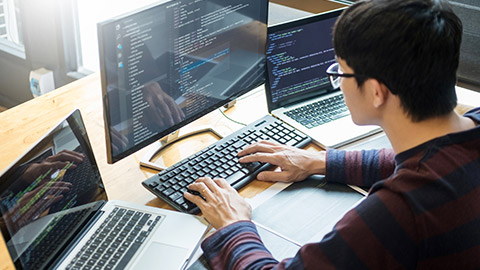
Expressions are combinations of literals, identifiers, operators and function calls. They represent computations or calculations within a program. You can create these expressions in the selection and iteration constructs. In creating these expressions, you can use logical operators.
Logical operators are symbols or keywords used to combine or manipulate Boolean values in programming. They allow for the evaluation of logical expressions and help control the flow of execution based on certain conditions. They are significant in both selection and iteration constructs in programming.
In selection and iteration constructs, logical operators are crucial in combining conditions. They are also vital in controlling the execution flow. Here are the logical operators you can use:
AND operators (&&)
The '&&' operator combines two or more conditions. It returns true only if all the conditions are true.
In using these operators, you can use a truth table. A truth table shows the possible combinations of truth values for a logical expression or a set of propositions. It provides a systematic way to analyse and check the truth or falsehood of a compound statement. This is based on the truth values of its component propositions.
Here is an example of the truth table for AND operators:
Condition 1 | Condition 2 | Result |
---|---|---|
True | True | True |
True | False | False |
False | True | False |
False | False | False |
The AND operator evaluates the conditions from left to right and stops when encountering a false condition. If all conditions are true, the final result is true.
OR operators (||)
The '||' operator combines two or more conditions, and it returns true if at least one of the conditions is true.
Here is an example of the truth table for OR operators:
Condition 1 | Condition 2 | Result |
---|---|---|
True | True | True |
True | False | True |
False | True | True |
False | False | False |
The '|| ' operator evaluates the conditions from left to right and stops when encountering a true condition. If all conditions are false, the final result is false.
NOT operators (!)
The '!' operator is a unary operator used to negate the Boolean value of an expression. It reverses the logical state of the expression. Here is an example of the truth table for NOT operators:
Condition | Result |
---|---|
True | False |
False | True |
The ! operator flips the Boolean value of the expression. If the expression is true, the result is false, and vice versa.
Combining logical operators
You can use a nested if statement or chained conditions to combine logical operators. Nested if statements allow for complex conditions to be evaluated step by step. Each nested if statement represents a separate condition.
Example
Here is an example:
This example shows a nested if statement. The outer if statement checks if 'x' is greater than '0'. If it is, the inner if statement is evaluated to check if 'y' is greater than '0'. Depending on the outcomes of the conditions, different messages are printed.
On the other hand, you can use logical operators to chain conditions together in a single if statement. It allows for many conditions to be evaluated simultaneously. The condition is true if any of the chained conditions is true. Here is an example:
In this example, we use the AND operator to chain two conditions together in a single if statement. The condition checks if 'age' is greater than or equal to 18 and less than or equal to 65. If the condition is true, the message 'You are of working age' is printed. If any of the conditions is false, the code inside the if statement is not executed.
Variations in syntax and behaviour
Logical operators are commonly used in creating expressions in constructs across different languages. While the basic purpose of these operators remains the same, there can be some variations in syntax and behaviour. These differences include the following:
- The symbols used for logical operators may vary between programming languages. For example, some languages use '&&' and '||' for AND and OR operators, while others use keywords like 'and' and 'or'.
- The priority and combining of logical operators can differ between languages. They determine the order in which operators are evaluated when they appear together in an expression.
- Different languages may handle type coercion differently when using logical operators. For example, some languages may automatically convert non-Boolean values to Boolean values. On the other hand, others may need explicit type conversions.
Create expressions in selection constructs using logical operators
To create expressions in selection constructs using logical operators, you can follow these steps:
- Determine the conditions you should check for the selection construct. These conditions can be comparisons, Boolean values or any expressions that yield a Boolean result.
- Choose which logical operator is suitable for expressing the relationship between the conditions. You learnt these in the previous discussion.
- Use the selected logical operator to combine the conditions into a single expression. You can use parentheses to group conditions and establish the desired order of evaluation.
- Evaluate the expression. The expression will yield a Boolean value based on the logical evaluation of the conditions.
- Apply the resulting expression in the appropriate selection construct. This is to determine the flow of execution based on the Boolean result.
Examples
Using ‘AND’ operators (&&)
In this example, the 'AND' operator creates an expression that checks if both 'condition1' and 'condition2' are true. If both conditions are true, the code block inside the 'if' statement will be executed.
Using ‘OR’ operators (||)
In this example, the 'OR' operator creates an expression that checks whether 'condition1' or 'condition2' is true. If either condition is true, the code block inside the 'if' statement will be executed.
Using ‘NOT’ operators (!)
In this example, the 'NOT' operator creates an expression that negates the value of 'condition'. If the 'condition' turns false, the code block inside the 'if' statement will be executed.
These examples show how logical operators can combine conditions in selection constructs. Evaluating the resulting expressions can control the flow of execution in your program. These are based on the condition's truth or falsity.
Use logical operators to make expressions for iteration constructs
You can also use logical operators to make expressions for iteration constructs. They provide a way to control the flow and behaviour of loops based on specific conditions. Here are ways you can use logical operators in iteration constructs:
Using logical operators in while loops
You can use logical operators in the loop condition to control when the loop should end. Combining conditions with logical operators can specify complex termination conditions. This depends on many variables or states. Here is an example:
In this example, the while loop continues if the variable 'x' is less than or equal to 10 and is an even number. The logical condition 'x <= 10 and x % 2 == 0' is evaluated at each iteration to determine whether to continue the loop.
Using logical operators in for loops
You can use logical operators within the loop body to filter or skip certain iterations based on specific conditions. Evaluating logical conditions can decide whether to execute or skip certain statements. Here is an example:
In this example, the for loop iterates if the variable 'i' is less than five or is an even number. The logical condition 'i < 5 || i % 2 === 0' is checked at the beginning of each iteration, and if the condition is true, the loop body is executed.
Using logical operators in do-while loops
You can use logical operators in the loop condition of a do-while loop to control when the loop should continue or end. The loop body is executed at least once, and the loop condition is checked. If the condition is true, the loop continues; otherwise, it terminates.
Example
In this example, the do-while loop continues executing the loop body if the variable 'num' is less than or equal to 10 and is not an even number. The logical condition 'num <= 10 && num % 2 != 0' is evaluated at the end of each iteration to determine whether to continue or end the loop.
Steps to create expressions in iteration constructs using logical operators
To create expressions in iteration constructs using logical operators, you can follow these general steps:
- Identify the condition that will control the loop iteration. This condition can involve one or more logical operators.
- Choose the appropriate loop construct based on your language and the code requirements. You learnt these loop constructs in the previous discussion.
- Use the logical operators to set up the condition within the loop construct. This condition will be evaluated at each iteration to determine whether to continue or end the loop.
- Define the statements or code blocks executed during each iteration of the loop.
- Test your code and ensure the logical condition correctly evaluates the desired conditions. You should adjust or refine the expressions or logical operators.
Precedence of logical operators
Understanding the order in which logical operators are evaluated is important. This is especially for writing correct expressions. Operator precedence rules determine the priority of operators in an expression. They specify which operators are evaluated first. In most languages, logical operators have lower precedence than arithmetic and relational operators.
The typical precedence of logical operators includes the following:
- Parentheses: Expressions within parentheses are evaluated first.
- NOT operator (!): It has the highest precedence among logical operators and is evaluated before the other logical operators.
- AND operator (&&): It has higher precedence than the OR operator and is evaluated before it.
- OR operator (||): It has the lowest precedence among the logical operators and is evaluated last.
To ensure the desired order of evaluation, parentheses can be used to group expressions. They override the default precedence. Using parentheses can specify which parts of the expression should be evaluated together.
Watch
Watch this video to learn more about the control structures:
Quiz
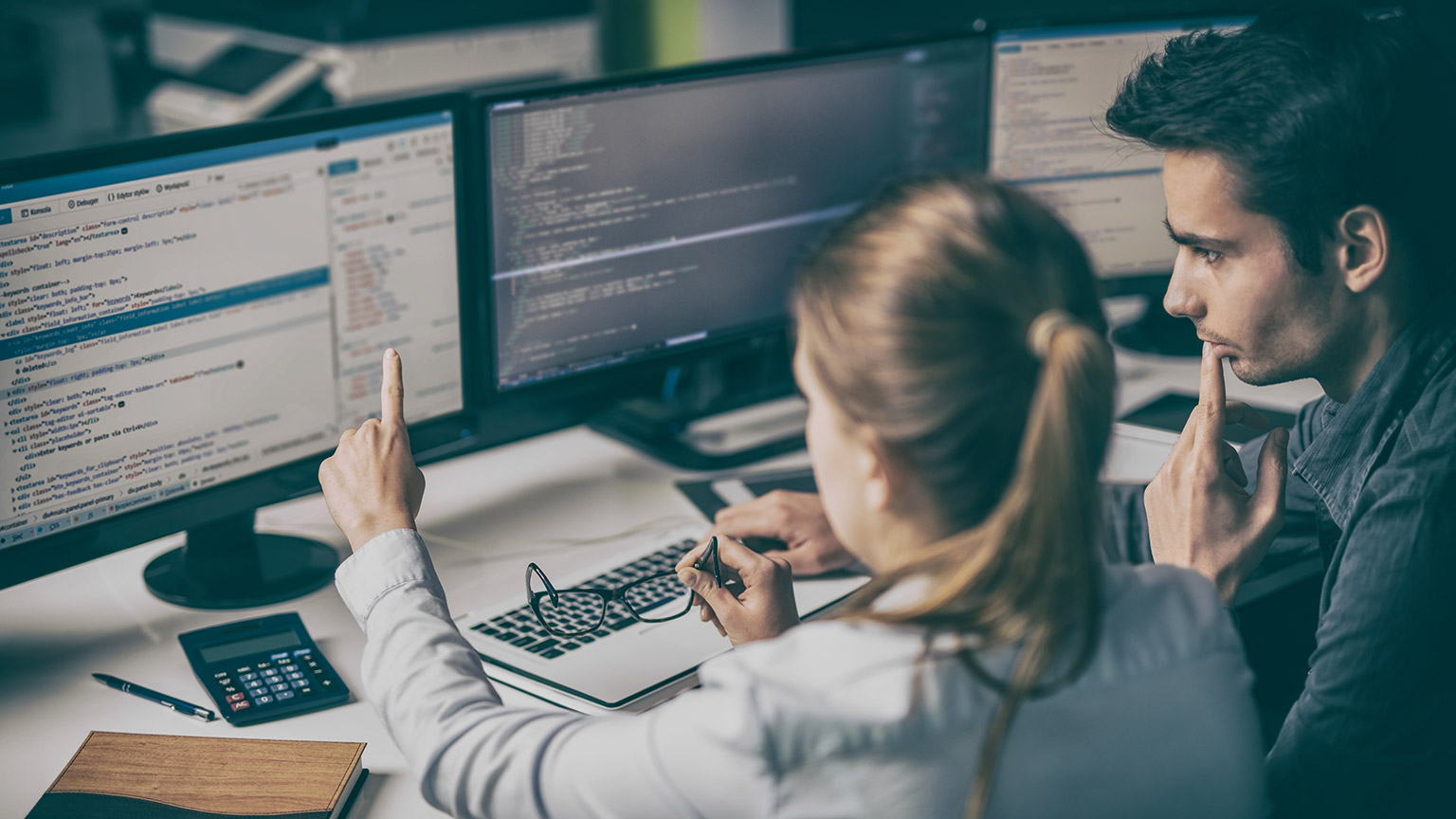