In this topic, we will cover:
- JSON
- Concepts.
JSON (JavaScript Object Notation) is a version of the JavaScript (JS) language that organises and structures data using JS's numerous syntactical patterns. It is very simple to convert JSON to JS objects, and to serialise JS objects into JSON.
JSON looks a lot like JS's object and array literals so it is easy to mix up JSON and JS, but knowing the differences is crucial:
- JSON = data format
- JS = programming language.
JSON lets you represent 3 types of data: simple values, objects and arrays.
Simple values
You can represent simple values in JSON like strings, numbers, booleans and null. For example, the following line is valid JSON:
"javascript"
This JSON represents the string value of "JavaScript", and it looks exactly like a normal JavaScript string. Do you see the big "difference" between strings in JavaScript and JSON yet?
JSON strings must use double-quotes (").
As a result, the following is invalid JSON:
'javascript'
Numeric data is represented by what appears to be number literals, like this:
10
This is valid JSON representing the number 10. Similarly, boolean values and null look like JavaScript literals, too:
true null
Objects
'This is a JSON string:
'{ "name": "John", "age": 30, "car": null }'
'Inside the JSON string there is a JSON object literal:
{ "name": "John", "age": 30, "car": null }
'JSON object literals are surrounded by curly braces {}.
- JSON object literals contain key/value pairs
- Keys and values are separated by a colon
- Keys must be strings, and values must be a valid JSON data type:
- string
- number
- object
- array
- boolean
- null.
'Each key/value pair is separated by a comma.
'It is a common mistake to call a JSON object literal "a JSON object". JSON cannot be an object. JSON is a string format. The data is only JSON when it is in a string format. When it is converted to a JavaScript variable, it becomes a JavaScript object.'24
Arrays
JSON array represents an ordered list of values. JSON array can store multiple values: string, number, boolean or object.
In JSON array, values must be separated by commas.
The []
(square brackets) represents a JSON array.
Here is an example of JSON arrays storing number values.
[ 12, 34, 56, 43, 95 ]
Or a simple JSON array example of 4 objects.
{ "employees": [ { "name": "Ram", "email": "ram@gmail.com", "age": 23 }, { "name": "Shyam", "email": "shyam23@gmail.com", "age": 28 }, { "name": "John", "email": "john@gmail.com", "age": 33 }, { "name": "Bob", "email": "bob32@gmail.com", "age": 41 } ] }
Serialising into JSON
The stringify()
method of the JSON object literal is used to serialise a JavaScript object into JSON. It takes any value, object, or array and converts it to JSON. For example:
/** * name {String} * age {Number} * birthDate {Date} * languages {Array} */ const jsObject = { name: "Todd", age: 20, birthDate: new Date(), languages: [ "Chinese", "English" ], }
Use the stringify()
function on the JSON object to convert this object to a JSON string.
As output of this method, we get the JSON string representation of our JavaScript object, which we can directly print to the console.25
console.log(JSON.stringify(jsObject));
Check out the following video where the presenter shows you how to deserialise a JSON.
Parsing JSON
'Parsing JSON into JavaScript objects is equally simple. The JSON object has a parse()
method that parses the JSON and returns the resulting object.
var johnDoe = JSON.parse(json);
'This code parses the JSON text contained in JSON and stores the resulting object in the johnDoe variable. And here is the wonderful thing—you can immediately use johnDoe and access its properties, such as:
var fullName = johnDoe.firstName + " " + johnDoe.lastName;
'It is really no wonder why developers embraced JSON. It is easy to work with! JSON is text, so you can serialize a JavaScript object at the beginning of the drag operation and parse the JSON when the drop event fires.'2
Learn more by watching the following JSON parse tutorial.
Events
A JSON-formatted string value can be used to represent an event. The properties of the JSON object apply to the event properties of JSON events.
'The code snippets below define a JSON event type, create a JSON event and send the event into the runtime. The sample defines the CarLocUpdateEvent event type. The @public means the event type has public visibility and the @buseventtype means your application can use sendEventJson for this event type.
@public @buseventtype create json schema CarLocUpdateEvent(carId string, direction int)
'The CarLocUpdateEvent can now be used in a statement:
select count(*) from CarLocUpdateEvent(direction = 1)#time(1 min)
'The sample code to send an event is'26:
String event = "{" + "\"carId\": \"A123456\",\n" + "\"direction\": 1\n" + "}"; runtime.getEventService().sendEventJson(event, "CarLocUpdateEvent");
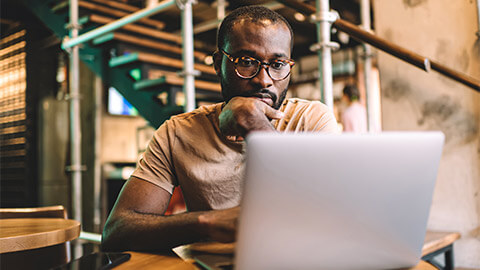
Knowledge check
Handwritten JSON
Create a file called todos.json. Write out a small set of JSON data that represents:
the data needed for the users of a 'To-do list' application.
If your text editor is not giving you enough feedback for errors, you can use a JSON linting tool to validate the JSON.
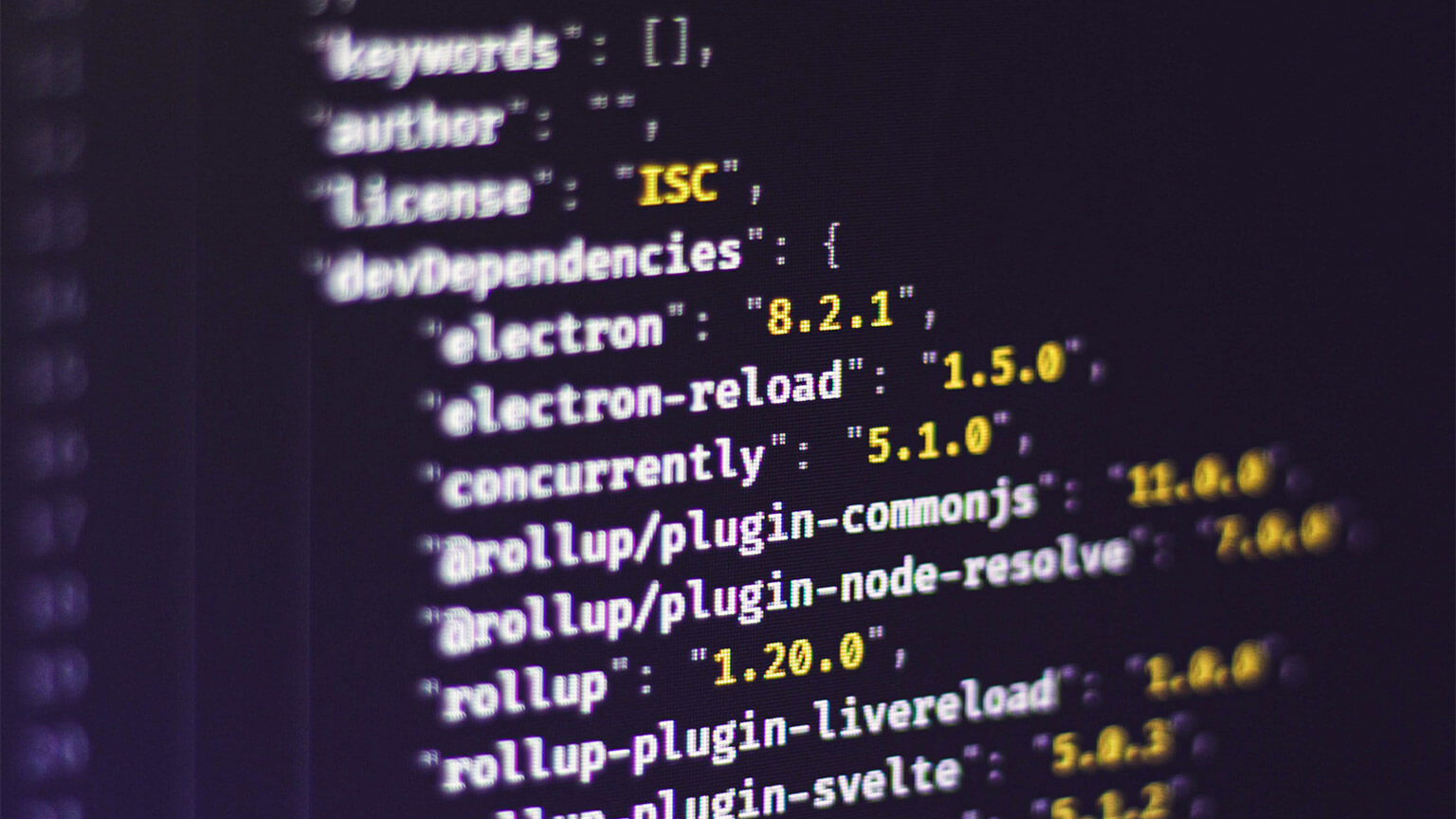