JavaScript is the most used programming language globally - nearly every web page uses JavaScript in some way or another.
As a UX Designer, JavaScript has appealing properties for interactivity and limiting lines of code (meaning faster loading web pages).
In this topic we will cover:
- what JavaScript does
- basic tools
- jQuery.
JavaScript itself is just a programming language. What that language can do depends on the environment it is being used in.
JavaScript was created in 1994 by Brendan Eich, working at Netscape to add interactivity and behaviour to web pages. JavaScript can react to events such as mouse clicks and keypresses in this environment and change the page's elements accordingly. JavaScript can even talk directly to the webserver, passing data back and forth.
More recently, JavaScript is also being used on web servers in the form of Node.js applications to generate web pages, read and write files, interact with databases and other web servers, among other server-side application tasks. Node.js can do everything other languages such as PHP, ASP.NET and Java can do.
Developers also use Node.js outside of the server environment as a powerful scripting and automation tool.
The Web Browser
Browsers are simply programs designed to interpret client-side code to create web pages to display. The code, along with the content, is separated by the browser into four layers:
- Content (text, pictures, video, audio) – Plain Text
- Document Semantics or 'markup' (headings, paragraphs, links, etc.) – HTML
- Presentation Rules (font, colour, position, etc.) – CSS
- Behaviours (interactivity, data handling, etc.) – JavaScript
JavaScript adds behaviour to web pages. This can be as simple as doing something when the page loads or causing something to happen when different page elements are clicked, dragged, or written into, for example, error messages that appear when a form field is filled in incorrectly.
JavaScript for the Web Browser
Where to write JavaScript?
- In an HTML file, placing script between
<script></script>
tags. - In a separate .js file, referenced by HTML files.
JavaScript can be written directly into the "developer console" when developing.
HTML Files
We can include JavaScript directly inside an .html file with the following code:
<script type="text/javascript"> alert("Hello, world!"); </script>
1. JavaScript files
We can also keep our JavaScript in external files and include those files on any pages that need it.
<script src="js/form-validation.js" type="text/javascript"></script>
Note: Any script inside the script element is ignored when the src attribute is present. You can write comments here if you must, but actual code will be ignored.
Aside: In older versions of Internet Explorer, the contents of the linked file would essentially be copied from the file and placed between the <script></script>
tags as if it had been there all along. This made working JavaScript in IE harder as the browser would report incorrect line numbers with errors.
Best Practice: All script tags should live just above the closing </body>
tag. This lets the browser focus on downloading CSS, Images, and other external resources before downloading and executing scripts, speeding up the page load time.
Tired Practice: Old HTML resources will recommend you put your <script>
elements in the <head>
element. However, this can potentially have a negative impact on the page's loading speed.
Worst Practice: JavaScript attributes:
<a href="javascript:alert('nope');">...</a>
<span onclick="alert('nope');">...</span>
In the same way in CSS you want to use class attributes over style attributes and separate your presentation layer from the document. Avoid writing JavaScript in attribute form like this. Keep your behaviour layer separate from your document and presentation layers.
The Extra Mile: The attributes async and defer can give better control over how your code will load. Research and discuss why these options have been provided
2. Developer Console
The console is a place where you can type JavaScript and see what it does straight away. What you type can even change the web page you have open.
Each web browser has a developer’s console built-in. Some browser’s consoles are more powerful than others.1
Exercises
- Locate the developer's console in three web browsers:
- Google Chrome
- Firefox
- Internet Explorer or Safari
- Find where you can type the following code into, and run it.
alert("Hello, world!");
- Discover and memorise the keyboard shortcuts for toggling the JavaScript Console for your three web browsers.
JavaScript syntax/tags
JavaScript can be implemented using JavaScript statements that are placed within the <script></script>
tags in a web page.
You can place the <script></script>
tags containing your JavaScript anywhere within your web page, but it is normally recommended that you link it to an external JS sheet in the <head></head>
tags.
The <script></script>
tags alert the browser program to start interpreting all the text between these tags as a script. A simple syntax of your JavaScript will appear as follows.
<script type="text/javascript"> // JavaScript code </script>
Objects
The JavaScript object data type can contain many values as key: value;
pairs. These pairs provide a useful way to store and access data. The object literal syntax is made up of name:value pairs separated by colons with curly braces on either side {}
.
Typically used to hold data that are related, such as the information contained in an ID, a JavaScript object literal looks like this, with whitespaces between properties:
var jessica { firstName: "jessica", lastName: "smith", color: "red", location: "sand" };
Alternatively, and especially for object literals with a high number of name:value pairs, we can write this data type on multiple lines, with a whitespace after each colon:
var jessica { firstName: "jessica", lastName: "smith", color: "red", location: "sand", };
The object variable jessica in each of the examples above has 4 properties: firstName
, lastName
, color
, and location
. These are each passed values separated by colons.
Forms provide you with a way of grouping together HTML interaction elements with a common purpose. For example, a form may contain elements that enable the input of a user’s data for registering on a website. Another form may contain elements that enable the user to ask for a car insurance quote. It is possible to have a number of separate forms in a single page. You don’t need to worry about pages containing multiple forms until you have to submit information to a web server—then you need to be aware that the information from only one of the forms on a page can be submitted to the server at one time.
To create a form, use the <form>
and </form>
tags to declare where it starts and where it ends. The form
element has a number of attributes, such as the action attribute, which determines where the form is submitted; the method attribute, which determines how the information is submitted; and the target attribute, which determines the frame to which the response to the form is loaded.
Generally speaking, for client‐side scripting where you have no intention of submitting information to a server, these attributes are not necessary. For now, the only attribute you need to set in the form
element is the name attribute to reference the form.
So, to create a blank form, the tags required would look something like this:
<form name="myForm"></form>
You will not be surprised to hear that these tags create an HtmlFormElement
object, which you can use to access the form. You can access this object in two ways.
First, you can access the object directly using its name—in this case document.myForm
. Alternatively, you can access the object through the document object’s forms collection property. You can manipulate this like a document object’s images collection. The same applies to the forms collection, except that instead of each element in the collection holding an HtmlImageElement object, it now holds an HtmlFormElement (hereby called simply Form) object. For example, if it’s the first form in the page, you reference it using document.forms[0]
.
Many of the attributes of the form
element can be accessed as properties of the HtmlFormElement
object. In particular, the name property mirrors the name attribute of the form
element.
The forms collection
In this Try It Out, you’ll use the forms collection to access each of three Form objects and show the value of their name properties in a message box. Open your text editor and type the following:
<!DOCTYPE html> <html lang="en"> <head> <title>Example 1</title> </head> <body> <form action="" name="form-1"> <p>This is inside form-1</p> </form> <form action="" name="form-2"> <p>This is inside form-2</p> </form> <form action="" name="form-3"> <p>This is inside form-3</p> </form> <script type="text/javascript"> var numberForms = document.forms.length; for (var index = 0; index < numberForms; index++) { alert(document.forms[index ].name); }; </script> </body> </html>
Save this as example1.html. When you load it into your browser, you should see an alert box display the name of the first form. Click the OK button to display the next form’s name, and then click OK a third time to display the third and final form’s name.
Within the body of the page you define three forms. You give each form a name and a paragraph of text.
In the JavaScript code, you loop through the forms collection. Just like any other JavaScript array, the forms collection has a length property, which you can use to determine how many times you need to loop. Actually, because you know how many forms you have, you can just write the number in. However, this example uses the length property, because that makes it easier to add to the collection without having to change the code. Generalizing your code like this is a good practice to get into.
The code starts by getting the number of Form objects within the forms collection and storing that number in the variable numberForms:
var numberForms = document.forms.length;
Next you define the for loop:
for (var formIndex = 0; formIndex < numberForms; formIndex++) { alert(document.forms[formIndex].name); };
index < numberForms
Remember that because the indexes for arrays start at 0
, your loop needs to go from an index
of 0
to an index
of numberForms - 1
. You enable this by initializing the index
variable to 0
, and setting the condition of the for loop to index < numberForms
.
Within the for loop’s code, you pass the index
of the form you want (index
) to document.forms[]
, which gives you the Form object at that index in the forms collection. To access the Formobject’s name
property, you put a dot at the end of the name of the property, name.2
jQuery is a JavaScript library. Be sure to check out their API documentation.
Single page website.
Instructions
This activity will aim to develop your technical skills further.
As we have already explored the early stages of the UX Process, this activity will focus on the design and implementation.
Task
Select an existing multi-page website to reimplement as a single-page website.
Steps
- Research the design patterns you think would be suitable for the site
- Quickly sketch a wireframe, get feedback, design a mockup and get feedback
- With design patterns in mind, complete the layout of page sections using a front-end framework and build in a layer of style
- Create media queries
- Use JS plugins to improve usability.
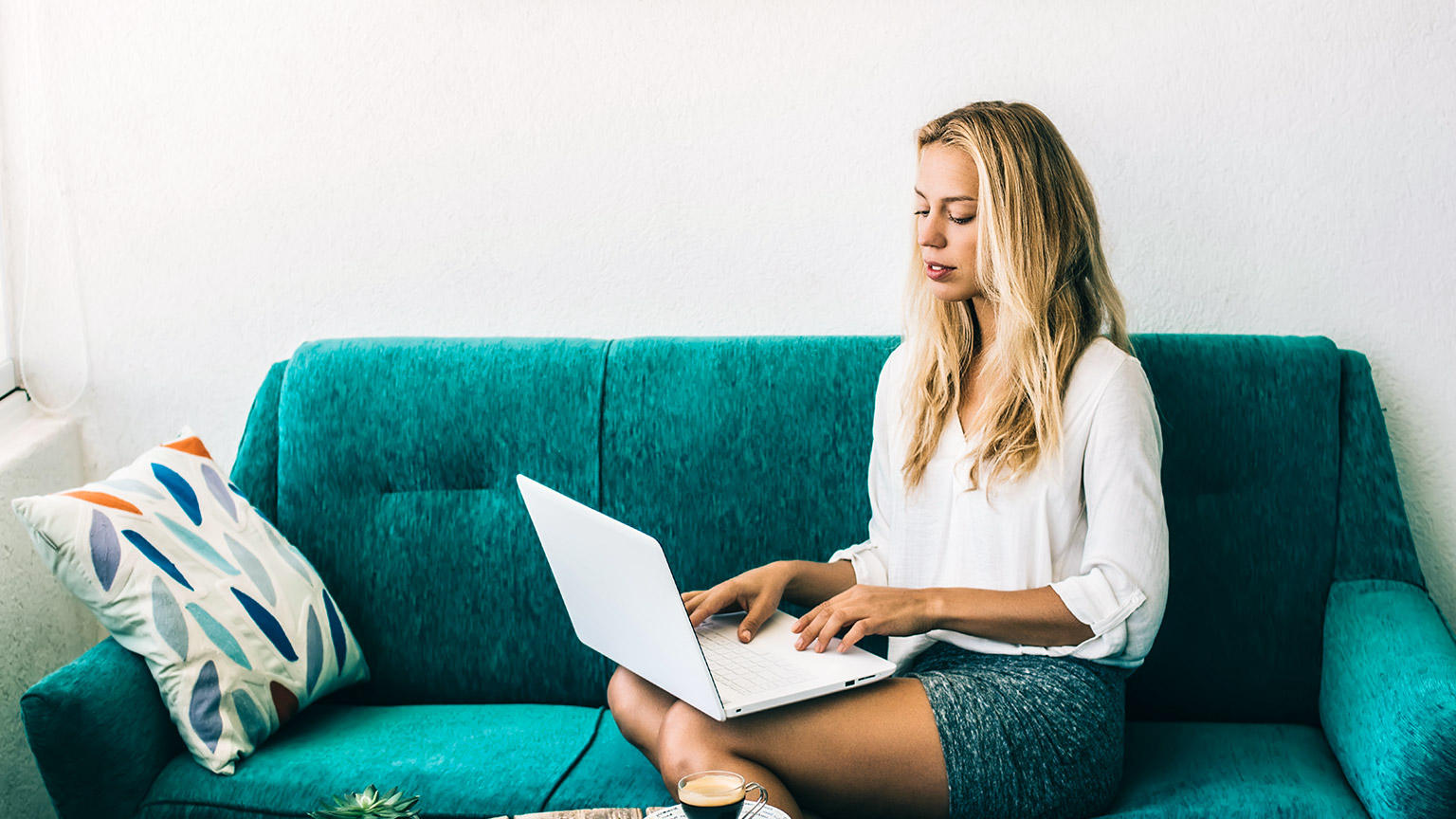