RESTful API design plays a crucial role in developing scalable and interoperable web services, particularly in the context of microservice-based architectures. REST (Representational State Transfer) is an architectural style that provides a standardised approach for building web services that are flexible, scalable, and easy to consume. This topic explores the fundamentals of RESTful API design within a microservice-based architecture, along with practical demonstrations and examples using the Django Framework and Python programming language.
The following aspects will be covered in this topic:
- Introduction to RESTful API design for a microservice-based architecture: Understanding the principles and concepts of RESTful API design is essential for developing robust and maintainable microservices. Topics such as resource identification, statelessness, uniform interface, and hypermedia as the engine of application state (HATEOAS) will be discussed. Additionally, the role of RESTful APIs in facilitating communication and integration between microservices will be explored.
- RESTful APIs using Django and Python: To provide hands-on experience and practical insights, this topic includes demonstrations and examples of building RESTful APIs using the Django Framework and the Python programming language. You will learn how to design and implement RESTful endpoints, handle HTTP methods and status codes, manage request and response payloads, and handle authentication and authorisation. The demonstrations will showcase best practices and patterns for building RESTful APIs within a microservice-based architecture.
In a microservice-based architecture, where applications are composed of small, independent services that work together to fulfil business functions, the design of RESTful APIs becomes crucial. RESTful APIs provide a standardised approach to building web services that are scalable, flexible, and easy to consume. Understanding the principles and concepts behind RESTful API design is essential for developing robust and maintainable microservices.
Let's explore the key aspects of RESTful API design in the context of a microservice-based architecture:
- Resource Identification: RESTful APIs are built around resources, which can be any information or entity that is meaningful within the application domain. Identifying and defining resources is a fundamental step in API design. Resources are typically represented by unique URIs (Uniform Resource Identifiers) and can be accessed and manipulated using HTTP methods.
- Statelessness: RESTful APIs are stateless, meaning that each request from a client to a server contains all the necessary information to understand and process that request. The server does not maintain any client state between requests. This design principle simplifies scalability and improves the reliability and performance of the system.
- Uniform Interface: RESTful APIs adhere to a uniform interface that defines a set of standard HTTP methods for manipulating resources. These methods include GET (retrieve resource), POST (create resource), PUT (update resource), PATCH (partially update resource), and DELETE (remove resource). A uniform interface allows clients to interact with different services using consistent and predictable operations.
- Hypermedia as the Engine of Application State (HATEOAS): HATEOAS is a principle that encourages the inclusion of hypermedia links in API responses. Hypermedia links provide clients with information about the available actions they can perform next. By embedding links within responses, APIs enable clients to navigate and discover related resources dynamically. HATEOAS enhances the decoupling between clients and services, enabling more flexibility and extensibility.
- Communication and Integration between Microservices: RESTful APIs play a vital role in facilitating communication and integration between microservices. Each microservice can expose its functionality through well-defined API endpoints, allowing other services to consume and interact with its resources. RESTful APIs provide a standardised and interoperable way for microservices to collaborate and exchange data, enabling loose coupling and independent evolution of services.
By understanding these principles and concepts of RESTful API design, developers can create microservices that are scalable, maintainable, and interoperable. Adopting RESTful API design principles allows for the decoupling of services, promotes reusability, and facilitates seamless integration within a microservice-based architecture. It empowers developers to build loosely coupled, independently deployable services that can be combined to deliver complex business functionalities.
Examples of RESTful APIs
Netflix follows a microservice-based architecture, where each microservice represents a specific business capability. RESTful APIs are used to expose and communicate with these microservices. For example, the Netflix API allows users to browse and search for movies, manage their account settings, and stream content. The API follows RESTful principles, providing a uniform interface for client applications to interact with different microservices.
Uber's architecture is built upon microservices, and RESTful APIs play a significant role in communication between various services. The Uber API enables interactions such as requesting rides, tracking drivers, calculating fares, and managing user accounts. Each of these functionalities is exposed through RESTful endpoints, allowing mobile applications and other integrations to consume the services provided by different microservices.
GitHub, a popular code hosting and collaboration platform, follows a microservice architecture. RESTful APIs are a central component of GitHub's architecture, enabling developers to interact with repositories, manage issues and pull requests, perform code reviews, and access user and organisation data. The APIs allow integrations with various development tools and services, fostering a vibrant ecosystem of third-party applications.
These real-life examples demonstrate the effective use of RESTful API design in microservice-based architectures. By leveraging REST principles, these companies have built scalable, modular, and interoperable systems. RESTful APIs provide a standardised and intuitive way for clients to interact with microservices, enabling seamless integration, decoupling of services, and independent evolution of functionalities.
Now you have an understanding of RESTful API’s let's look at the hosting environment for web applications, Amazon EC2.
Amazon EC2
Amazon EC2 (Elastic Compute Cloud) is a web service provided by Amazon Web Services (AWS) that allows users to rent virtual servers in the cloud. It provides resizable computing capacity in the form of virtual machines known as instances. Amazon EC2 enables developers and businesses to quickly deploy applications and scale their compute resources based on demand.
Developers can deploy their applications on EC2 instances and configure them to handle incoming HTTP requests. This means that EC2 instances can serve as the infrastructure where RESTful APIs are hosted and accessed by clients.
Key features of Amazon EC2 include:
- Elasticity: Users can easily scale their compute resources up or down based on their requirements. This allows for efficient resource utilisation and cost optimisation.
- Variety of Instance Types: Amazon EC2 offers a wide range of instance types to cater to different workload requirements, such as general-purpose, compute-optimized, memory-optimized, and storage-optimized instances. Each instance type is designed to deliver specific performance characteristics.
- Flexible Pricing Options: Users can choose from various pricing models, including On-Demand instances for pay-as-you-go pricing, Reserved instances for long-term commitments with lower rates, and Spot instances for accessing spare compute capacity at discounted prices.
- Security: Amazon EC2 provides several security features, including security groups, network access control lists (ACLs), and Virtual Private Cloud (VPC) integration. Users can control inbound and outbound traffic to their instances and configure network settings as per their security requirements.
- Integration with Other AWS Services: Amazon EC2 seamlessly integrates with other AWS services, such as Amazon S3 for storage, Amazon RDS for databases, and Amazon VPC for networking. This allows users to build comprehensive and scalable cloud-based solutions.
- Availability and Fault Tolerance: Amazon EC2 offers high availability by providing multiple Availability Zones within each AWS region. Users can distribute their instances across different Availability Zones to ensure fault tolerance and minimise downtime.
- Management and Monitoring: Amazon EC2 provides management capabilities through the AWS Management Console, command-line interface (CLI), and software development kits (SDKs). It also integrates with monitoring services like Amazon CloudWatch for performance monitoring and operational insights.
Amazon EC2 is widely used by organisations of all sizes to run a variety of workloads, including web applications, data processing, batch processing, high-performance computing, and more. It offers a flexible and scalable infrastructure that allows users to quickly provision and manage compute resources in the cloud without the need for upfront hardware investment.
Links
Follow these links to find out more about Amazon EC2:
The Django framework is a high-level Python web framework that follows the Model-View-Controller (MVC) architectural pattern. It provides a set of tools and libraries that simplify the development of web applications by promoting code reusability, modularity, and rapid development. Django is widely used for building robust and scalable web applications, including RESTful APIs in the context of microservice design. Here's why Django is commonly used for RESTful APIs in microservice architectures:
- Rapid Development: Django offers a streamlined development process with built-in features and conventions. It provides a rich set of pre-built components, such as authentication, URL routing, form handling, and database management. These components accelerate the development process, allowing developers to focus on implementing business logic rather than boilerplate code.
- Object-Relational Mapping (ORM): Django includes a powerful ORM that abstracts database access and allows developers to interact with the database using Python objects. This abstraction simplifies data manipulation and makes it easier to work with multiple databases or switch between different database engines.
- Batteries Included: Django follows the "batteries included" philosophy, which means it provides a comprehensive set of features and functionalities out of the box. This includes a built-in admin interface, automatic form handling, template engine, caching, and security features. These pre-built components save development time and effort, making Django a productive framework for building RESTful APIs.
- Scalability: Django is designed to handle high-traffic and high-volume applications. It supports horizontal scalability by allowing the deployment of multiple instances behind load balancers. Additionally, Django integrates well with caching systems and asynchronous task queues, further enhancing the scalability of the system.
- Security: Django incorporates security best practices by default. It includes features like protection against common web vulnerabilities (e.g., cross-site scripting, cross-site request forgery), user authentication and authorisation, and secure password management. Django's security features help developers build secure RESTful APIs that protect sensitive data.
- Community and Ecosystem: Django has a large and active community of developers, which means there are extensive resources, documentation, and community-driven packages available. This vibrant ecosystem provides solutions for various needs, such as authentication, serialisation, testing, and API documentation generation. Community support makes it easier for developers to find solutions to common challenges and stay up-to-date with best practices.
By leveraging the Django framework, developers can build RESTful APIs in a microservice architecture efficiently. Django's ease of use, rapid development capabilities, built-in components, scalability, security features, and thriving community make it a popular choice for developing web applications and RESTful APIs. It enables developers to focus on the core functionality of the APIs while providing a solid foundation for building robust and maintainable microservices.
Live Session Tutorial: RESTful API
Your tutor will facilitate an online class to demonstrate this process in a live setting. Please check 'Live Sessions' in your navigation bar to register and attend this session.
Links
Follow these links to find out more about how to use the Django framework:
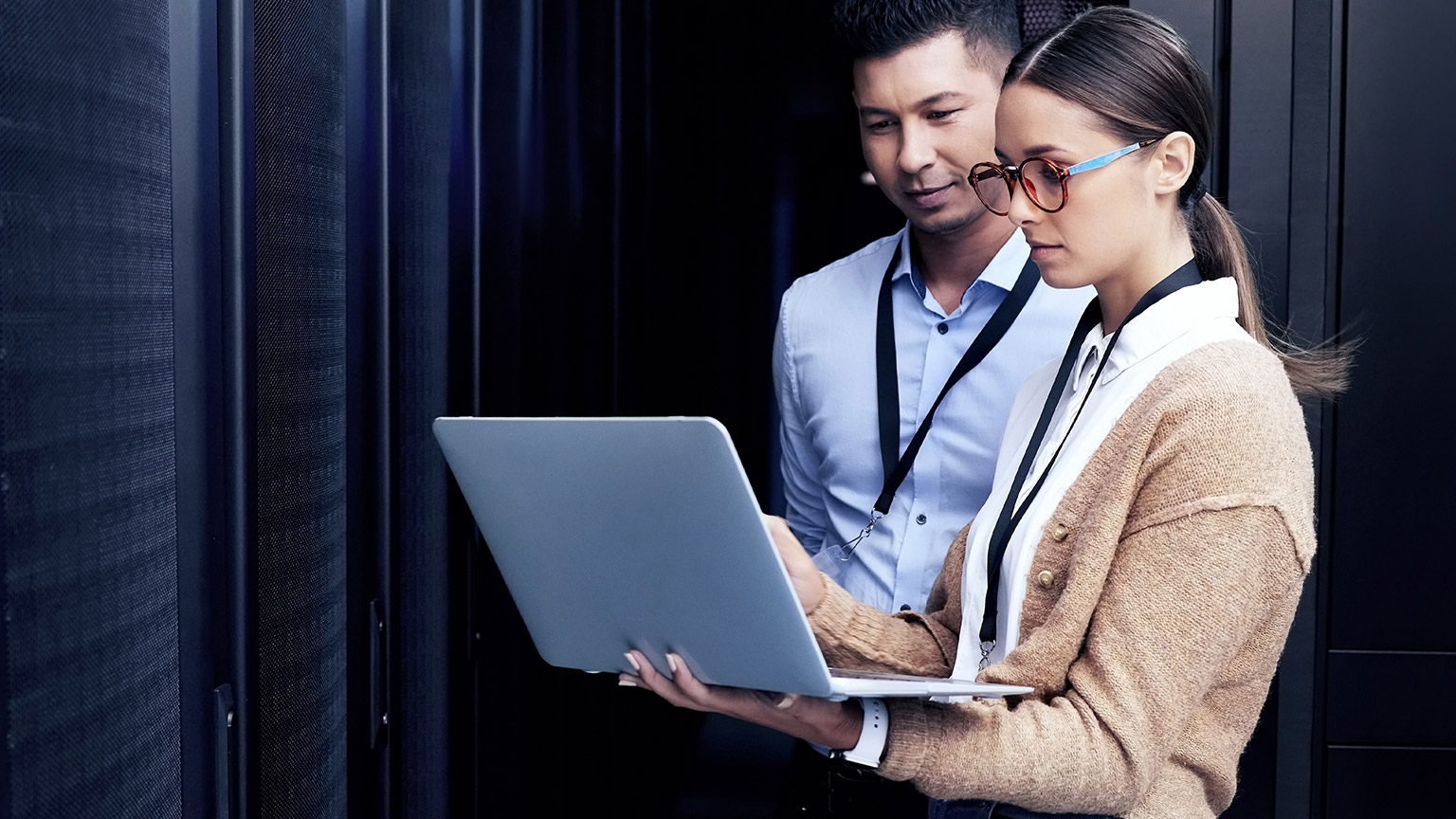