In this topic, we will cover:
- Browser environment
- Navigating the DOM
- Searching
- DOM nodes
- Manipulating DOM elements.
The host environment
JavaScript was created for web browsers but now can be used on a variety of platforms including browsers, web servers or another host. Each platform has different platform-specific functionality. JavaScript specification calls this a 'host environment'.
Host environments provide their own objects and functions, which are in addition to the language core.
This is what is going on when JavaScript runs in a web browser:
Window is a root object. It is a global object for JavaScript code and represents the browser window. It provides methods to control the browser methods.
For example, here we use window as a global object:
function sayHi() { alert("Hello"); } // Global functions are methods of the window object. window.sayHi();
And here we use it as a browser window, to see the window height12:
alert(window.innerHeight); // Inner window height.
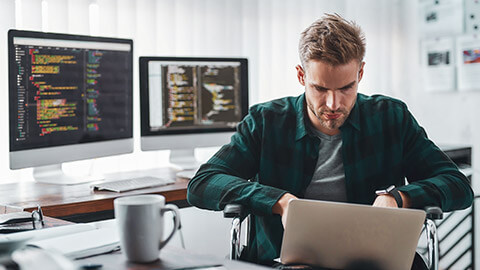
DOM (Document Object Model)
The Document Object Model (DOM) represents everything on the page content as objects that can be modified. We can use the document object to create or alter anything on the page–it is the 'entry point' to the page.
For example:
// Change the background color to blue. document.body.style.background = "blue"; // Change it back after two seconds. setTimeout(() => document.body.style.background = "", 2000);
The example uses document.body.style, but there are a lot of properties and methods that you can change. The DOM Living Standard has a complete specification list.
DOM is a W3C standard
'The W3C Document Object Model (DOM) is a platform and language-neutral interface that allows programs and scripts to dynamically access and update the content, structure, and style of a document.'6
'The Document Object Model (DOM) is a programming API [Application Programming Interface] for HTML and XML documents. It defines the logical structure of documents and the way a document is accessed and manipulated.'19
There are 3 parts to the W3C DOM standard:
- Core DOM: standard model for all document types
- XML DOM: standard model for XML documents
- HTML DOM: standard model for HTML documents.
'In the DOM specification, the term "document" is used in the broad sense–increasingly, XML is being used as a way of representing many different kinds of information that may be stored in diverse systems, and much of this would traditionally be seen as data rather than as documents. Nevertheless, XML presents this data as documents, and the DOM may be used to manage this data.
'With the Document Object Model, programmers can create and build documents, navigate their structure, and add, modify, or delete elements and content. Anything found in an HTML or XML document can be accessed, changed, deleted, or added using the Document Object Model, with a few exceptions–in particular, the DOM interfaces for the internal subset and external subset have not yet been specified.
'As a W3C specification, one important objective for the Document Object Model is to provide a standard programming interface that can be used in a wide variety of environments and applications. The Document Object Model can be used with any programming language.'19
Not just for browsers
The DOM specification details the structure of a document and provides objects to manipulate it.
It can also use non-browser instruments such as server-side scripts that download and process HTML pages.
HTML DOM
'The HTML DOM is a standard object model and programming interface for HTML. It defines:
- The HTML elements as objects
- The properties of all HTML elements
- The methods to access all HTML elements
- The events for all HTML elements.
'In other words: The HTML DOM is a standard for how to get, change, add, or delete HTML elements.'20
Watch the following video for a summary of what the DOM is and what we can do with it. Pay attention to how objects can be laid out in a hierarchal tree diagram. The video also introduces the concept of 'nodes' which we will explore in the next subtopic.
Browser Object Model (BOM)
You will note the BOM is also referenced regarding the host environment. We will not look at the BOM in detail, but you may be interested to know that the 'Browser Object Model (BOM) represents additional objects provided by the browser (host environment) for working with everything except the document'.12
This could include providing background information relating to the browser and OS using the navigator object:
navigator.userAgent
provides detail about the current browsernavigator.platform
provides detail about the platform (Windows/Linux/Mac).
You can also use the location object to read the current URL and redirect the browser to a new one. For example:
alert(location.href); // Shows current URL. if (confirm("Go to Wikipedia?")) { location.href = "https://www.wikipedia.org/"; // Redirect the browser to another URL. }
Other functions (including alert, confirm and prompt) are also a part of BOM. They represent pure browser methods of communicating with the user, and do not directly relate to the document.12
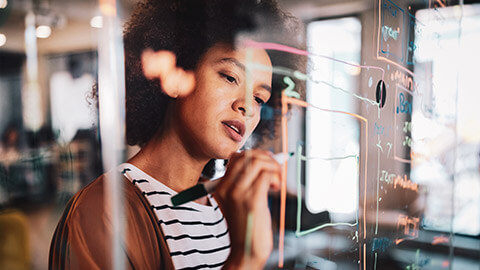
DOM tree
Tags are the backbone of an HTML document and in the Document Object Model (DOM), these tags are 'objects'. Text inside a tag is an object as well. Nested tags are referred to as 'children' of the enclosing tag.
We can access all these objects using JavaScript and use them to modify the page.
Consider the following example where document.body
is the object representing the <body>
tag. Executing the code will make the <body>
green for 2 seconds:
// Change the background color to green. document.body.style.background = "green"; // Change it back after two seconds. setTimeout(() => document.body.style.background = "", 2000);
So, we can use style.background
to change the background colour of document.body
. We can modify other properties too, such as the HTML contents of the node using innerHTML and the node width using offsetWidth
.
Before we become too involved with manipulating the DOM, we will learn about its structure and how to navigate it.
Here is a simple document:
<!DOCTYPE html> <html> <head> <title>Whio don’t quack</title> </head> <body> Whio whistle and purr </body> </html>
DOM represents the HTML as a 'tree structure' of tags.
▼ HTML ├── ▼ HEAD │ ├── #text │ ├── ▼ TITLE │ │ └── #text "Whio don't quack" │ └── #text ├── #text ├── ▼ BODY │ └── #text "Whio whistle and purr" └── #text
Using the HTML DOM, you are able to navigate the node tree using node 'relationships'.
'Everything in an HTML document is a node:
- The entire document is a document node
- Every HTML element is an element node
- The text inside HTML elements are text nodes
- Every HTML attribute is an attribute node (deprecated)
- All comments are comment nodes.
'With the HTML DOM, all nodes in the node tree can be accessed by JavaScript. New nodes can be created, and all nodes can be modified or deleted.'21
Node relationships
There is a hierarchical relationship that links nodes to each other in the node (or DOM) tree. These relationships are described using the terms 'parent', 'child' and 'sibling'.
- 'The top node is called the root (or root node)
- Every node has exactly one parent, except the root (which has no parent)
- A node can have a number of children
- Siblings are nodes with the same parent.'21
Consider the following HTML:
<!DOCTYPE html> <html> <head> <title>Songs about birds</title> </head> <body> <h1>Ducks</h1> <p>Five little ducks went swimming one day</p> </body> </html>
Note: The <head>
and <body>
elements—the children of the <html>
element—are siblings to one another.
From this, we can read that:
<html>
is the root node<html>
has no parents<html>
is the parent of<head>
and<body>
<head>
is the first child of<html>
<body>
is the last child of<html>
.
and:
<head>
has one child:<title>
<title>
has one child (a text node):"Songs about birds"
<body>
has two children:<h1>
and<p>
<h1>
has one child:"Ducks"
<p>
has one child:"Five little ducks went swimming one day"
<h1>
and<p>
are siblings.21
Navigating between nodes
Use the following node properties to navigate between nodes with JavaScript:
parentNode
childNodes[nodeNumber]
firstChild
lastChild
nextSibling
previousSibling
.
Walking the DOM
We can do anything with elements and their contents using the DOM. But first, we need to reach the relevant DOM object.
All operations on the DOM start with the document object. Think about the document object as the main 'entry point' to DOM: from here, we can access any node.
The links that allow for travel between DOM nodes are illustrated below.
DocumentElement and body
'The topmost tree nodes are available directly as document properties:
<html> = document.documentElement;
'The topmost document
node is document.documentElement
. That [is] the DOM node of the <html>
tag.
<body> = document.body;
'Another widely used DOM node is the <body>
element – document.body
.
<head> = document.head;
'The <head>
tag is available as document.head
.'12
Null
Note that document.body
can be null
.
If an element does not exist at the moment of execution, a script cannot access it. If a script is inside <head>
, then document.body
is unavailable, because the browser has not read it yet.
Consider the following example. The first alert shows null
:
<!DOCTYPE html> <html> <head> <script> alert("From HEAD: " + document.body); // => null </script> </head> <body> <script> alert("From BODY: " + document.body); // => HTMLBodyElement </script> </body> </html>
In the DOM, the null
value means 'no such node' or 'does not exist'.12
childNodes, firstChild, lastChild
There are 2 terms we will use from now:
- 'Child nodes (or children)–elements that are direct children. In other words, they are nested exactly in the given one. For instance,
<head>
and<body>
are children of<html>
element. - Descendants–all elements that are nested in the given one, including children, their children and so on.
'For instance, here <body>
has children <div>
and <ul>
(and few blank text nodes):
<!DOCTYPE html> <html> <head> <title>Example Document</title> </head> <body> <div>Begin</div> <ul> <li><b>Information</b></li> </ul> </body> </html>
'…And descendants of <body>
are not only direct children <div>
, <ul>
but also more deeply nested elements, such as <li>
(a child of <ul>
) and <b>
(a child of <li>
)–the entire subtree.'12
All child nodes, including text nodes, are listed in the childNodes
collection.
The following example shows children of document.body
:
<!DOCTYPE html> <html> <head> <title>Example Document</title> </head> <body> <div>Begin</div> <ul> <li><b>Information</b></li> </ul> <div>End</div> </script> for (var i = 0; i < document.body.childNodes.length; i++) { alert(document.body.childNodes[i]); // => DIV, Text node, UL, LI, ..., SCRIPT } </script> <!-- More stuff. --> </body> </html>
If we run this code, the last element shown is <script>
even though the document has 'More stuff' below. This is because at the moment of the execution, the browser had not read it yet, so the script does not see it.
The properties firstChild
and lastChild
provide quick access to the first and last children, respectively. They work like shorthand. If child nodes exist, then the following is always true:
elem.childNodes[0] === elem.firstChild; elem.childNodes[elem.childNodes.length - 1] === elem.firstChild;
A special function called elem.hasChildNodes()
checks whether there are any child nodes.12
DOM collections
object. This means that:
- we can iterate over it using for..of
- array methods will not work–it is not an array.
For example:
for (var node of document.body.childNodes) { alert(node); // => All nodes from the specified collection. }
And:
alert(document.body.childNodes.filter); // => undefined
However, if we do want to use array methods, we can use elem.from()
to create a 'real' array from the collection; such as:
alert(Array.from(document.body.childNodes).filter); // => function
Read-only
DOM collections are read-only. It is not possible to replace a child by something else by assigning childNodes[i] = ...
.
DOM collections are live
With some minor exceptions, all DOM collections are live. They reflect the current state of the DOM.
If we keep a reference to elem.childNodes
, and add nodes to (or remove nodes from) the DOM, then they appear in the collection automatically.
Do not loop over collections using for..in
You can iterate collections using for...of
. Sometimes people try to use for...in
to achieve that. Do not do this.
The for...in
loop iterates over all enumerable properties. Collections have some extra, rarely used properties that we usually want to avoid.
For example12:
<!DOCTYPE html> <html> <head> <title>Example Document</title> </head> <body> </script> for (var prop in document.body.childNodes) alert(prop); // Shows 0, 1, length, item, values and more. </script> </body> </html>
Siblings and the parent
Siblings are nodes that are children of the same parent. Consider the following:
<!DOCTYPE html> <html> <head> ... </head> <body> ... </body> </html>
In the example <head>
and <body>
are siblings:
<body>
is the 'next' or 'right' sibling of<head>
<head>
is the 'previous' or 'left' sibling of<body>
.
The nextSibling
property contains the next sibling, and the previous sibling is in the previousSibling
property. The parent is available as parentNode
.
For example:
// The parent of <body> is <html>. alert(document.body.parentNode === document.documentElement); // => true // After <head> is <body>. alert(document.head.nextSibling); // => HTMLBodyElement // Before <body> is <head>. alert(document.body.previousSibling); // => HTMLHeadElement
Element-only navigation
Navigation properties explored so far refer to all nodes. So, in childNodes
we see text nodes, element nodes, and even comment nodes if they exist.
Often, we do not want text or comment nodes. Instead, we want to manipulate element nodes that represent tags and form the structure of the page.
Here are our navigation links that only consider element nodes:
The links are very similar to the previous navigation properties but this time they have the word 'Element' inside:
children
– only children that are element nodes.firstElementChild
,lastElementChild
– first and last element children.previousElementSibling
,nextElementSibling
– neighbour elements.parentElement
– parent element.
A closer look at the parentElement
'The parentElement
property returns the "element" parent, while parentNode
returns "any node" parent. These properties are usually the same: they both get the parent.
'With the one exception of document.documentElement
:
alert(document.documentElement.parentNode); // => document alert(document.documentElement.parentElement); // => null
'The reason is that the root node document.documentElement
(<html>
) has document
as its parent. But document
is not an element node, so parentNode
returns it and parentElement
does not.
'This detail may be useful when we want to travel up from an arbitrary element elem
to <html>
, but not to the document
'12:
// Go up till <html>. while (elem = elem.parentElement) { alert(elem); }
Replacing childNodes
'In the following example, we replace childNodes
with children
. Now it shows only elements12:
<!DOCTYPE html> <html> <head> ... </head> <body> <div>Begin</div> <ul> <li>Information</li> </ul> <div>End</div> <script> for (var elem of document.body.children) { alert(elem); // => DIV, UL, DIV, SCRIPT } </script> </body> </html>
Tables
So far, we have looked at basic navigation properties. Some types of DOM elements, such as tables, provide additional properties for convenience.
The <table>
element supports these additional properties:
table.rows
– the collection of<tr>
elements of the tabletable.caption/tHead/tFoot
– references to elements<caption>
,<thead>
,<tfoot>
;table.tBodies
– the collection of<tbody>
elements. There will always be at least one–even if it is not in the source HTML, the browser will put it in the DOM.
<thead>
, <tfoot>
, <tbody>
elements provide the rows property:
tbody.rows
– the collection of<tr>
inside.
<tr>
:
tr.cells
– the collection of<td>
and<th>
cells inside the given<tr>
.tr.sectionRowIndex
– the position (index) of the given<tr>
inside the enclosing</thead></tbody></tfoot>
.tr.rowIndex
– the number of the<tr>
in the table as a whole (including all table rows).
<td>
and <th>
:
td.cellIndex
– the number of the cells inside the enclosing<tr>
.
Here is an example in practice12:
<table> <tr> <td>One</td> <td>Two</td> </tr> <tr> <td>Three</td> <td>Four</td> </tr> </table> <script> // Get td with "Two" (first row, second column). var td = table.rows[0].cells[1]; td.style.backgroundColor = "red"; // Highlight it. </script>
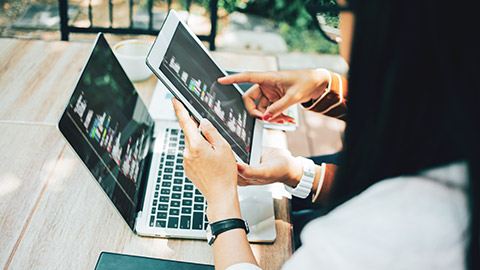
getElement*
When elements are close to each other, navigating the DOM is reasonably straightforward. But what if they are not close to each other?
document.getElementById
(or just id) provides an additional search method.
If an element has the id attribute, we can use the method document.getElementById(id)
to get the element, regardless of where it is.
For example:
A global variable id references the element:
The above is true unless we declare a JavaScript variable with the same name, which would take precedence.
<div id="elem"></div> <script> var elem = 5; // => Now elem is 5, not a reference to <div id="elem"> alert(elem); // => 5 </script>
Do not use id-named global variables to access elements. This behaviour is described in the specification, so is standard. However, it is mostly supported for compatibility reasons. It can create naming conflicts or confusion when you are only reading JavaScript, and not the HTML.
While we use id here, it is purely for brevity when it is clear where the element has come from. In practice, use the document.getElementById
method.
The id must be unique
Only one element in the document should have the given id. If multiple elements have the same id, behaviour of methods can be unpredictable.
Only document.getElementById, not anyElem.getElementById
'The method getElementById()
can be called only on document object. It looks for the given id in the whole document.'22
querySelectorAll
elem.querySelectorAll(css)
is the most versatile method, returning all elements inside elem
matching the given CSS selector.
In the following example, we are looking for all <li>
elements that are last children:
<ul> <li>The</li> <li>test</li> </ul> <ul> <li>has</li> <li>passed</li> </ul> <script> var elements = document.querySelectorAll("ul > li:last-child"); for (var elem of elements) { alert(elem.innerHTML); // => "test", "passed" } </script>
Any CSS selector can be used, which makes this method is really useful.
Pseudo-classes in the CSS selector such as :hover and :active are also supported. For example, document.querySelectorAll(":hover")
returns the collection with elements that the pointer is over now (in nesting order: from the outermost <html>
to the most nested one).22
querySelector
The first element for the given CSS selector is returned using the call to elem.querySelector(css)
.
So, the result is the same as elem.querySelectorAll(css)[0]
, but the latter is looking for all elements and picking one, while elem.querySelector()
just looks for one. This makes querySelector()
faster. It is also shorter to write.
matches
getElement()
* and querySelector()
* search the DOM. Whereas the elem.matches(css)
does not look for anything, it just checks if elem
matches the given CSS-selector. It returns true
or false
.
This is helpful when we are iterating over elements (such as an array) and want to filter out those of interest.
For example:
<a href="http://example.com/file.zip">...</a> <a href="http://ya.ru">...</a> <script> // Can be any collection instead of document.body.children. for (var elem of document.body.children) { if (elem.matches("a[href$='zip'")) { alert("The archive reference: " + elem.href); } } </script>
closest
An element has ancestors: the parent, the parent of the parent, its parent and so on. Together, the ancestors form the chain of parents from the element to the top.
'The method elem.closest(css)
looks for the nearest ancestor that matches the CSS-selector. The element itself is also included in the search.
'In other words, the method closest goes up from the element and checks each of the parents. If it matches the selector, then the search stops, and the ancestor is returned.'22
For example:
<h1>Contents</h1> <div class="contents"> <ul class="book"> <li class="chapter">Chapter 1</li> <li class="chapter">Chapter 2</li> </ul> </div> <script> var chapter = document.querySelector(".chapter"); // => LI alert(chapter.closest(".book")); // => UL alert(chapter.closest(".contents")); // => DIV alert(chapter.closest("h1")); // => null (H1 is not an ancestor) </script>
getElementsBy*
It is also possible to look for nodes by a tag, class, and so on. The following methods are generally historic and have been replaced by querySelector()
. You may find them in old scripts.
document.getElementsByTagName(tagName)
finds elements that have the given tag and returns the collection of them. The tag parameter can be asterisks"*"
for “any tags”.document.getElementsByClassName(className)
finds elements that have the given CSS class.document.getElementsByName(name)
finds elements with the given name attribute, document-wide. This is rarely used.
Note that the above methods use plural 'Elements' and return a collection of elements, not a single element as is the case with document.getElementsById()
.
All methods "getElementsBy*" return a live collection. They always reflect the current state of the document and "auto-update" when it changes. Whereas, querySelectorAll()
returns a static collection, similar to a fixed array of elements.22
How do the search methods compare?
We have looked at the 6 main methods to search for nodes in the DOM. The most common methods are querySelector()
and querySelectorAll()
. In addition, getElementBy()
* and getElementsBy()
* can be helpful and may be found in old scripts.22
Method | Searches by | Can call on an element? | Live or static? |
---|---|---|---|
querySelector() |
".selector" |
Yes | Static |
querySelectorAll() |
".selector" |
Yes | Static |
getElementById() |
"id" |
No | Static |
getElementsByName() |
"name" |
No | Live |
getElementsByTagName() |
"tag" or "*" |
Yes | Live |
getElementsByClassName() |
"class" |
Yes | Live |
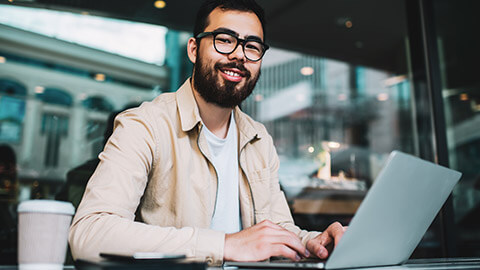
Properties and methods
You have seen how the DOM organises objects within a webpage into a hierarchal tree, and that each node (object) can be accessed in JavaScript. Now, we will look closer at the properties and methods you can use to manage them.
Basic node properties
Every node has basic properties that you can examine or set, 'including the following:
nodeName
—The name of the node (not the ID). For nodes based on HTML tags, such as<p>
or<body>
, the name is the tag name:p
orbody
. For the document node, the name is a special code:#document
. Similarly, text nodes have the name#text
. This is a read-only value.nodeType
—An integer describing the node’s type, such as1
for normal HTML tags,3
for text nodes, and9
for the document node. This is a read-only value.nodeValue
—The actual text contained within a text node. This property returnsnull
for other types of nodes.innerHTML
—The HTML content of any node. You can assign a value including HTML tags to this property and change the DOM child objects for a node dynamically.'7
While innerHTML
is supported by major browsers, it is not part of the W3C DOM specification. In most instances, it is okay to use. However, if your webpage needs to be 100 per cent standards compliant, do not use it.
The most important nodeType properties are21:
Node | Type |
---|---|
ELEMENT_NODE |
1 |
ATTRIBUTE_NODE |
2 |
TEXT_NODE |
3 |
COMMENT_NODE |
8 |
DOCUMENT_NODE |
9 |
DOCUMENT_TYPE_NODE |
10 |
Remember that each node also has properties that describe its relationships to other nodes. For example, firstChild
, nextSibling
and so on.
Document methods
We have already discussed several document methods for navigating the DOM. Additional document node methods that you may find helpful include:
createTextNode(text)
—Creates a new text node containing the specified text, which you can then add to the document.createElement(tag)
—Creates a new HTML element for the specified tag. As withcreateTextNode()
, you need to add the element to the document after creating it. You can assign content within the element by changing its child objects or theinnerHTML
property.7
Node methods
'Each node within a page has a number of methods available. Which of them are valid depends on the node’s position in the page and whether it has parent or child nodes. These methods include the following:
appendChild(new)
—Appends the specified new node after all the object’s existing nodes.insertBefore(new, old)
—Inserts the specified new child node before the specified old child node, which must already exist.replaceChild(new, old)
—Replaces the specified old child node with a new node.removeChild(new)
—Removes a child node from the object’s set of children.hasChildNodes()
—Returns the Boolean value true if the object has one or more child nodes or false if it has none.cloneNode()
—Creates a copy of an existing node. If a parameter of true is supplied, the copy will also include any child nodes of the original node.'7
Watch the video for an overview of nodes, how to find their properties, and see node methods in action.
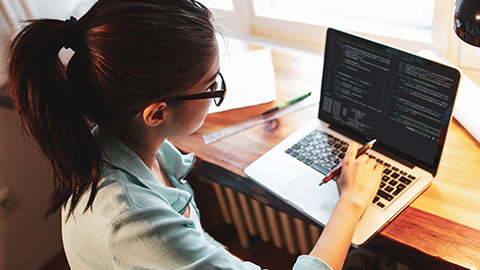
With an understanding of the DOM structure, you can start thinking about how to control any element in a webpage. For example, you can use the DOM to change the position, visibility, and other attributes of an element (such as a paragraph or image).
While you can position elements individually, it can be highly useful to work with groups of elements.
You can create a layer, or a group of HTML objects, by using the <div>
container element.
'To create a layer with <div>
, enclose the content of the layer between the <div>
and </div>
tags and specify the layer’s properties in the style attribute of the <div>
tag.
For example:
<div id="layer1" style="position: absolute; top: 100px; left: 100px;"> This is the content of the layer. </div>
This code defines a layer with the name layer1. This is a movable layer positioned 100px down and 100 pixels to the right of the upper-left corner of the browser window.'7
Controlling positioning with JavaScript
You can control the positioning attributes of an object by using JavaScript. We will look at a code snippet to view this in action.
'Here is our sample layer (a <div>
):
<div id="layer1" style="position: absolute; top: 100px; left: 100px;"> This is the content of the layer. </div>
'To move this layer up or down within the page by using JavaScript, you can change its style.top
attribute. For example, the following statements move the layer 200px
down from its original position:
var obj = document.getElementById("layer1"); obj.style.top = 200;
'The document.getElementById method returns the object corresponding to the layer’s <div>
tag, and the second statement sets the object’s top positioning property to 200px
you can also combine these two statements, like so:
document.getElementById("layer1").style.top = 200;
'This simply sets the style.top
property for the layer without assigning a variable to the layer’s object.
'Note: Some CSS properties, such as text-indent and border-color, have hyphens in their names. When you use these properties in JavaScript, you need to combine the hyphenated sections and use camel case: textIndent
and borderColor
.'7
Hiding and showing objects
'As a refresher, objects have a visibility style property that specifies whether they are currently visible within the page:
object.style.visibility = "hidden"; // Hides an object. object.style.visibility = "visible"; // Shows an object.
'Using this property, you can create a script that hides or shows objects in either browser.'7
The following example 'shows the HTML document for a script that allows two headings to be shown or hidden.
'The <h1>
tags in this document define headings with the ids heading1 and heading2. Inside the <form>
element are two check boxes, one for each of these headings. When a check box is modified (checked or unchecked), the onclick method calls the JavaScript showHide function to perform an action.
'The showHide function is defined within the <script>
tag at the bottom of the document. This function assigns the objects for the two headings to two variables named heading1 and heading2, using the getElementById()
method. Next, it assigns the value of the check boxes within the form to the showheading1
and showheading2
variables. Finally, the function uses the style.visibility
attributes to set the visibility of the headings.
'Note: The lines that set the visibility property might look a bit strange. The ? and : characters create conditional expressions, a shorthand way of handling if statements.'7
Modifying text in a page
‘You can create a simple script to modify the contents of a heading (or any element, for that matter) within a web page. The nodeValue
property of a text node contains its actual text, and the text node for a heading is a child of that heading. So, this would be how to change the text of a heading with the id heading1:
var heading1 = document.getElementById("heading1"); heading1.firstChild.nodeValue = "New text here.";
'This assigns the heading’s object to the variable called heading1
. The firstChild
property returns the text node that is the only child of the heading, and its nodeValue
property contains the heading text.
'Using this technique, it is easy to create a page that allows the heading to be changed dynamically.
'For example:
'This example defines a form that enables the user to enter a new heading for the page. Clicking the button calls the changeTitle()
function, defined in the <script>
tag at the bottom of the document. This JavaScript function gets the value the user entered in the form and changes the heading’s value to the new text by assigning the value of the input to the heading1.firstChild.nodeValue
property.'7
Adding text to a page
'Next, you can create a script that actually adds text to a page rather than just changing existing text. To do this, you must first create a new text node. This statement creates a new text node with the text "This is a test"
:
var node = document.createTextNode("This is a test");
'Next, you can add this node to the document
. To do this, you use the appendChild()
method. The text can be added to any element that can contain text, but in this example, we will just use a paragraph. The following statement adds the text node defined previously to the paragraph with the id paragraph1:
document.getElementById("paragraph1").appendChild(node);
'The following example shows the HTML document that uses this technique, using a form to allow the user to specify text to add to the page.
'In this example, the <p>
element with the id paragraph1 is the paragraph that will hold the added text. The <form>
element is a form with a text field called sentence, and an Add! button, which calls the addText()
function when clicked.
'This JavaScript function is defined in the <script>
tag at the bottom of the document
. The addText()
function first assigns text typed in the text field to the sentence
variable. Next, the script creates a new text node containing the value of the sentence
variable and appends the new text node to the paragraph.
'Load this document into a browser to test it and try adding several sentences by typing them and clicking the Add! Button.'7
Knowledge check
Navigating the DOM and manipulating DOM elements
First, navigate the DOM. Go to en.wikipedia.org/wiki/JavaScript and create scripts that will produce the following outputs:
"Here we are at: JavaScript - Wikipedia, the free encyclopedia"
"You came from: https://en.wikipedia.org/wiki/Main_Page"
"There are 82 p tags on the page."
"There are 130 elements with the class name of 'reference'."
"The first element with a class name of 'infobox' has the following class list: infobox vevent"
"The first element with a class name of 'infobox' has 2 classes"
"The html inside the element with an id of 'firstHeading' is: JavaScript"
"The id of the element that is the first child of an element with the id of 'siteNotice' is: centralNotice"
"The id of the element that is the first sibling of an element with the id of 'content' is: mw-navigation"
"The padding of the element with a class of 'navbox' is: 3px"
Your next task is to manipulate DOM elements. Create scripts that will implement the following:
- Set the first occurrence of an h1 element to have a font size of
3em
. - Give the first occurrence of an h1 element a class of
"hatnote"
. - Remove the styling of the element with an id of
"catlinks"
(it's the Categories box at the bottom of the page). - Programmatically hide the
"Contents"
menu using the"hide"
hyperlink that has an id of"togglelink"
.
Note: Values may have changed since the time of writing.
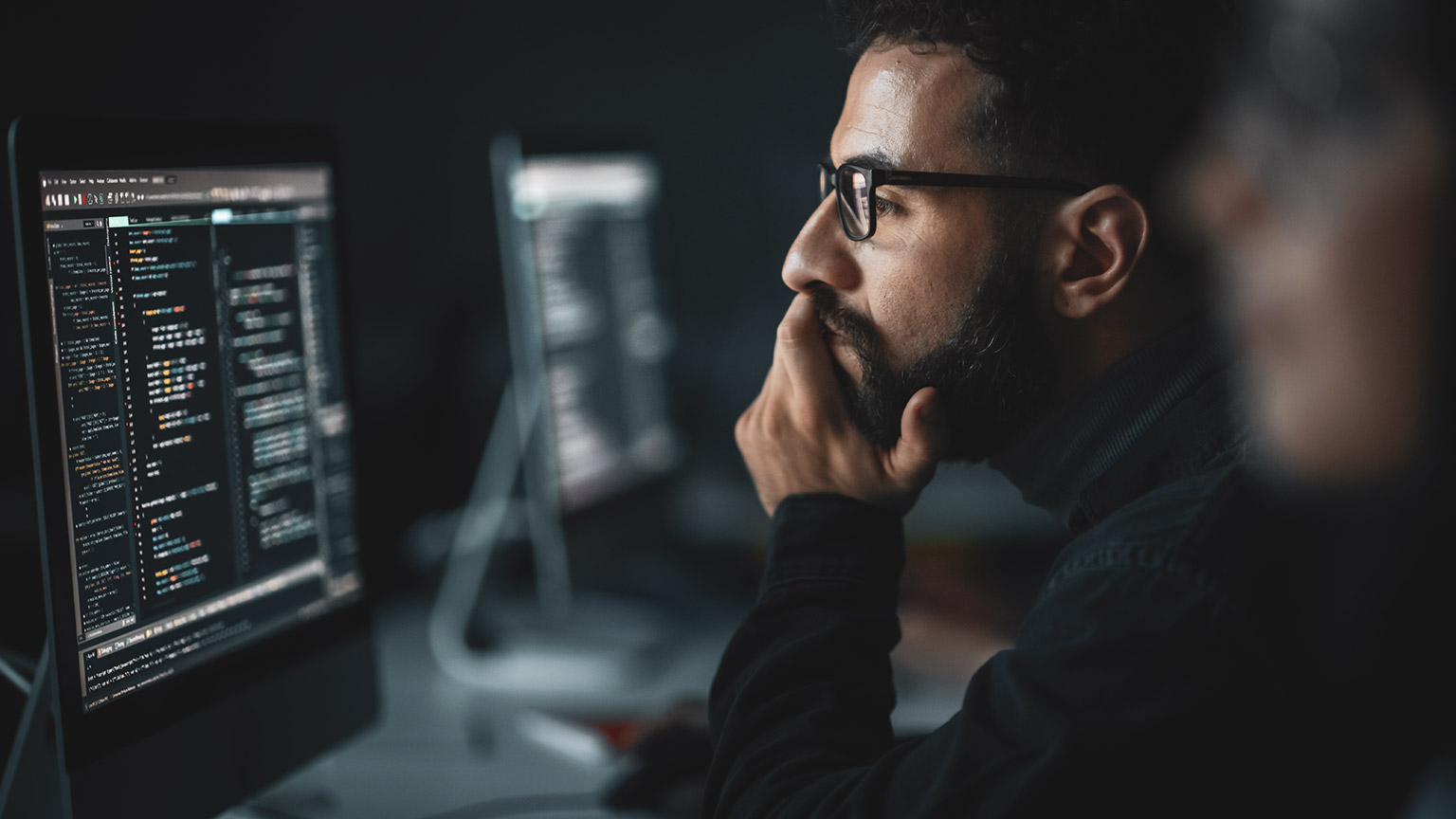