In this topic, we will cover:
- JavaScript overview
- Variables
- Operators
- Conditional statements
- Loops
- Input, processing and output
- Functions
- Arrays
- Overview of object programming
- Coding standards
- Yoobee best practices
- GitHub.
What is JavaScript?
One of the most important web development technologies, JavaScript (JS) is a fun and universal programming language. It can be used on both the front-end and back-end platforms and allows you to make web pages interactive.
HTML and CSS provide structure and aesthetics to web pages, while JavaScript adds interactive components that keep users engaged.
JavaScript is a set of instructions that guide the computer to perform a specific task and that could be anything from displaying text to changing an image, to inquiring about the user's preferences or information. The Search box on Amazon, a news recap video posted on Stuff, and refreshing your Twitter feed are all examples of JavaScript that you may encounter daily.
In most cases, the instructions, also known as code, are processed from the top line down. Simply put, the computer examines the code you've written, determines the action you want it to take, and then executes it. Running or executing code refers to the process of processing it.1
JavaScript and the web
'JavaScript code runs inside a web page loaded into a browser. All you need to create these web pages is a text editor (such as Windows Notepad) and a web browser to view your pages on.
'Browsers (such as Chrome, Safari, Firefox, and Edge) come equipped with JavaScript interpreters (also known as JavaScript engines).
'Today, JavaScript support is more unified than ever across browsers, but developers may still need to cope with older, and non‐standard, JavaScript implementations. The ECMAScript standard controls aspects of the language and helps ensure that different versions of JavaScript are compatible.'2
What can JavaScript do?
‘JavaScript is primarily used to interact with users. This “interaction with users” can be broken down into two categories:
- User input validation
- Progressive enhancement.'
JavaScript was created for validating form input.
For example, you may have seen that when you submit an invalid email address into some forms, the form prompts you to 'Please enter a valid email address'. User-based JavaScript is commonly used for this type of quick validation.
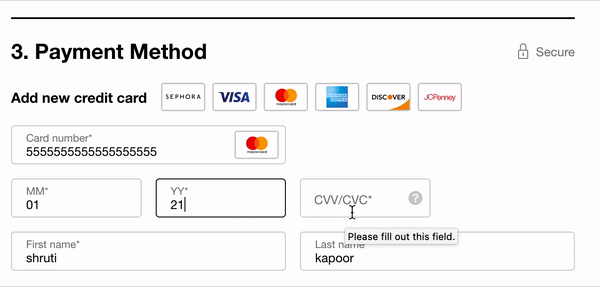
'Progressive Enhancement' allows anyone with any browser or internet connection to access a webpage's fundamental content and functionality, while also offering an upgraded version of the page to those with more advanced browser software or more bandwidth.
Here are a few examples of how JavaScript is used:
- Adding interactive behaviour for webpages
- Show or hide more information with the click of a button
- Change the colour of a button when the mouse hovers over it
- Slide through a carousel of images on the home pages
- Zooming in or zooming out on an image
- Displaying a count-down on a website
- Playing audio and video in a web page
- Displaying animations
- Using a drop-down menu
- Creating web and mobile apps
- For developing web and mobile apps, developers can use a variety of JavaScript frameworks
- JavaScript frameworks are collections of JavaScript code libraries
- Gives developers pre-written code used for common programming features
- Build web servers and develop server applications
- JavaScript is used to create simple web servers
- Node.js is used to develop the back-end infrastructure
- Game development
- JavaScript is used to create browser games
Tools for JavaScript
The great news is that learning JavaScript requires no expensive software purchases. You can learn JavaScript for free on any PC or Mac.
This section discusses what tools are available and how to obtain them.
Development tools
All that you need to start writing JavaScript for web applications is a simple text editor, such as Notepad for Windows, or TextEdit for Mac OS X. You can also use one of the many advanced text editors that provide line, numbering, colour coding, search and replace, and so on. Here are just a few:
- Notepad++ (Windows)
- VSCode (cross-platform)
- Chrome DevTools (Windows)
- Sublime Text (cross‐platform)
- Codepen (cross-platform)
Check out the accordion for some more detail on these tools.
Notepad++ is a multi-language source code editor and Notepad replacement. And it is free! Notepad++ is written in C++, resulting in faster execution and smaller programme size.
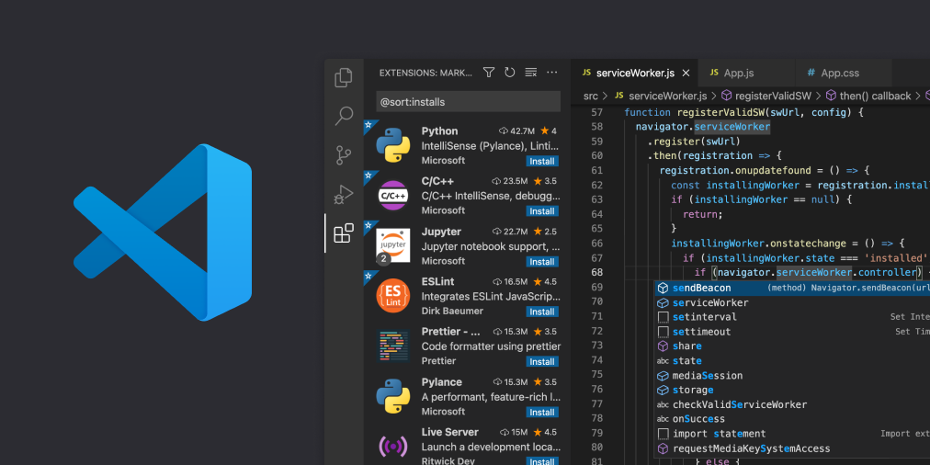
Visual Studio Code (VSCode) is an improved code editor for developing and debugging modern web and cloud applications. VSCode is a source-code editor made for both Windows and macOS.
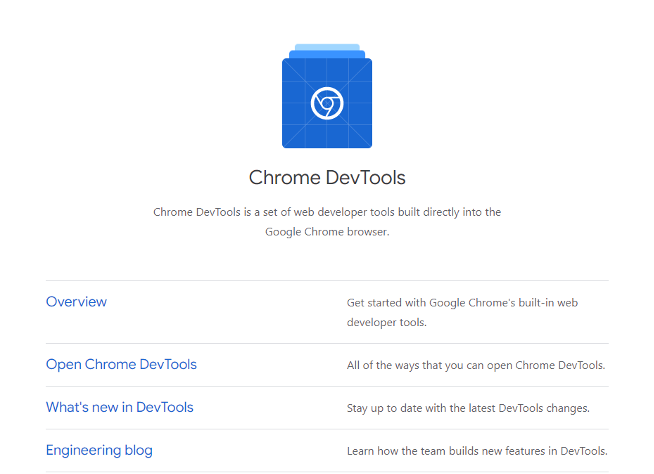
Google’s built-in Chrome developer tools let you edit your HTML and CSS in real-time, or debug your JavaScript, all while viewing a thorough performance analysis of your website.
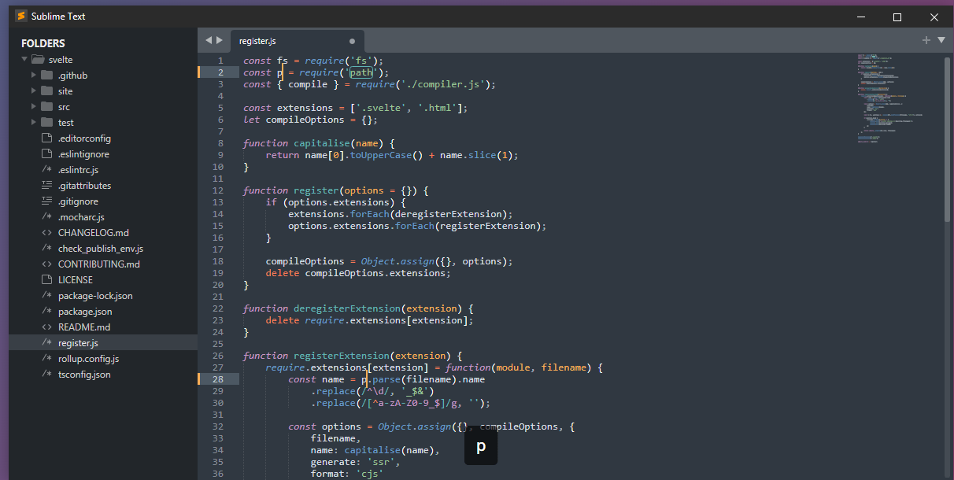
Sublime's popularity lies in its extensive set of keyboard shortcuts, which include the ability to perform simultaneous editing and easy access to files, symbols and lines.
Codepen
Codepen is a simple method to show not only the code behind a feature you have created, but also how it appears for users.
If you prefer an HTML editor, you will need one that allows you to edit the HTML source code. This is where you add your JavaScript. There are many applications on the market specifically geared towards developing web-based applications, such as VSCode.
When it comes to learning the basics, it is best to hand code. This gives you experience and helps you understand the fundamentals of the language before undertaking more advanced logic that is beyond a tool’s capability. Once you have the basics down, you can use tools as timesavers and spend your time on more complex and interesting code.2
Console.log()
The console.log()
function is a simple JavaScript function for displaying or printing messages to the debugger's console. Any previously specified variable (including arrays, objects and so on) or any string can be used as the message. During the development phase, console.log()
is frequently used for testing purposes.
Web browsers
In addition to software that lets you edit webpages, you will also need a browser to view your webpages. It is best to develop your JavaScript while testing the browsers you expect visitors to use to access your website. Some major browsers include:
A final point to note is how to open the JavaScript console in your browsers. Check out the following video on how to open JavaScript in Google Chrome.
Making JavaScript work with <script>
Inserting JavaScript into a webpage is much like inserting any other HTML content. You use tags to mark the start and end of your script code. The element you use to do this is </script>
.
This tells the browser that the following chunk of text, bounded by the closing tag, is not HTML to be displayed, but rather script code to be processed. The chunk of code surrounded by <script></script>
tags is called a script block. Here’s an example:
<script type="text/javascript"> Your JavaScript goes here... </script>
Basically, when the browser spots <script>
tags, instead of trying to display the contained text to the user, it uses the browser’s JavaScript engine to run the code’s instructions. Of course, the code might give instructions about changes to the way the page is displayed or what is shown in the page, but the text of the code itself is never shown to the user.
"The <script>
tag can be placed in the <head>
section of your HTML or in the <body>
section, depending on when you want the JavaScript to load.
Generally, JavaScript code can go inside of the document <head>
section in order to keep them contained and out of the main content of your HTML document. However, if your script needs to run at a certain point within a page’s layout—like when using document.write
to generate content—you should put it at the point where it should be called, usually within the <script>
section."3
For example:
Linking to an external JavaScript file
You can alternatively create a separate file with the (.js) extension to store the code and then use the src attribute of the <script>
element to include it into your HTML content. When you wish to use the same code in different HTML documents, it comes in handy. It also saves you from having to write the same code over and over again, making webpage maintenance easier.
'Another advantage of using external files is that the browser will cache them, much as it does with images shared between pages. If your files are large, this could save download time and also reduce bandwidth usage.'2
For example, imagine you have created a file called MyCommonFunctions.js to which you want to link, and the file is in the same directory as your web page. The <script>
element would look like this:
<script src="MyCommonFunction.js"></script>
The web browser will read this code and include the file contents as part of your webpage. When linking to external files, you must not put any code within the opening and closing <script>
tags. For example, the following would be invalid:
<script src="MyCommonFunction.js"> var myVariable; if (myVariable == 1) { // Do something } </script>
'It is important to note that an opening <script>
tag must be accompanied by a closing </script>
tag. You cannot use the self‐closing syntax found in XML. Therefore, the following is invalid:
<script src="MyCommonFunction.js" />
Generally, you use the <script>
element to load local files (those on the same computer as the web page itself). However, you can load external files from a web server by specifying the web address of the file. For example, if your file was called MyCommonFunctions.js and was loaded on a web server with the domain name www.mysite.com, the </script>
element would look like this:
<script src="https://www.mysite.com/MyCommonFunctions.js"></script>
Linking to an external file is common when incorporating well‐known JavaScript libraries into a web page. The servers hosting these libraries are referred to as Content Delivery Networks, or CDNs. CDNs are relatively safe, but beware of linking to external files if they are controlled by other people. It would give those people the ability to control and change your web page.'2
Watch this next video to learn more about the use of external files and JavaScript.
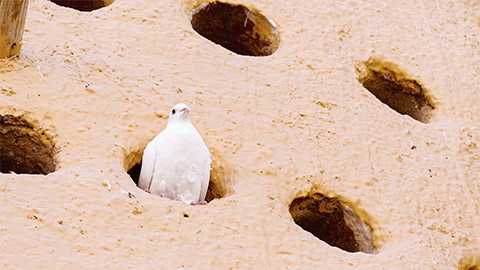
'Think of computer memory as a giant wall of mailboxes or pigeon holes. Each box or hole has a label on it--the variable. A JavaScript variable is simply a name of storage location. There are two types of variables in JavaScript: local variable and global variable.'4
- Local Variable: When you use JavaScript, local variables are variables that are defined within functions. They have local scope, which means that they can only be used within the functions that define them.
- Global Variable: In contrast, global variables are variables that are defined outside of functions. These variables have global scope, so they can be used by any function without passing them to the function as parameters.
'There are some rules while declaring a JavaScript variable (also known as identifiers).
- Name must start with a letter (
A–Z
,a–z
), underscore(_
), or dollar($
) sign. - After first letter we can use digits (0 to 9), for example value1.
- JavaScript variables are case sensitive, for example
x
andX
are different variables. - JavaScript variables are containers for storing data values.'4
In this example, x
, y
, and z
, are variables:
var x = 5; var y = 6; var z = x + y; // => 11
z
will output 11
.
Defining variables
The keyword var
tells JavaScript to create a variable. A variable can hold one value and one value only. If we put a new value in, any previous value is discarded.
Variables do nothing but provide a place for a value to live between statements. Everything in JavaScript works with the value stored by the variable, not the variable itself. You could say that JavaScript code is interested in the peanut butter stored in the jar, but not really concerned about the jar.
Variable names
Your variable should have a name that accurately identifies it. Your code is easier to understand when you use suitable variable names. It is critical to name variables correctly!
The rules for naming variables in JavaScript are listed here.
- Names for things in JavaScript, such as variables, should be formed from the 26 upper and lowercase letters (
A–Z
,a–z
), the 10 digits (0–9
), and underscore (_
). - Variables and functions should start with a lowercase letter.
- Variables in JavaScript cannot start with a digit.
- Variables and functions cannot have spaces in their names.
There are tons of reserved keywords in JavaScript that you cannot use as a name--var is one of them.
Check Mozilla Developer Network's Lexical Grammar page for the complete list of words you can't use. Notable reserved words include class and super, but you can use className and superMan.
Naming conventions
By convention, JavaScript programmers use so-called 'camelCase' for their variable names. The first word is lowercase, and subsequent words have their initial letter capitalised. We will use camelCase.
You may also see variables named with 'snake_case', where the whole name is lowercase, and words are separated with underscores.
Code should always look like it has been written consistently by a single person, even when written by a team. Use camelCase or snake_case, but remain consistent. When editing other people's code, obey the conventions you see being followed: When in Rome, do as the Romans do.
Names should be meaningful, so avoid shortenings, abbreviations and acronyms. It is better to be clear than clever:
phNum
should bephoneNumber
addr2
should beaddress2
clr
should becolor
.
It is best to use American English spelling where it matches HTML or CSS--most notably the word 'color' and not the British 'colour'.
That said, some are universally accepted, such as id
for identifier and i
for iteration.
Initial values - undefined
When you initialise a variable, the variable is considered to have the value 'undefined'. This is a value all variables get before any other value is assigned to them.
var name; console.log(name); // => undefined
The above coding output to the console undefined.
Assigning values to variables
The =
is called the 'assignment operator'. Try not to think of this as 'equals' but 'is assigned the value'.
var username = "Earl"; var age = 27;
Here, username
is assigned the value "Earl"
.
We can also assign a value to a variable when it is defined.
username = "Earl"; age = 27; canDrive = true;
Using variables as values
console.log(username); // => "Earl" console.log(age); // => 27 console.log(canDrive); // => true
Here the method console.log()
is being passed the username
variable. What is actually taking place here is the value inside username
is being evaluated to its value before being passed into console.log()
:
console.log("Earl"); console.log(27); console.log(true);
If the value obtained from the variable is changed, the value still in the variable is left unchanged.
Changing a variable's value
var coffeesConsumed = 2; console.log(coffeesConsumed); // => 2 coffeesConsumed = 3; console.log(coffeesConsumed); // => 3
We can replace a variable's value by assigning a new one into it. The old value is discarded permanently, unless of course, you copy it into another variable:
var coffeesConsumed = 2; console.log(coffeesConsumed); // => 2 var coffeesPreviouslyConsumed = coffeesConsumed; coffeesConsumed = 99; console.log(coffeesConsumed); // => 99 console.log(coffeesPreviouslyConsumed); // => 2
Reference error: variable is not defined
When you attempt to use a variable that has not been defined, you may get an error message:
console.log(chunkyBacon); // => Uncaught ReferenceError: chunkyBacon is not defined.
To resolve this, make sure you define the variable in a statement before the line where you use the variable. If you already have, check your spelling as one of them may be spelled incorrectly or inconsistently.
Defining multiple variables at once
You can define multiple variables at once, separating them with a comma (,
).
var john, paul, george, ringo;
Best practice: Only use the , with var when defining multiple variables that do not have initial values.
var coach, captain, teamName; var waterboy = "Bobby"; var score = 2; var winning = true;
What can we do with values? Make new values from old ones! These are called 'operations'.
We use 'operators' to signify that we wish to perform these operations.
Operations that are performed on 1, 2 or 3 other values, are called 'operands'.
Assignment operator
=
value assignment – 'is set to' The assignment operator assigns a value to a variable. For example:
If a = 10
and y = a
then y = 10
.
Numbers and arithmetic
+
addition-
subtraction*
multiplication/
division%
remainder.
Addition is performed with the +
operator. For example:
y = 5 + 5
gives y = 10
.
Division operator
/
divides like a calculator divides, so 10 / 4
is 2.5
, and 12 / 4
is 3
.
Remainder operator
%
is the remainder operator. It returns the remainder of an integer division.14 % 4
is 2
. Because an integer division is happening, 14 ÷ 4 is 3 remainder 2.
Strings and concatenation
+
concatenation "to link things together end to end".
If either operand around the + operator is a string value, + will not do addition, but instead will do concatenation and return the two values joined together as a string. If one operand is a string and the other is not, the other will be type coerced into a string. Type coercion is talked about below.
10 + "10"
is"1010"
. The number is coerced into a string, and a new string is created"hello" + "world"
is"helloworld"
. Note how no space is placed between the 2 words. JavaScript has no awareness of English or other written languages."hello" + "world"
is"hello world"
. A space follows the word "hello" and so is included in the concatenation.var hello = "hello"; var world = "world"; console.log(hello + " " + world)
In the parentheses "hello" + " " + "world"
is being computed, which is "hello world"
.
Boolean values and logical operations
In JavaScript, there are 4 logical operators: ||
(or), &&
(and), and !
(not), ??
(coalescing of nulls).
We will go through the first 3. Even though they are called 'logical', they can be used to any sort of value, not just boolean. Their outcome can be of any kind.
Let us look at the specifics.
&&
logical (and)
It checks whether 2 operands are non-zero (0
,false
,undefined
,null
or""
are considered as zero), if yes then return1
otherwise0
.
Example:y = 5
andx = 6
y && x
istrue
.||
logical (or)
It checks whether any 1 of the 2 operands is non-zero (0
,false
,undefined
,null
or""
are considered as zero). Thus||
returnstrue
if either operand istrue
and if both arefalse
it returnsfalse
.
Example:y = 5
andx = 6
y || x
istrue
.!
logical NOT (unary)
It reverses the boolean result of the operand (or condition).
Example:y = 5
andx = 0
!(y || x)
isfalse
.
In Boolean logic, we can combine 2 Boolean values into 1 using the Boolean operators.
With &&
the logical and operator, both operands must be true
to get a true
result.
false && false
isfalse
true && false
isfalse
false && true
isfalse
true && true
istrue
With ||
the logical or operator, 1 or more of the operands must be true
to get a true
result.
false || false
isfalse
true || false
istrue
false || true
isfalse
true || true
istrue
With !
the logical not operator, it only takes 1 operand, and the value of that operand is 'flipped':
!true
isfalse
!false
istrue
As an aside, the &
symbol is called an 'ampersand' and the |
is called the 'vertical bar' (or in a command-line interface a 'pipe').
If an operand for a Boolean operator is not a Boolean value, it will be type coerced into a Boolean value.
Watch this Logical OR operator video to learn more about JavaScript.
Formatting
Because good code is easily read, always put a space on both sides of an operator. Good formatting:
1 + 1
"His name is " + " Robert Paulson."
!true
isWeekday && sunny
(!forgotten || overAged)
Order of operations
Some operators take precedence over others and must be calculated or 'evaluated' first. Take for example:
9 + 9 * 9
Is this 9 plus 9 equals 18, 18 times 9 equals 162? (the =>
are here to show us the result):
9 + 9 // => 18 18 * 9 // => 162
Or is it 9 times 9 equals 81, 9 plus 81 equals 90?
9 * 9 // => 81 9 + 81 // => 90
In programming, this is clearly defined. Each programming language has its own order of operations.
Mathematics has a standard 'order of operations', often memorised as BEDMAS:
- Parentheses (brackets)
- Exponents
- Division
- Multiplication
- Addition
- Subtraction.
In programming, every operator takes a place in the language's order of operations hierarchy. All languages follow the BEDMAS order, but there are more operators. Most languages share the same order but can differ slightly.
Check out JavaScript's Order of Operations.
The = assignment operator is very low in the order of operations, and thus all other calculations happen before a value is assigned to a variable.
var result = 9 + 9 * 9; console.log(result); // => 90
This code will write to the console the number 90
.
Associativity
Multiplication and division actually share the same level in the order of operations, as do addition and subtraction. Other operators are also grouped in levels.
Depending on the operator, the left-most or the right-most operator might be evaluated first. This is called associativity. For example:
- the assignment operators have right-to-left associativity
- the arithmetic operators have left-to-right.
Parentheses
()
- Grouping operators
Because the order of operations is hard to memorise, it's best to be explicit with what order of operations you (the programmer) intend by using parentheses to group operands with the desired operators.
9 + 9 * 9 // => 90
– the order of operations will be applied.9 + (9 * 9) // => 90
– here the parentheses are optional, but document the intent.(9 + 9) * 9 // => 162
– parentheses are required to override default order of operations.
Documenting values with variables
Sometimes when writing a calculation, you may want to multiply one number with another.
var quantity = 2; var total = quantity * 9; // => 18
Bad practice: The 9 here is referred to as a 'magic number'–it is unclear what it actually represents. Maybe you figured out that 9 is the unit price, but did you have to think about it?
Best practice: Document what a value is by putting it in a variable. We will also make sure that it is clear we are calculating a price and not a unit total. This makes for very readable code:
var unitPrice = 9; var quantity = 2; var totalPrice = quantity * unitPrice; // => 18
Best practice: By putting numbers we use multiple times in a variable, we can keep that value in one place. This builds maintainable code. If the unitPrice changes in the future, we only need to change it in one place.
Variables that are not supposed to be changed while the program is running are called 'constants'. The value in the variable should stay the same.
Some languages have syntax to declare the variable as read-only--JavaScript does not.
If you want a variable to be treated as a constant, type it in ALLCAPS as a sign to yourself and others that this variable should not be modified by the program. Using this formatting is not always necessary, but it is an established pattern you will see in others' published code.
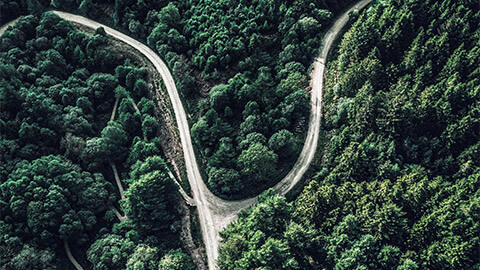
A 'condition' is like a calculation, but returns a Boolean true or false.
Conditions are used by programming languages to check, test or evaluate the state of a variable's value. Soon, we will use them to control what parts of our program will run depending on the value of the variables.
This video explains conditional statements in JS (JavsaScript).
‘The conditional statements included in the JavaScript code assist with decision making, based on certain conditions. The condition specified in the conditional statement can either be true or false. The conditional statements execute the associated piece of code only if the condition is true. We have four types of conditional statements in JavaScript:
- An if statement executes a specified code segment if the given condition is
true
- An
else
statement executes the code associated with theelse
if the givenif
condition isfalse
- An
else if
statement specifies a new condition for testing when the first if condition isfalse
- A switch-case statement specifies multiple alternative code blocks for execution and executes these cases based on certain conditions.
The if conditional statement
The conditional if
statement specifies a JavaScript code for execution only if the given condition is true
.
Example:
if (age >= 18) { eligibility = "Votable age"; }
Explanation:
The above if statement checks if the variable age
is greater than or equal to 18
. If it is greater than or equal to 18
, it assigns "Votable age"
to the variable eligibility
. For age
values less than 18
, the condition fails and the code associated with the if statement will not be executed.
The else conditional statement
When the condition inside the if statement is false, the code associated with the else statement gets executed.
Example:
if (age >= 18) { eligibility = "Votable age"; } else { eligibility = "Not votable age"; }
Explanation:
If the value of the age
variable is >= 18
, the code associated with the if condition is executed. On the other hand, if the age
variable's value is >= 18
, the code associated with the else statement is executed and "Not votable age"
is assigned to the variable eligibility
.
The else-if conditional statement
When the first condition fails, the else-if allows the program to specify some new condition(s).
Example:
if (marks > 90 && marks <= 100) { grade = "A+"; } else if (marks > 80 && marks <= 90) { grade = "A"; } else if (marks > 70 && marks <= 80) { grade = "B"; } else if (marks > 60 && marks <= 70) { grade = "C"; } else if (marks > 50 && marks <= 60) { grade = "D"; } else { grade = "Fail"; }
Explanation:
In the above example, multiple else if statements are used.
- The first condition checks if the
marks
are between90
and100
(inclusive) and assigns"A+"
to thegrade
variable if the condition istrue
- If the first condition fails, the second condition checks if the
marks
are between80
and90
(inclusive) and assigns"A"
tograde
if the condition istrue
- If the second condition fails, the third condition checks if the
marks
are between70
and80
(inclusive) and assigns"B"
tograde
if the condition istrue
- If the third condition fails, the fourth condition checks if the
marks
are between60
and70
(inclusive) and assigns"C"
tograde
if the condition istrue
- If the fourth condition fails, the fifth condition checks if the
marks
are between50
and60
(inclusive) and assigns"D"
tograde
if the condition istrue
- If all the above conditions fail, the
else
statement is executed and thegrade
is assigned the value"Fail"
.
The switch case statement
The case
statement evaluates an expression, compares the result against case
values, and then executes the statement that matches the case
value.45
Example:
switch (expression) { case x: // Code block break; case y: // Code block break; default: // Code block };
Explanation:
- The
switch
expression is evaluated once - The value of the expression is compared with the values of each case
- If there is a match, the associated block of code is executed
- If there is no match, the default code block is executed.
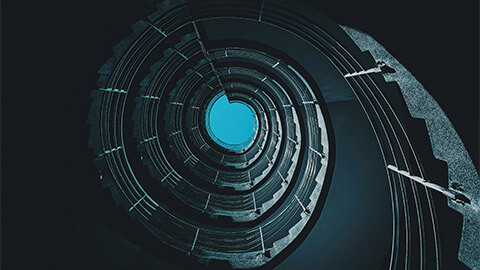
'Loops' are used in JavaScript to perform repeated tasks based on a condition. Conditions typically return true or false when analysed. A loop will continue running until the defined condition returns false.
The three types of loops are:
for
while
do...while
5
A trivial example might be we want to show a string five times:
var username = prompt("What's your name?"); console.log(username); console.log(username); console.log(username); console.log(username); console.log(username);
Here we are repeating code. If we need to change the variable, we need to change it five times. There is a better way.
Choosing the right loop can be challenging. The three types of loops will be discussed in the next section, but the for loop is the most frequently used and most efficient loop in terms of execution performance. Keep your attention on the for loop as it will be sufficient for most loop actions.
For
for (init, condition, final) statement for (var i = 0; i < 5; i++) { console.log(i); };
The for loop is the most fundamental form of iteration method in JavaScript. It consists of three parameters. The three optional parameters are followed by a code block in the for loop:
- The first parameter is the initialiser statement: the statement here will only be run once, before the first time through the loop. It is used to initialise the count variable.
- The second parameter is the condition. Just like the other loops, if this condition is true, it will run the loop block.
- The third parameter is the final statement: after running the loop block, the final statement will run, followed by checking the condition to see if it should loop again. This is used to increment or decrement the count variable.
The code above uses i as a variable. This convention is as old as the hills when programming languages could only have single-letter variables. i here stands for iteration, keeping count of how many times through the loop it has or has yet to go.
Be aware of inclusiveness when writing your loop's condition--if you want the loop to run exactly ten times, you could * Start at i at 1 and check for i < 11, or * Start at i at 0 and check for i < 10.
Hopefully, you can see why starting at 0 is important. After it is has been through once, i will be 1 and after ten times it will be 10.
while loop
while (condition) statement while (conditionIsTrue) { // Code block };
This is a loop. The statements in the block between do and while will be run once, after which the while condition will be evaluated. If true, the block will be run again, creating a loop. Each time through the loop is sometimes called an iteration.
var username = prompt("What's your name?"); var outputCount = 5; while (outputCount < 0) { console.log(username); outputCount--; // Subtract 1 from outputCount and keep the new value };
This code will ask for a string, and output that string five times.
Before it runs the loop block, it will check to see if outputCount is greater than zero, and if that condition is true. If true, it will run the loop block: output the string, and decrement outputCount by one. After each loop iteration, it checks the while condition again, and as long as the condition is true, it will continue to run the loop block.
do…while
The break statement terminates the loop and replaces the ended statement with the next statement in the programme.
You can break out of a loop early with break:
alert("Welcome to the clubhouse..."); while (true) { var password = prompt("...what's the password?"); if (password === "banana") { break; } alert("Nope, that's not it. View the source and try again."); } alert("You know the password? Awesome! Have a squishy, yellow day!");
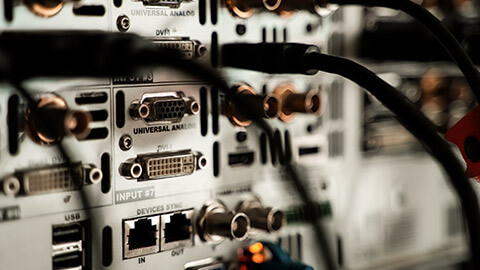
Every computer programme you write does 3 things:
- takes input
- performs something with or to the input
- tells the program's user how the input has changed.
This process is sometimes carried out by the programme using a technique known as 'bulk processing', in which all of the inputs are received, processed, and the results are then produced.
Watch this video for further explanation of input and output in JS.
Functions are like smaller programs within your larger program and are sometimes referred to as 'subroutines'. They wrap a set of statements that work together, usually to compute one piece of output.
Those functions usually work with some input given to them. Functions are essentially a variable that stores a function object.
Calling a function
We can invoke or execute a function by calling it, using the name of the function followed by a pair of parentheses. A function call looks like this:
alert()
When we call a function we can pass it values as input. These values are called parameters or arguments.
parseInt("123", 10);
Here the function parseInt is being called, passing it the string "123"
as the first parameter and the number 10
as the second parameter. Functions, when they are defined, can capture these parameter values in parameter variables.
Built-in functions
You have already used some of JavaScript's built-in functions:
alert("Hello, world!"); console.log("Hello devs"); Number("123.45"); parseFloat("321.98"); parseInt("123", 10);
All these functions take a value, in order to do something with that value. Some of these functions return useful values that can be used in our own programming. JavaScript has plenty more built-in functions for you to research and discover.
Some of the built-in functions you will find most useful at this stage follow.
Modal functions
alert("for your information"); var performDelete = confirm("Do you want to delete that?"); var username = prompt("What's your name?");
These 3 functions can be very useful when learning and debugging your code, but are considered bad practice for common use. The 3 functions are 'modal', meaning they pause the whole browser tab from doing anything: repainting, further JavaScript execution. The user cannot interact with the webpage until the modal is dismissed. Resources such as images and other files will continue to download, but the page will not be updated until the modal window is closed.
alert(message);
When the browser executes the alert()
function, the browser will display a little window with your message in it, and clicking the OK button, pressing Enter, or pressing Escape will dismiss it. If the website uses a lot of alert modals, your browser may ask you if you would like to ignore any future alerts.
alert() // Always returns undefined.
confirm(message);
When the browser executes the confirm()
function, the browser will display a little window with your message in it, along with 2 buttons: OK and Cancel.
confirm()
will return true or false depending on if OK or Cancel was clicked, respectively. You probably intend to capture this Boolean value in a variable for use later in your program.
prompt(message, [defaultValue]);
When the browser executes the prompt()
function, the browser will display a little window with your message in it, along with a text field and the 2 buttons: OK and Cancel. The text field will have focus and the user can enter a string as input.
If the second parameter defaultValue is provided, the text field will initially contain defaultValue. The text will be highlighted so it can be overwritten easily.
If the user clicks OK or presses Enter, prompt()
returns the string entered into the text field. prompt()
always returns a string, even when you enter a valid number. If you want a number, coerce the value prompt()
returns into a number before you treat it as one.
If the user clicks Cancel or presses Escape, prompt()
returns null. null is another value similar to undefined but represents the purposeful value of nothing. null is not a string, number, or Boolean.
Development console functions
The console object is a built-in object for your program to interact with the browser's developer console. Functions stored on an object are called 'methods'. There are many console methods, which you can research. The ones to know are:
console.log(message, [...]);
This outputs values to the developer console panel. Note that different browsers output different values differently. For example, Chrome and Firefox will colour strings and numbers differently, but Firefox shows quotes around strings while Chrome does not.
console.info(message, [...]); console.warn(message, [...]); console.error(message, [...]);
These functions share the same signature as console.log, but create different output. Depending on the browser, these functions might colour the output or put an icon next to the output. When writing complicated programs, this can help you differentiate your messages from one another.
console.info()
is for informative output, a 'heads up' that something expected happenedconsole.warning()
is for warnings: the program has detected something out of the ordinaryconsole.error()
is for errors: the program cannot do what it should because something is wrong.
Sometimes we need to store a list of values. Who's coming to dinner? What were the team's scores for the season? Arrays are ordered lists of data. Each item in the list is called an 'element', which we can access by the element's index.
var guests = ["Alice", "Charles", "Dave", "Bob"]; var scores = [10, 15, 12, 17, 3, 11, 23]; var bananas = [true, 0, "Today"]; // Yes! We have no bananas.
Once we have an array, we can loop over its elements the same way we loop over a string's characters. We can add and remove elements, change each element, and even sort the array any way we like.
In the example above, we use square brackets to denote an array literal. There are other ways to create arrays, but this is the most useful.
Learn some of the basics that apply to arrays from the following video.
Array elements
If we try to output an array, we'll see the contents of the array as a string:
console.log(guests); // => "Alice,Charles,Dave,Bob" console.log(guests); // => "10,15,12,17,3,11,23"
Note how the order of the elements is kept intact; no sorting takes place.
We can access elements of an array by index:
guests[0]; // => "Alice" guests[2]; // => "Dave"
We can find out how many elements are in an array with the length property:
scores.length; // => 7 for (var i = 0; i < scores.length; i++) { console.log(i + ": " + scores[i]); console.log(""); }
Creating an array from a string
We can create an array from a string with the split method:
String.split(delimiter); var guests = "Alice,Charles,Dave,Bob".split(","); var scores = "9 15 12 17 3 11 23".split(" "); guests.length; // => 4 scores.length; // => 7
Creating a string from an array
Usually, when an array is output, it will be coerced into a string:
alert(guests); // => "Alice,Charles,Dave,Bob" alert(scores); // => "9,15,12,17,3,11,23"
However, when using console.log or the like, the console panel will often show you the array in literal form.
We get more control over converting an array to a string using the join method:
Array.join(separator = ",");
It coerces each element's value to a string, and concatenates them, separating each element string with the separator, which defaults to ,.
scores.join(); // => "9,15,12,17,3,11,23" scores.join(" "); // => "9 15 12 17 3 11 23" guests.join(", "); // => "Alice, Charles, Dave, Bob" guests.join(" & "); // => "Alice & Charles & Dave & Bob"
Modifying an array
We can modify an element in an array:
guests[2] = "Steve"; guests.join(); // => "Alice,Charles,Steve,Bob" scores[0] = 8; scores.join(); // => "8,15,12,17,3,11,23"
We can add elements onto the end of an array with the push method:
scores.push(11); scores.join(); // => "9,15,12,17,3,11,23,11" scores.length; // => 8 guests.push("Elaine", "Frodo"); guests.join(); // => "Alice,Charles,Steve,Bob,Elaine,Frodo" guests.length; // => 6
JavaScript can also take elements off the end of an array with pop:
var lastGuest = guests.pop(); guests.join(); // => "Alice,Charles,Steve,Bob,Elaine" guests.length; // => 5 lastGuest; // => "Frodo"
We can also add and remove elements from the start of an array with unshift and shift:
guests.unshift("Gollum", "Samwise", "Merry", "Pippin"); guests.join(); // => "Gollum,Samwise,Merry,Pippin,Alice,Charles,Steve,Bob,Elaine" guests.length; // => 9 var unableToAttend = guests.shift(); unableToAttend; // => "Gollum"
Deleting and adding elements into an array is possible with splice:
Array.splice(start, deleteCount[, item1[, item2...]]); guests.splice(5, 2); guests.join(); // => "Samwise,Merry,Pippin,Alice,Charles,Elaine" guests.splice(3, 0, "Frodo"); guests.join(); // => "Samwise,Merry,Pippin,Frodo,Alice,Charles,Elaine" guests.splice(1, 0, "Bilbo", "Gandalf"); guests.join(); // => "Samwise,Bilbo,Gandalf,Merry,Pippin,Frodo,Alice,Charles,Elaine"
The modify functions
unshift shift │ ▲ │ │ ┌──▼──────┴─┐ 0│ Frodo │ ├───────────┤ 1│ Sam │ ───▶ ├───────────┤ splice 2│ Merry │ ◀─── ├───────────┤ 3│ Pippin │ └─▲───────┬─┘ │ │ │ ▼ push pop
Check out the Yoobee JavaScript Manual for more information on terms and definitions, or to save and use later.
'Real life objects, properties and methods
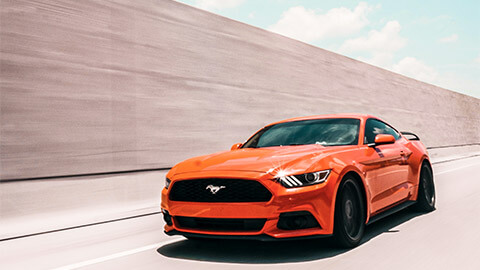
In real life, a car is an object. A car has properties like weight and colour, and methods like start and stop. All cars have the same properties, but the property values differ from car to car.'6
Properties | Methods |
---|---|
car.make = "Ford"; |
car.start(); |
car.model = "Mustang"; |
car.drive(); |
car.weight = "1,750 kg"; |
car.brake(); |
car.color = "Cherry Red"; |
car.stop(); |
Understanding objects
'JavaScript also supports objects. Like variables, objects can store data—but they can store two or more pieces of data at once.
The data stored in an object are called the properties of the object. For example, you could use objects to store information about people in an address book. The properties of each person object might include a name, an address, and a telephone number.
You should become intimately familiar with object-related syntax because you will see objects quite a lot, even if you do not build your own. You will definitely find yourself using built-in objects, and objects will very likely form a large part of any JavaScript libraries you import for use. JavaScript uses periods to separate object names and property names. For example, for a person object called Bob, the properties might include Bob.address and Bob.phone.
Objects can also include methods. A method is a function that works with an object’s data. For example, our person object for the address book might include a display() method to display the person’s information. In JavaScript terminology, the statement Bob.display() would display Bob’s details.
JavaScript supports three kinds of objects:
- Built-in objects—These objects are built into the JavaScript language. Other built-in objects include Array and String, Boolean and Number
- DOM (Document Object Model) objects—These objects represent various components of the browser and the current HTML document. For example, the alert() function you used earlier in this lesson is actually a method of the window object
- Custom objects—These are objects you create yourself. For example, you could create a person object.'7
Objects
'Objects' are collections of properties, with each property storing a value. If arrays are ordered lists, objects are more like dictionaries, only you cannot sort an object.
Everything in JavaScript is actually an object. Strings have properties such as length, charAt, and split. Numbers have properties like toFixed, and even Booleans have toString.
Some of these properties contain simple values: strings, numbers and Booleans. When a property contains a function, we call that property a 'method'. Many of the examples above are methods. The console object has many methods on it. The most commonly used one is log.
Objects that share a common set of properties and methods are said to be of the same 'class'; therefore, Strings are all of the String class, Numbers are all of the Number class, and so on.
Next, watch this video to experience An Encounter with JavaScript Objects.
Creating objects
We can create custom objects:
var car = {};
This is an empty object. It is actually of the Object class, which does have the odd method or two, but for all intents and purposes, we consider this empty.
We can fill the car with properties like so:
var car = { make: "Morris", model: "1100", year: 1966, paintColor: "blue", odometer: 207293.1, // km fuel: 30, // l maxFuel: 38.6, // l kmPerLitre: 13.9, // kpl };
- Some of these properties are likely to be permanent: cars do not change their make, model, year and maxFuel.
- Some will change rarely: like their colour.
- Some will change frequently: like the amount of fuel in the tank, and the odometer.
The name of a property is sometimes called a key.
Accessing properties
We use the . Member operator to access properties of objects.
console.log(car.make + " " + car.model); car.paintColor = "red"; car.odometer += 15; car.fuel -= 1.07;
Sometimes, we want to access properties programmatically. We can pass a variable into some square brackets like we would an array:
var prop = "make"; console.log(car[prop]); // => "Morris" car["paintColor"] = "green"; // This would be better written as car.paintColor.
JavaScript Object Notation (JSON)
Objects that are described in the curly brace notation can sometimes be valid JSON--a popular way to share data records between servers and browsers. JSON has some stricter rules than JavaScript, and works with not just JS, but every popular programming language.
Creating methods
When we put a function into a property, we call that function a 'method'. Methods can use the this keyword to refer to the object they are a part of:
car.drive = function (distanceKm) { var fuelConsumption = distanceKm / this.kmPerLitre; if (fuelConsumption > this.fuel) { return false; } this.fuel -= fuelConsumption; this.odometer += distanceKm; return this.odometer; } car.fuel = car.maxFuel; console.log(car.odometer); // => 207328.1 console.log(car.drive(20)); // => 207328.1 console.log(car.fuel); // => 37.161151079136694
These coding standards will help you write JavaScript code that is free of syntactical problems. It includes:
- variable naming
- whitespace and semicolon placement
- different statement guidelines.
It can also help with code quality, readability and maintenance. Coding standards can help with:
- keeping the code consistent
- making it easier to read and understand
- making it easier to maintain.
Naming conventions
Always use the same naming convention for all your code.
For example:
- do use camelCasing for variables, function names and function argument names
- do use PascalCasing for global variables
- do use UPPERCASE for constants (like PI)
- do not use under_scores in variable, constants, function arguments or function names
- do not use hyphens in JavaScript names.
Do use camelCasing for function names:
function helloWorld() { // Code block }
Do use camelCasing for function arguments and local variables:
function hello(isShow) { // Code block } var firstName = "Jane" var lastName = "Doe" var price = 19.90 var discount = 0.10 var fullPrice = price * 100 / discount;
Indentation
Indentation and line breaks add readability to complex statements.
Always indent code using 2 spaces. Code must not end with trailing whitespaces.
Tabs should be used for indentation. Even if the entire file is contained in a closure (i.e. an immediately invoked function), the contents of that function should be indented by one tab.
For example:
(function ($) { // Expressions indented function doSomething() { // Expressions indented } })(jQuery);
Web coding
The following are industry standard best practice measures.
You are highly encouraged to follow these practices and incorporate them into your coding practice.
HTML
When producing HTML for a web project, you should:
- Use the correct HTML5 doctype
- Validate HTML
- Do not use deprecated tags
- Remove redundant tags and commented out tags
- Do not use HTML for styling purposes
- Use the alt attribute for all images
- Format your code
- Ensure all file, image and anchor links work
- Use a consistent HTML structure for all pages unless justified
- Delivered markup as utf-8 (the most friendly for internationalisation)
- Do not use tables for layout unless justified
- Do not use div or p tags for lists unless justified
- Use label fields to label each form field
- If nesting views across files (like in some WordPress templates), use HTML comments to indicate which elements you are closing
- Do not use images where CSS styles could be used instead
- Use title-case for headers and titles and use CSS to apply all caps or all lowercase; i.e. text-transform:uppercase/lowercase.
CSS
When producing CSS, you should:
- Plan and create your HTML first
- Use CSS to adhere to a responsive design plan
- Use only one style sheet per page (IE conditional styles excepted)
- Validate your CSS
- Use comments to add meaning
- Include a CSS reset at the start of the style sheet
- Organise the style sheet with a top-down structure from generic to specific
- Use classes (or multiple classes), combine rules and take other measures to reduce repetition
- All CSS should be consistent with a methodology (such as SMACSS, BEM, or OOCSS)
- Reduce specificity as much as your CSS methodology will allow
- Selectors must be named consistently and meaningfully, not start with numbers and have no spaces or special characters
- Use absolute positioning only when default positioning cannot fulfil a use case
- If using floats, remember to clear them
- Do not use an image where there is a CSS alternative
- Use external files unless justified.
JavaScript
When producing JavaScript, you should:
- Use external scripts unless justified
- Minimise the number of external scripts a page uses
- Acknowledge all third party scripts using comments
- Code should be written to the DRY principle (i.e. no repetition). Create functions that take parameters, and return values to foster code reuse
- Comment your code
- Ensure site is usable when JavaScript is turned off
- Use consistent naming: functionNamesLikeThis, variableNamesLikeThis, ClassNamesLikeThis, EnumNamesLikeThis, methodNamesLikeThis, CONSTANT_VALUES_LIKE_THIS, and file-names-like-this-1.0.min.js
- Name functions and variables logically
- Prefer single 'quotes' over double "quotes"
- Always declare variables with var (or in ES6 - var, let, const)
- Always use semicolons
- Do not declare a named function within a block
- Minimise the use of global variables
- Separate event handling from functionality
- Minify all JavaScript for production
- Use a JavaScript linting tool
- Organise your code into the Modular Pattern, Object Literal/Singleton, or Objects with Constructors where possible.
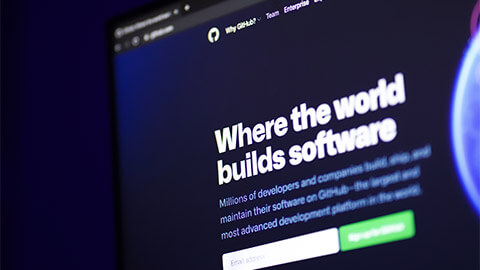
GitHub is a website and cloud-based service that allows developers to store and manage their code while also tracking and controlling changes. To fully grasp what GitHub is, you must first understand 2 connected principles:
- Version control
- Git.
What is version control?
'Developers can use version control to keep track of and manage changes to a software project's code. Version control allows developers to safely branch and merge code. With branching, a developer duplicates part of the source code (called the repository). The developer can then safely make changes to that part of the code without affecting the rest of the project.
Then, once the developer gets his or her part of the code working properly, he or she can merge that code back into the main source code to make it official. All of these changes are then tracked and can be reverted if need be.'8
Every developer can see these new changes, download them and contribute.
What is Git?
'Git is a specific open-source version control system created by Linus Torvalds in 2005.'8
The 'Hub'
While 'Git' is a version control system, the 'Hub' is the centre around which all things revolve--this is where developers store projects and network with like-minded people.
Let us look at some of the common terms that you will need to understand when using GitHub.
- A repository (repo) is a folder where all of the files for a project are kept. Each project has its own repository, which can be accessed by a unique URL.
- A branch is a workspace in which you can make changes that will not affect the live site.
'Specifically, Git is a distributed version control system, which means that the entire codebase and history is available on every developer’s computer, which allows for easy branching and merging.'
Some of the features that make GitHub so dynamic are:
- Git clone
- Git add
- Git commit
- Git push.
Git clone—is a Git command-line tool that allows you to target an existing repository and produce a clone, or duplicate of it.
Git add–the command adds a modification to the staging area from the working directory.
Git commit–the command takes a snapshot of the current state of the project's changes.
Git push–the command is used to transfer content from a local repository to a remote repository.
The following video shows you how to create your own Github account.
Now that you know what GitHub is about, are you ready to get started? Head over to GitHub.com and try for yourself.
Knowledge check
Loop practice
Display 0 to 9
Display a row of 20 stars (*)
Request a number and display a row of that many stars (*)
Find out the sum from 1 to 20 (1 + 2 + 3 + 4 +..+ 19 + 20)
Find out the sum of 1 + 1/2 + 1/3 + 1/4 +..+ 1/100
Find out the sum of odd numbers from 1 to 20
Salary estimator
Write a program to estimate how much a young worker will make before retiring at 65. Request the worker's name, age and starting salary as input. Assume the worker receives a 5 per cent raise each year. For example, if the user enters Helen, 25 and 20000, then the output is:
Helen will earn about $2,415,995.
Best way to get paid
You are offered 2 salary options.
- Option 1: $100 per day
- Option 2: $1 the first day, $2 the second day, $4 the third day, $8 the fourth day, and so on with the amount doubling daily.
Write a program to ask the number of workdays and determine which option pays better.
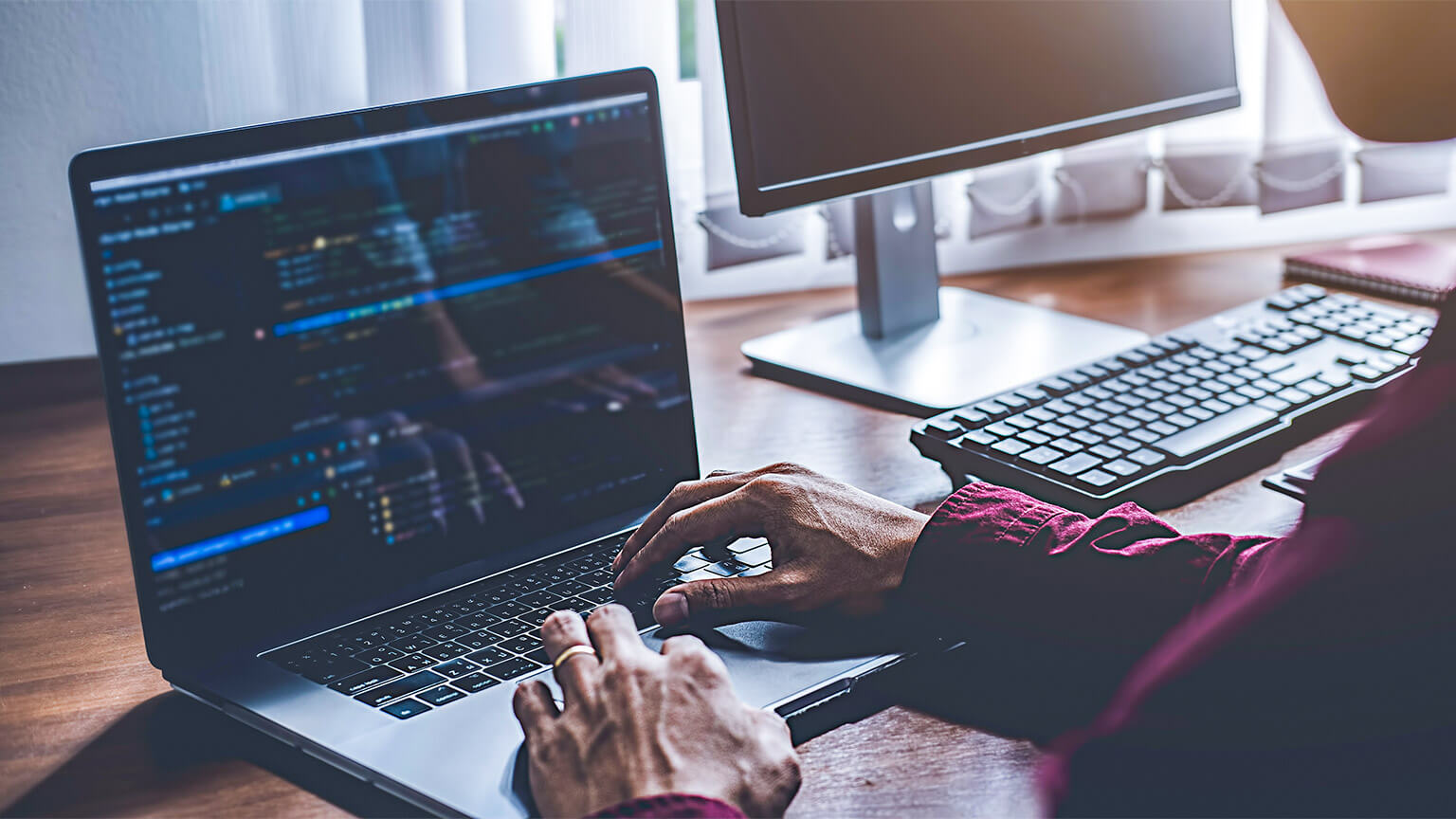