A familiar science fiction trope is the concept of the Universal Translator. In Star Wars it is C3PO, in the Hitchhiker’s Guide to the Galaxy it is the Babelfish, and Captain Kirk has his gadget. The concept of the universal translator is simple – it is a device (or in the case of the Hitchhiker's Guide a little fish you put in your ear!) that can translate between two languages as quickly as possible.
That is what programming languages do – they take the instructions software developers write and translate them into the ones and zeroes of the binary language computers understand, and therefore operate. The act of writing these instructions is called ‘programming’ or ‘coding’
In this section of the course, you will be introduced to some of the fundamentals of programming language, and in your workshop, you will practice and start developing fluency in programming language and coding.
Watch - What is a programming language? (3:21 minutes)
To start this section, watch and learn about the function of programming languages.
In the video the distinction was made between low-level languages and high-level languages. Do you remember the distinction?
Low-level languages more closely resemble the language understood by a computers CPU. The drivers for your printer, and other hardware peripherals use instructions written in low-level languages.
In this course and throughout the programme you will be learning and using high-level programming languages.
Understanding the relationships between programme languages, software engines and IDE’s is important. Which ones you choose is affected by the type of product being developed (e.g., is it a game or something else), the device the application will run on, and the IDE you will use.
Let’s start by defining each of these concepts.
Programme Language:
This is the language in which you write your code that instructs the computer what to do. Examples of commonly used languages include:
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
HTML5 | Python | C++ | C# | JavaScript | SQL |
---|
Engines:
Software engines are programs that perform a core or essential function for other programs. They are uses in operating systems, subsystems or application programmes to coordinate the overall operation of other programmes. Examples are game engines and database engines.
IDE:
The Integrated Development Environment is a software application that contains development tools like text editors, code libraries, compilers, build automation tools and debuggers. They provide a user interface for code development, testing and debugging. They help to make software development easy and standardised based on the particular programme language you are using.
It is important to note the programming language you use may determine the correct engine and IDE you need to use.
The following chart provides a quick overview of which languages, engines, and IDE work with each other.
Language | Engine | IDE |
---|---|---|
HTML5 | Any engine | Boilerplate Notepad++ Atom |
CSS | Any engine | Sass |
Javascript | Unity | Mono-develop Eclipse |
C# | Unity | MS Visual Studio Mono-develop |
SQL | Any engine | MySQL Workbench |
Lua | Corona Unity3D | Mono-develop MS Visual Studio |
Conduct your own research on the programme languages, engines, and IDEs. Your tutor will provide instruction and learning on certain languages, and you will have to opportunity to use this learning as you complete the required assessment tasks.
Can you pick the real programming language?
Click on the link below and see if you can identify which are real programming languages from this list. It’s just a bit of fun
C++ was developed by Bjarne Stroustrup in 1979. It is one of the most widely used programming languages and is used across a variety of platforms and applications in different domains. It was first called C with classes, becoming C++ in 1983
C++ is a high-level general-purpose programming language which syntactically similar to English, and popularly known as an extension of C (another programming language. It is a highly portable language, and it is the language of choice for developing multi-platform applications.
C++ gives the user complete control over memory management, so if you are developing an application which needs memory-efficient coding then C++ is the go-to language of choice.
Its compatibility with C acts as an advantage for converting legacy software to an object-oriented language.
It is a compiled language which means that the code goes through a pipeline of software tools which takes the code written by the programmer, interprets it so the computer understands and produces the output the user expects to see and engage with. You will be prompted to download and install a software application that has a suite of tools that you will use.
Applications of C++ Programming
The presence of C++ is felt in almost every area of software development. Some examples of its ubiquity are listed below:
Application Software - Almost all the major operating systems (Windows, Mac OS and the various versions of UNIX like Linux and Ubuntu) were developed using C++. In addition of the core part of many browsers like Mozilla Firefox and Chrome have been written using C++. The language has also been used in the development of MySQL, one of the most popular database systems used.
Programming Languages - C++ has been used extensively in developing new programming languages like C#, Java, JavaScript, Perl, UNIX’s C Shell, PHP and Python.
Computation Programming - Because of its fast speed and computational efficiencies C++ is used in the building of computerized solutions for scientific analysis and modelling.
Games Development - C++ is extremely fast which allows programmers to do procedural programming for CPU intensive functions and provides greater control over hardware. Because of this it has been widely used in the development of gaming engines like Unity, Unreal and Frostbite.
Embedded Systems - C++ is the language used to program embedded systems hardware. You encounter examples of embedded systems in your car, or the GUI on your phone. For a brief explanation, refer to this article on embedded systems.
These are just examples of some of the applications and areas in which C++ is used as a programming, and aptly demonstrates its popularity, utility and versatility.
Before you start ….
You will need to download and install Microsoft Visual Studio. You will use this software throughout this course and beyond.
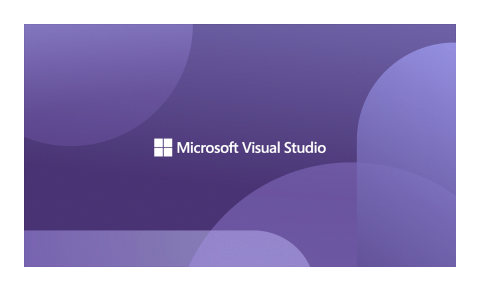
Install Visual Studio with C++ - Community version. A Mac version is also available for download.
Follow the installation instructions.
When prompted you should also install the following optional features. You may not need most of these for the work in this module but will do over the duration of the Diploma:
- Microsoft Visual Studio Workload Managed Desktop
- Microsoft Visual Studio Workload Native Desktop
- Microsoft Visual Studio Workload Managed Game
- Microsoft Visual Studio Workload Python
- Microsoft Visual Studio Workload Native Mobile
- Microsoft Visual Studio Workload Data Science
- Microsoft Visual Studio Component Class Designer
For further information on setting up Visual Studio, refer to this Install Visual Studio documentation.
The Basic Structure of C++
The structure of any C++ program must adhere to a number of rules and conventions. As you learn programming you will become more and more acquainted with the intricacies and power of C++.
The structure of the program is important, and must have the following structural features:
- Documentation Section
- Preprocessor Section
- Header or Standard Files
Documentation Section
This section is used to document program functionality.
The documentation section is where comments about what the underlying code are written. It is not mandatory to include a documentation section but including the section helps programmers and users understand the program code easily. Comments are not compiled by compiler; they are ignored. So, they don’t have any syntax and we can write anything in the comment section.
In large programs with thousands of lines of codes, comments are used to minimize confusion and increase readability.
There are two types of comments:
-
Single-line comment:
// I'm a single-line comment
-
Multi-line comment:
/* I'm a multi-line comment */
Preprocessor Section
In a C++ program, the statements which are starting with the “#” symbol are called preprocessor directives. The program provides a lot of inbuilt preprocessor directives that are at hand. This section is also called the Link Section.
#define
This directive defines a sequence of characters, called symbols. It is led by preprocessor before the compiler starts execution
#warning
This allows generating a level one warning from a specific location in your code.
Header or Standard Files
These files contain definitions of pre-defined functions like cin , or cout . These files must be included for working with these functions.
Different functions are declared in different header files. For example, standard I/O functions are in an iostream
file whereas functions which perform string operations are in a string
file.
Use this syntax: #include<file_name>
For Header Files:
#include<string>
if you're going to be using the string class#include<iostream>
when you want to usecin
andcout
.#include<math.h>
Perform mathematical operations likesqrt()
andpow()
.#include<ctype.h>
Perform character type functions likeisaplha()
andisdigit()
#include<conio.h>
Perform console input and console output operations likeclrscr()
to clear the screen andgetch()
to get the character from the keyboard.#include<fstream>
when you want to read or write files.
Watch - the basic structure of a C++ programme (12:31 minutes)
In the video below the presenter talks about the basic structure of a C++ programme, and goes through the lines of code step by step with explanations. He discusses syntax and the use of cin
and cout
.
A data type constrains the values that an expression, such as a variable or a function, might take. They define the operations that can be done on the data, the meaning of the data, and the way values of that type can be stored.
There are several types of data in programming:
- Numeric data
- Integer
- Float
- Character
- All characters (a to z, A to Z)
- Boolean
- This data type returns one of two possible values –
true
orfalse
- This data type returns one of two possible values –
It is declared with the keyword bool and returns the values true = 1
and false = 0
.
See the example below:
bool isSnowboardingFun = true; bool isSteakTasty = false; cout << isSnowboardingFun // => 1 (true) cout << isSteakTasty // => 0 (false)
C++ data types can be put into one of two categories:
Primitive
Data types that specify the size and type of variable values
These are the basic data types available in C++. Also called ‘built-in data types’. There are 7 basic data types:
- Boolean
- Character
- Integer
- Floating point
- Double floating point
- Valueless
- Wide character
Non-primitive
Derived data types and user defined data types. These refer to objects and call methods to perform certain functions.
Keywords for user-defined data types include struct, enum, union, class and typedef
For Derived data types include array, linked list, pointers and reference. These data types will be discussed later in this module and in CS103 Integrated Studio I
Explore Further
For some further explanation of data types, review Educba (2020) Introduction to C++ Data Types. C++ Programming Tutorial.
Tutorialspoint (n.d.) C++ Data Types also provides further explanation of data types, variable sizes and range, and the modification of data types, along with examples of coding using data types.
In this section we will stop to consider C++ keywords and identifiers. Note the relationship between these different features of C++
Keywords
C++ keywords are also known as reserved words. They are predefined words which are reserved; therefore, they cannot be used to name a variable, method, class or any other identifiers. These words are defined by the compiler to perform the internal operation and have a meaning that is defined by the compiler to accomplish a task in code. There are 64 keywords in total.
Keywords cannot be used for declaring class names, object names, variable names or function names.
Listed below are data types encountered in the last section with the associated keywords given.
Type | Keyword |
---|---|
Boolean | bool |
Character | char |
Integer | int |
Floating point | float |
Double floating point | double |
Valueless | void |
Wide character | wchar_t |
This article provides a complete list of keywords, and some coding examples with discussion.
Identifiers
The name of the various user-defined objects in C++ are called identifiers. These names are unique and can be short (x
or y
) or descriptive (e.g., totalVolume
, age
). Descriptive identifiers should be used wherever possible as it helps to create understandable and meaningful code.
Some of the valid identifiers are: shyam
, _max
, j_47
, name10
.
And invalid identifiers are: 4xyz
, x-ray
, abc 2
.
RULES FOR NAMING IDENTIFIERS:
- Identifiers are case sensitive because C++ is case sensitive i.e., know the difference between uppercase letters and lowercase letters and where to use them
- You cannot start the name of an identifier with a digit/number. An identifier can be started with a letter ‘A’ to ‘Z’ or ‘a’ to ‘z’ or from underscore (_) which is followed by zero or more letters, underscores and digits (0 to 9).
- Other special characters such as $, %, and @ are not allowed.
- You cannot use keywords as identifier.
Watch - Identifiers in C++ (11:20 minutes)
Watch the following video. It discusses the concept of identifiers in C++ and some rules and conventions for using identifiers.
What are the Differences between Keywords and Identifiers?
In the following table the differences between keywords and identifiers are listed:
Keywords | Identifiers |
---|---|
Predefined/reserved words | The values used to define different programming items like a variable, integer, structure or union |
Always start with a lowercase letter | Can start with either lowercase or uppercase letters |
Contains only alphabetical characters | Can consist of alphabetical characters, numbers, and underscore |
No special characters or punctuation marks are used in keywords or identifiers, except underscore for identifiers.
A variable is a named location in memory that is used to hold a value that may be modified by the program. Each variable in C++ has specific characteristics that determine the size and layout of the variable’s memory, the range of values that can be stored within that memory, and the set of operations that can be applied to the variable.
The name of a variable can be composed of letters, digits, and the underscore character. It must begin with either a letter or an underscore. C++ is case sensitive therefore upper and lowercase letters are distinct. All variables must be declared before they can be used.
- The general form of a declaration is
type variable_list;
type
must be a valid C++ data type (including char
, w_char
, int
, float
, double
, bool
or any user-defined object), and variable_list
may consist of one or more identifier names separated by commas.
Some valid declarations are shown here:
Examples of variable declarations:
int i, j;
short int si;
unsigned int ui;
double balance, profit, loss;
Variable Rules
When you write variables you need to adhere to the following rules to avoid errors in your code.
- Variable names must begin with a letter or underscore (_) or dollar sign ($);
- Variable names are case sensitive.
- No special characters can be used except “_” and “$” sign;
- No blank spaces
- C++ keywords cannot be used as variable names.
Watch - Variables in C++ (13:45 minutes)
In this video the presenter passionately talks about variables and demonstrates the use of variables in coding.
Constants
Constants are variables or values in C++ that are fixed. They cannot be modified once they are defined.
They are useful for setting parameters in your code, such as setting the size of the screen, or the length of a memory buffer.
Constants can be set by either using the preprocessor defined directive #define
or implemented as regular variable using the const
prefix.
The term constant is often used interchangeably with the term literal. Technically the term constant refers to expression, and literal is the value of the constant. Check out what Geeksforgeeks has to say about Constants, as well as some examples of using constants in code.
Operators
Operators are the symbols used by the compiler to perform mathematical operations. There are various types of operators in C++, each perform specific functions.
The different types of operators and their functions/purpose are listed below:
- Arithmetic Operators are used to perform mathematical operations. There are two types of arithmetic operators – Unary and Binary. Unary operators work on single operators (
++
,--
), and binary operators work with two operands (+
,-
,*
,/
) - Relational or Comparison Operators are used to compare the values of two operands. For example, is the value of one operand greater than the other?
- Logical Operators are used for combining two or more conditions/constraints or to complement the evaluation of the original condition in consideration. The result of the operation of a logical operator is a Boolean value either
true
orfalse
. - Assignment Operators are used to assign values to another according to specific conditions.
For a deeper consideration of operators, the range of operators and their associated symbols, along with examples of the syntax of writing operators check out the following links:
Expressions and statements work together.
In C++ a statement is a unit of code, particularly a line of code. They are the instructions given to the computer to perform any kind of action. These actions could be data movements, decisions or repeating actions for example. Statements always end with a semi-colon.
C++ statements are typically executed sequentially, except when an expression statement, a selection statement, an iteration statement, or a jump statement specifically modifies that sequence.
The following statements are included in C++:
- Labeled Statements
- Expression Statements
- Compound Statements
- Selection Statements
- Iteration Statements
- Declaration Statements
- Try-throw-catch Statements
C++ expressions are ordered collections of operators and operands that specify any computations within the program. They are anything that returns a value whether or not the value is used. An expression may be part of a statement, or it may be an entire statement. An expression can include operators and parentheses, and operands can be constant or variable.
The following list shows the types of expressions that exist in C++:
- Special assignment expressions
- Constant expressions
- Integral expressions
- Float expressions
- Pointer expressions
- Relational expressions
- Logical expressions
- Bitwise expressions
Expressions can be combined where appropriate and are called Compound Expressions. For further information on expressions, with examples of code click on the following links:
In this section you will be introduced to the different ways in which you can code decision making processes. This is achieved through the use of if statements and switch statements.
if Statements
If statements are used for decision making in C++. Consider the example assigning assessment marks to a particular grade:
- If the assessment mark is greater than 90 percent, assign grade A;
- if the mark is above 75 percent, assign grade B; and so on
The if statement allows you to control if a program enters a section of code or not based on whether a given condition is true or false. One of the important functions of the if statement is that it allows the program to select an action based upon the user's input. For example, by using an if statement to check a user-entered password, your program can decide whether a user is allowed access to the program.
There are 3 forms of the if statements in C++:
- if statements
- if … else statements
- if … else if …. else statement
For detailed information about if statements, the syntax of the statements, with examples of where and how they are used click on the following links:
- W3Schools (n.d) C++ if Statements
- Alex Allain@Cprogramming (2019) If statements in C
- Programiz (n.d) C++ if, if...else and Nested if...else
The presenter of the following two videos discusses if statements very clearly and demonstrates the writing of if statement code to ‘solve’ several problems.
Switch Statements
In C++ a switch statement is a multiway branch statement that provides a way to organise the flow of execution to parts of code based on the value of the expression. In other words, a switch statement
- evaluates an expression,
- tests it and compares it against the several cases written in the code.
- When a match with any case is found, the control will enter that case and start executing the statements written in that case until a break statement has been found.
- When a break statement appears, the switch statement terminates, and the program control exits.
Watch - Programming tutorial for beginners in c++ Switch statements (9:29 minutes)
For an overview of the use of switch statements in programming with C++ watch the short video posted below:
watch - C++ for beginners (2020) Switch/case statement (25:35 minutes)
In this fabulous video you will learn to build a calculator application using switch-case statements. A great way to learn programming!
If you wanted a particular message to appear 20 times, then instead of having to write the print statement 20 times, a Loop can be used. Using Loops is a way to achieve efficiency and sophistication in your programming.
Loop statements (also known as iterative statements) in C++ allow a set of instructions to be executed repeatedly until a certain condition is reached. The type of Loop you use is dependent on whether the condition is predefined or open-ended.
C++ supports several Loop types, and each has its own syntax, advantages, and usage.
For Loop
This is an entry-controlled Loop, meaning that the condition specified by you is verified before entering the loop block. It is a repetition control structure. The Loop written by you is run a specified number of times.
While Loop
The While Loop is also an entry-controlled Loop, where you verify the condition specified by you, before running the Loop. The difference being that you would use For Loops when you know the number of times the body of the Loop needs to run, whereas you would use While Loops in circumstances when you do not know beforehand the precise number of times the body of the Loop needs to run. The execution of the Loop is terminated based on the test condition.
Do While Loop
Do While Loop is an exit-controlled loop, meaning the test condition is verified after the execution of the Loop, at the end of the body of the Loop. The body executes at least once, regardless of the result of the test condition, whether it is true or false. This is the difference between the While Loop and the Do While Loop. In the While Loop, the condition is tested beforehand, whereas in the Do While Loop the condition is verified at the finish of body of the Loop.
Watch - Loops in C++ (for loops, while loops) (12:20 minutes)
In the video below the presenter discusses the role and function of For loops and While Loops and gives some examples of where the Loops can be used in programming.
Watch - C++for beginners- Do while Loop: Difference between while and do while (15:05 minutes)
Have you ever forgot your PIN, and locked yourself out of your account or phone? That is an example of where Do While Loops are useful. The following video explores Do While Loop and uses to the example of the ‘forgotten PIN’ to explain the concept.
Whilst you are able to create a useful, functional C++ programs solely out of statements that you have written, it is not typical because there no keywords that can perform things such as I/O operations, high-level mathematical computations, or character handling. Therefore, most programs include calls to various functions that are contained in what is called the standard library.
All C++ compilers come with a standard library of functions that perform most commonly needed tasks. Standard C++ specifies a minimal set of functions that will be supported by all compilers. However, your compiler will probably contain many other functions. For example, the standard library does not define any graphics functions, but your compiler will probably include some.
Check out the following links to find out more about the C++ Standard Library:
An array is simply a collection of variables of the same data type that are referenced by a common name. In other words, when elements of linear structures are represented in the memory by means of contiguous memory locations, these linear structures are called arrays.
An array stores a list of finite number (n) of homogeneous data elements (i.e., data elements of the same type). The number n is called length or size or range of an array. Specific elements in an array are accessed by an index
There are two types of arrays in C++. They are:
Single-Dimension Arrays
Single-Dimension Arrays are essentially lists of information of the same type that are stored in contiguous memory locations in index order.
Multi – Dimension Arrays
Multi-Dimensional Arrays are stored in a row-column matrix, where the first index indicates the row and the second indicates the column. These types of arrays will be considered in greater depth in the module CS103 Integrated Studio I.
Online Resources:
There are many websites that provide opportunities to learn about programming languages, and in particular programming in C++
The following sites are recommended for you as sources for further learning on C++ programming and opportunities put your skills in practice. These sites provide free content, as well as additional content behind a paywall (which you are under no obligation to purchase).
Microsoft also provides a site with comprehensive information on C++, as well as using Microsoft Visual Studio.
Video Tutorials:
Youtube can be a great source of content, and when it comes to C++ programming there are many enthusiastic presenters who wish to share their knowledge with you. You have already watched some of these videos as you have worked your way through this module. However here you will find links to a number of playlists that you will find very useful as you complete your assessments and develop your skills as a programmer:
CodeBeauty is the channel of Saldina Nurak a software engineer. She started her channel during the COVID-19 pandemic, and sees it “as a way of giving my contribution, sharing the knowledge, making programming tutorials, answering questions related to this industry, and making fun developer life videos.” In her videos she discusses programming concepts, presents real life situations or problems, and takes you through the process of writing the code to ‘solve’ the scenario. There is also another relevant playlist on Saldina's channel.
Bookmark her channel as we are sure you will revisit it again to continue learning about C++ and other aspects of programming
TheCherno C++ Playlist
The Cherno is a young Australian programmer who has nearly 100 videos in his C++ playlist. The videos take you through setting up C++, understanding the compiler and linker, and covers all the topics introduced in this module, and also in future modules. His interest lies in game development, and he has several game programming playlists on his channel. He has nearly 450K subscribers - will you be another?
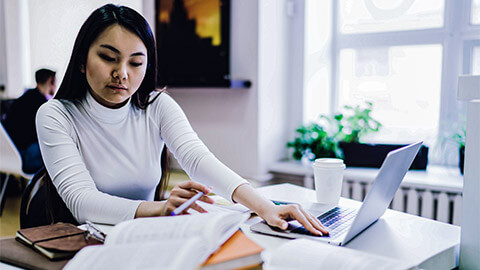
Written Resources:
The following written texts on C++ are recommended. You may be able to borrow them from your local library, or request an interloan from the librarian:
- Stroustrup, Bjarne (2013). The C++ Programming Language (Fourth Edition). USA: Pearson Education
- Schildt, Herbert (2033). C++: The Complete Reference (Fourth Edition). New York: McGraw Hill
Programming Exercises:
In this section you will be able to find some coding exercises to hone your programming skills. The exercise sheets can be downloaded and worked through at your own pace as your knowledge and coding skills develop.
- CS102 Practical Coding Exercises I
- CS102 Practical Coding Exercises II
- CS102 Practical Coding Exercises III
INSTRUCTIONS FOR COMPLETING & SUBMITTING PRACTICAL EXERCISES:
- Open Visual Studio and complete the practical coding exercises.
- Once you have completed your coding you will need to upload your complete coding in your Github repository. Instructions for setting up Git and Github are provided below.
- Contact your online tutor if you have any problems or issues with submitting your practical exercises.
Installing Git and Setting Up Your Github Repository
What is Github:
Github is a cloud-based code hosting platform that allows you to store code, share and collaborate with others, and provides version control.
A GitHub repository is used to store your development projects. It can contain folders and any type of file (HTML, CSS, JavaScript, Documents, Data, Images). A GitHub repository should also include a licence file and a README file about the project. It can also be used to store ideas, or any resources that you want to share with others.
Setting up your Github account
Before you start you will need to set up a Github account (if you don’t have one already). Head to Github and click the ‘Signup’ button. This will take you through the setup process.
Now you are all set up, but in order to upload your coding to Github you will need to install another application called Git.
Installing and setting up Git
Git can be installed on the most common operating systems like Windows, Mac, and Linux. In fact, Git comes installed by default on most Macs. To see if you already have Git installed, open up your terminal application.
- If you're on a Mac, look for a command prompt application called "Terminal".
- If you're on a Windows machine, open the windows command prompt
Once you've opened your terminal application, type git version. The output will either tell you which version of Git is installed, or it will alert you that git is an unknown command. If it's an unknown command, you will need to install Git. Click here for instructions on installing Git on your computer.
Follow these download and installation instructions.
Uploading your code from Git to Github
Push and Pull are the processes for uploading your code files to Github (push) and downloading files to your local computer (pull)
To place your code in the Github repository you will need to follow the process for uploading your files using the command prompt (this is the preferred way to upload). Follow these upload instructions
watch - how to push git repo from windows 10 CMD (9:32 minutes)
You can also watch the following video about the process of uploading your code from Github.
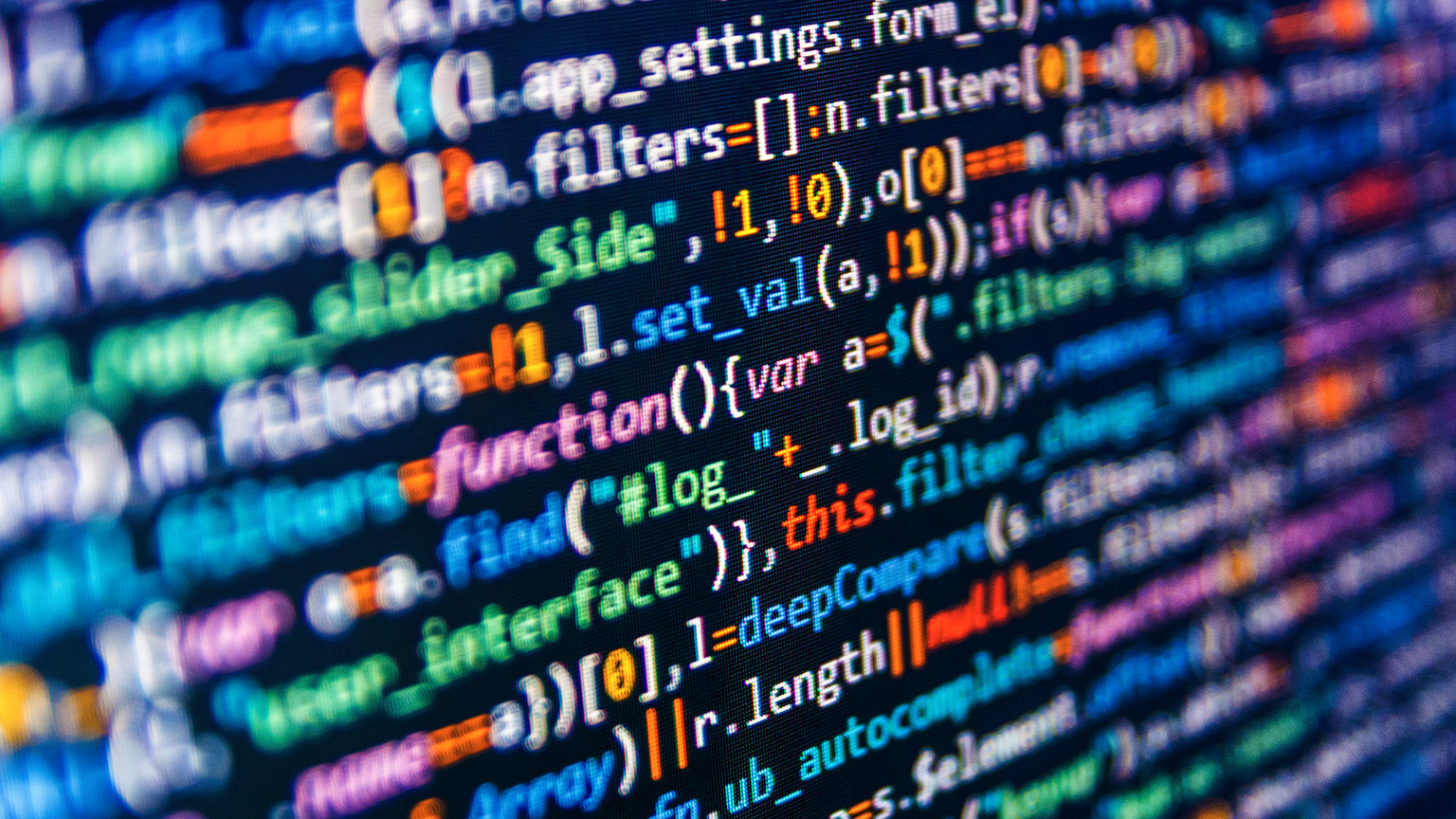